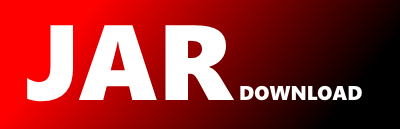
org.qas.api.ApiWebServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api;
import org.qas.api.handler.RequestHandler;
import org.qas.api.http.ExecutionContext;
import org.qas.api.http.HttpAuthClient;
import org.qas.api.http.basic.HttpUrlConnectionAuthClient;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
/**
* ApiWebServiceClient
*
* @author: Dzung Nguyen
* @version: $Id ApiWebServiceClient 2014-03-26 15:30:30z dungvnguyen $
* @since 1.0
*/
public abstract class ApiWebServiceClient> {
//~ class properties ========================================================
/**
* the service endpoint to which this client will send request.
*/
protected URI endpoint;
/**
* The client configuration.
*/
protected ClientConfiguration configuration;
/**
* Low level client for sending request to services.
*/
protected HttpAuthClient client;
/**
* Optional request handlers for additional request processing.
*/
protected final List requestHandlers;
/**
* Optional offset (in seconds) to use when signing request.
*/
protected int timeOffset;
//~ class members ===========================================================
/**
* Constructs a ApiWebServiceClient object using the specified configuration.
*
* @param configuration the client configuration for this client.
*/
protected ApiWebServiceClient(ClientConfiguration configuration) {
this.configuration = configuration;
this.client = createHttpAuthClient(configuration);
requestHandlers = Collections.synchronizedList(new LinkedList());
}
/**
* Overrides the default endpoint for this client.
*
* @param endpoint The endpoint or a full URL, including the protocol of
* specified endpoint this client will communicate with.
* @throws IllegalArgumentException If any problems are detected with the
* specified endpoint.
*/
public void setEndpoint(String endpoint) throws IllegalArgumentException {
if (endpoint.contains("://") == false) {
endpoint = configuration.getProtocol().toString() + "://" + endpoint;
}
try {
this.endpoint = new URI(endpoint);
} catch (URISyntaxException ex) {
throw new IllegalArgumentException(ex);
}
}
/**
* Sets the client configuration.
*
* @param configuration the client configuration to set.
*/
public void setConfiguration(ClientConfiguration configuration) {
this.configuration = configuration;
client = createHttpAuthClient(configuration);
}
/**
* Sets the client configuration and returns the updated client object.
*
* @param configuration the client configuration to set.
* @return the updated client object with new client configuration object.
*/
public ApiWebServiceClient withConfiguration(ClientConfiguration configuration) {
setConfiguration(configuration);
return this;
}
/**
* Shutdown this client object, release any resources that might be held
* open.
*/
public void shutdown() {
client.shutdown();
}
/**
* Appends a request handler to the list of registered handlers that are
* run as part of a request's lifecycle.
*
* @param requestHandler The new handler to add to the current list of
* request handlers.
*/
public void addRequestHandler(RequestHandler requestHandler) {
requestHandlers.add(requestHandler);
}
/**
* Appends a request handler to the list of registered handlers that are
* run as part of a request's lifecycle and return the updated client
* object.
*
* @param requestHandler The new handler to add to the current list of
* request handlers.
* @return the updated client object.
*/
public ApiWebServiceClient withRequestHandler(RequestHandler requestHandler) {
addRequestHandler(requestHandler);
return this;
}
/**
* Removes the request handler from the list of registered handlers and
* returns the updated client object.
*
* @param requestHandler the handler to remove from the current list of
* request handlers.
* @return the updated client object.
*/
public ApiWebServiceClient removeRequestHandler(RequestHandler requestHandler) {
requestHandlers.remove(requestHandler);
return this;
}
/**
* Sets the optional value for time offset for this client. This value
* will be applied to all requests processed through this client.
*
* @param timeOffset The optional value for time offset (in seconds)
* for this client.
*/
public void setTimeOffset(int timeOffset) {
this.timeOffset = timeOffset;
}
/**
* Sets the optional value for time offset for this client. This value
* will be applied to all request processed through this client and
* returns the updated client object.
*
* @param timeOffset The optional value of time offset (in seconds)
* for this client.
* @return the updated client object.
*/
public ApiWebServiceClient withTimeOffset(int timeOffset) {
setTimeOffset(timeOffset);
return this;
}
/**
* @return the optional value of time offset for this client.
*/
public int getTimeOffset() {
return timeOffset;
}
/**
* @return the execution context.
*/
protected ExecutionContext createExecutionContext() {
return new ExecutionContext(requestHandlers);
}
/**
* @param configuration configuration
* @return the {@link HttpAuthClient} from the given {@link ClientConfiguration configuration}.
*/
protected HttpAuthClient createHttpAuthClient(ClientConfiguration configuration) {
return new HttpUrlConnectionAuthClient(configuration);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy