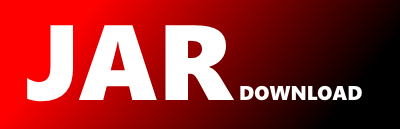
org.qas.api.AuthServiceException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api;
import java.io.IOException;
/**
* AuthServiceException
*
* @author: Dzung Nguyen
* @version: $Id AuthServiceException 2014-03-25 10:44:30z dungvnguyen $
* @since 1.0
*/
public class AuthServiceException extends AuthClientException {
//~ class properties ========================================================
private static final long serialVersionUID = -417899261734955807L;
/** The error code represented by this exception. */
private String errorCode;
/** Indicates whether this exception was the fault of the caller or the service. */
private ErrorType errorType = ErrorType.Unknown;
/** The HTTP status code that was returned with this error. */
private int statusCode;
/** The name of service that sent this error response. */
private String serviceName;
/** the request identifier. */
private String requestId;
//~ class members ===========================================================
/**
* Constructs a new {@link AuthServiceException} with the specified message.
*
* @param message An error message describing what went wrong.
*/
public AuthServiceException(String message) {
super(message);
}
/**
* Constructs a new {@link AuthServiceException} with the specified message
* and exception indicating the root cause.
*
* @param message An error message describing what went wrong.
* @param cause The root exception that caused this exception to be thrown.
*/
public AuthServiceException(String message, Throwable cause) {
super(message, cause);
}
/**
* @return the name of service that sent this error response.
*/
public String getServiceName() {
return this.serviceName;
}
/**
* Sets the name of service that sent this error response.
*
* @param serviceName the name of service that sent this error response.
*/
public void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
/**
* Sets the name of service that sent this error response and returns
* the updated {@link AuthServiceException} object.
*
* @param serviceName the name of service that sent this error response.
* @return the updated {@link AuthServiceException} object with the new
* service name.
*/
public AuthServiceException withServiceName(String serviceName) {
setServiceName(serviceName);
return this;
}
/**
* @return the error code represented by this exception.
*/
public String getErrorCode() {
return this.errorCode;
}
/**
* Sets the error code represented by this exception.
*
* @param errorCode the error code represented by this exception.
*/
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
/**
* Sets the error code represented by this exception and returns
* the updated {@link AuthServiceException} object.
*
* @param errorCode the error code represented by this exception.
* @return the updated {@link AuthServiceException} object with the new
* error code.
*/
public AuthServiceException withErrorCode(String errorCode) {
setErrorCode(errorCode);
return this;
}
/**
* @return The HTTP status code that was returned with this service exception.
*/
public int getStatusCode() {
return statusCode;
}
/**
* Sets the HTTP status code that was returned with this service exception.
*
* @param statusCode the HTTP status code that was returned with this service
* exception.
*/
public void setStatusCode(int statusCode) {
this.statusCode = statusCode;
}
/**
* Sets the HTTP status code that was returned with this service exception
* and returns the updated {@link AuthServiceException} object.
*
* @param statusCode the HTTP status code that was returned with this service
* exception.
* @return the updated {@link AuthServiceException} object with the new
* status code.
*/
public AuthServiceException withStatusCode(int statusCode) {
setStatusCode(statusCode);
return this;
}
/**
* @return A value indicating who is responsible for this exception.
*/
public ErrorType getErrorType() {
return this.errorType;
}
/**
* Sets the type of error represented by this exception, indicating if this
* exception was the caller's fault, or the service's fault.
*
* @param errorType The type of error represented by this exception,
* indicating if this exception was the caller's fault
* or the service's fault.
*/
public void setErrorType(ErrorType errorType) {
this.errorType = errorType;
}
/**
* Sets the type of error represented by this exception, indicating if this
* exception was the caller's fault, or the service's fault and returns the
* updated {@link AuthServiceException} object.
*
* @param errorType The type of error represented by this exception,
* indicating if this exception was the caller's fault
* or the service's fault.
* @return the updated {@link AuthServiceException} object with the new
* error type.
*/
public AuthServiceException withErrorType(ErrorType errorType) {
this.errorType = errorType;
return this;
}
/**
* @return the request identifier that uniquely identifies the service request the caller made.
*/
public String getRequestId() {
return this.requestId;
}
/**
* Sets the request identifier for this exception.
*
* @param requestId The unique identifier for the service request the caller made.
*/
public void setRequestId(String requestId) {
this.requestId = requestId;
}
/**
* Sets the request identifier for this exception.
*
* @param requestId The unique identifier for the service request the caller made.
*
* @return the updated {@link AuthServiceException} object with the new request identifier.
*/
public AuthServiceException withRequestId(String requestId) {
setRequestId(requestId);
return this;
}
@Override
protected void appendExceptionTo(Appendable out, Throwable e) throws IOException {
out.append(">>> Type: ").append(e.getClass().getName()).append(NL);
out.append(">>> Message: ").append(e.getMessage()).append(NL);
out.append(">>> Request Id: ").append(getRequestId()).append(NL);
out.append(">>> Status Code: ").append(String.valueOf(getStatusCode())).append(NL);
out.append(">>> Error Code: ").append(getErrorCode()).append(NL);
out.append(">>> Error Type: ").append(getErrorType().name()).append(NL);
}
/**
* Indicates who is responsible for a failed request.
*/
public enum ErrorType {
Client,
Service,
Unknown
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy