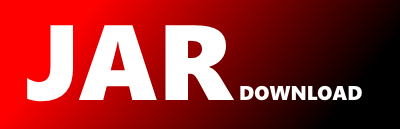
org.qas.api.SimpleCredentials Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api;
import com.fasterxml.jackson.databind.node.ObjectNode;
import org.qas.api.internal.PropertyContainer;
/**
* SimpleCredentials
*
* @author: Dzung Nguyen
* @version: $Id SimpleCredentials 2014-03-26 15:22:30z dungvnguyen $
* @since 1.0
*/
public class SimpleCredentials extends PropertyContainer implements Credentials, Cloneable {
//~ class properties ========================================================
private static final String USERNAME_RESERVED_PROPERTY = "__username__";
private static final String PASSWORD_RESERVED_PROPERTY = "__password__";
/**
* Creates SimpleCredentials object.
*/
private SimpleCredentials() {
}
/**
* Creates SimpleCredentials object from the given username and password.
*
* @param username the given username.
* @param password the given password.
*/
public SimpleCredentials(String username, char[] password) {
this(username, new String(password));
}
/**
* Creates SimpleCredentials object from the given username and password.
*
* @param username the given username.
* @param password the given password.
*/
public SimpleCredentials(String username, String password) {
// set username and password.
setProperty(USERNAME_RESERVED_PROPERTY, username);
setProperty(PASSWORD_RESERVED_PROPERTY, password);
}
/**
* Returns the value of the named attributes as an Object, or {@code null} if
* no attribute of the given name exists.
*
* @param name a String specifying the name of the attribute.
* @return an Object containing the value attribute, or {@code null} if the
* attribute does not exists.
*/
public Object getAttribute(String name) {
return getProperty(name);
}
/**
* Stores an attribute in this credentials instance.
*
* @param name the String specifying the name of attribute.
* @param value the Object to be stored.
*/
public void setAttribute(String name, Object value) {
setProperty(name, value);
}
/**
* Stores an attribute in this credentials instance and return the updated
* {@link SimpleCredentials} object.
*
* @param name the String specifying the name of attribute.
* @param value the Object to be store.
* @return the updated {@link SimpleCredentials} object with the new
* new attribute to store.
*/
public SimpleCredentials withAttribute(String name, Object value) {
setAttribute(name, value);
return this;
}
/**
* @return the credentials username.
*/
public String getUsername() {
return getProperty(USERNAME_RESERVED_PROPERTY).toString();
}
/**
* @return the credentials password.
*/
public String getPassword() {
return getProperty(PASSWORD_RESERVED_PROPERTY).toString();
}
/**
* @return the deep copy of the current {@link SimpleCredentials} object.
*/
@Override
public SimpleCredentials clone() {
SimpleCredentials credentials = new SimpleCredentials();
credentials.setPropertiesFrom(this);
return credentials;
}
@Override
public String toString() {
ObjectNode node = JsonMapper.toJsonNode(this);
if (null == node) {
return "{}";
}
node.remove(PASSWORD_RESERVED_PROPERTY);
return node.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy