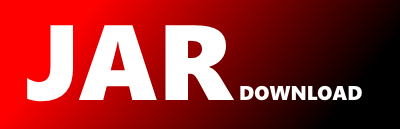
org.qas.api.http.ExecutionContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.http;
import org.qas.api.Credentials;
import org.qas.api.auth.Signer;
import org.qas.api.handler.RequestHandler;
import org.qas.api.internal.CustomBackoffStrategy;
import java.util.LinkedList;
import java.util.List;
/**
* ExecutionContext
*
* @author: Dzung Nguyen
* @version: $Id ExecutionContext 2014-03-26 15:35:30z dungvnguyen $
* @since 1.0
*/
public final class ExecutionContext {
//~ class properties ========================================================
private final List requestHandlers;
private String contextUserAgent;
private Signer signer;
private Credentials credentials;
private CustomBackoffStrategy customBackoffStrategy;
//~ class members ===========================================================
/**
* Creates {@link ExecutionContext} object from the empty list of
* {@link RequestHandler} objects.
*/
public ExecutionContext() {
this(new LinkedList());
}
/**
* Creates {@link ExecutionContext} object from the list of {@link RequestHandler}
* objects.
*
* @param requestHandlers the list of {@link RequestHandler} objects to handle the
* request and response.
*/
public ExecutionContext(List requestHandlers) {
this.requestHandlers = requestHandlers;
}
/**
* @return the context user agent.
*/
public String getContextUserAgent() {
return contextUserAgent;
}
/**
* Sets the context user agent.
*
* @param contextUserAgent the context user agent to set.
*/
public void setContextUserAgent(String contextUserAgent) {
this.contextUserAgent = contextUserAgent;
}
/**
* Sets the context user agent and returns the updated {@link ExecutionContext}
* object.
*
* @param contextUserAgent the context user agent.
* @return the updated {@link ExecutionContext} object with a new context user
* agent setting.
*/
public ExecutionContext withContextUserAgent(String contextUserAgent) {
setContextUserAgent(contextUserAgent);
return this;
}
/**
* @return the list of {@link RequestHandler} object.
*/
public List getRequestHandlers() {
return requestHandlers;
}
/**
* @return the {@link Signer} object used to sign the request.
*/
public Signer getSigner() {
return this.signer;
}
/**
* Sets the {@link Signer} object.
*
* @param signer the given {@link Signer} object to set.
*/
public void setSigner(Signer signer) {
this.signer = signer;
}
/**
* Sets the {@link Signer} object and returns the updated {@link ExecutionContext}
* object.
*
* @param signer the {@link Signer} object to set.
* @return the updated {@link ExecutionContext} object with a new {@link Signer}
* object.
*/
public ExecutionContext withSigner(Signer signer) {
setSigner(signer);
return this;
}
/**
* @return the execute context credentials.
*/
public Credentials getCredentials() {
return this.credentials;
}
/** http://analytics.bkav.com/
* Sets the execute context credentials.
*
* @param credentials the credentials to set.
*/
public void setCredentials(Credentials credentials) {
this.credentials = credentials;
}
/**
* Sets the execute context credentials and return the updated
* {@link ExecutionContext} object.
*
* @param credentials the execution context credentials
* @return the updated {@link ExecutionContext} object with a new
* execution context credentials.
*/
public ExecutionContext withCredentials(Credentials credentials) {
setCredentials(credentials);
return this;
}
/**
* Returns the optional custom backoff strategy for controlling how long
* between retries on error responses. If no custom backoff strategy is
* specified, a default exponential backoff strategy is used.
*
* @return the optional custom backoff strategy for the associated request.
*/
public CustomBackoffStrategy getCustomBackoffStrategy() {
return customBackoffStrategy;
}
/**
* Sets the optional custom backoff strategy for controlling how long
* between retries on error responses. If no custom backoff strategy is
* specified, a default exponential backoff strategy is used.
*
* @param backoffStrategy
* The optional custom backoff strategy for the associated
* request.
*/
public void setCustomBackoffStrategy(CustomBackoffStrategy backoffStrategy) {
this.customBackoffStrategy = backoffStrategy;
}
/**
* Sets the optional custom backoff strategy for controlling how long
* between retries on error responses. If no custom backoff strategy is
* specified, a default exponential backoff strategy is used.
*
* @param backoffStrategy
* The optional custom backoff strategy for the associated
* request.
* @return the updated execution context.
*/
public ExecutionContext withCustomBackoffStrategy(CustomBackoffStrategy backoffStrategy) {
setCustomBackoffStrategy(backoffStrategy);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy