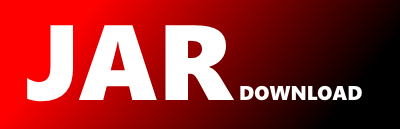
org.qas.api.JsonMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.util.Collections;
import java.util.Map;
import java.util.logging.Logger;
/**
* @author trongle
* @version $Id 4/21/2017 3:42 PM
*/
public class JsonMapper {
private static final Logger LOG = Logger.getLogger(JsonMapper.class.getName());
/**
* Use for JSON
*/
private static final ObjectMapper mapper = new ObjectMapper();
static {
// mapper
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
}
private JsonMapper() {
}
/**
* Create new ObjectNode
*
* @return
*/
public static ObjectNode newNode() {
return mapper.createObjectNode();
}
/**
* Get instance of valueType from JSON data
*
* @param body JSON string
* @param valueType class type to cast
*
* @return instance of class valueType
*/
public static T fromJson(String body, Class valueType) throws IOException {
return parseJson(body, valueType);
}
/**
* @param body
* @param valueType
* @param
*
* @return
*
* @throws IOException
*/
public static T parseJson(String body, Class valueType) throws IOException {
if (isEmpty(body))
return null;
return mapper.readValue(body, valueType);
}
public static T parseJson(String body, TypeReference type) throws IOException {
if (isEmpty(body))
return null;
return mapper.readValue(body, type);
}
public static T fromJson(String body, TypeReference type) {
try {
if (isEmpty(body))
return null;
return mapper.readValue(body, type);
} catch (IOException e) {
LOG.info("ERROR: cannot parse json" + e.getMessage());
return null;
}
}
/**
* Get JsonNode from object append field extraData to JsonNode
*
* @return JsonNode, if data is a instance of POJO. Otherwise return empty string
*/
public static ObjectNode toJsonNode(Object data) {
if (null == data)
return newNode();
ObjectNode node = null;
try {
// convert data to ObjectNode
node = mapper.valueToTree(data);
} catch (IllegalArgumentException e) {
return newNode();
}
return node;
}
/**
* serial object to JSON string
*
* @param data
*
* @return
*/
public static String toJson(Object data) {
if (null == data)
return "";
try {
return mapper.writeValueAsString(data);
} catch (JsonProcessingException e) {
return "";
}
}
public static Map toMap(Object data) {
if (null == data) {
return Collections.emptyMap();
}
try {
return mapper.convertValue(data, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy