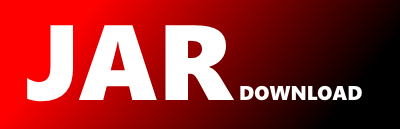
org.qas.api.http.HttpRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.http;
import org.qas.api.ApiServiceRequest;
import java.io.InputStream;
import java.net.URI;
import java.util.Map;
/**
* HttpRequest
*
* @author: Dzung Nguyen
* @version: $Id HttpRequest 2014-03-26 15:43:30z dungvnguyen $
* @since 1.0
*/
public interface HttpRequest {
/**
* @return HTTP request content type.
*/
String getContentType();
/**
* @return HTTP request content length.
*/
int getContentLength();
/**
* @return HTTP request underlying object.
*/
T getUnderlying();
/**
* Adds the specified header to this request.
*
* @param name the name of the header to add.
* @param value the header's value.
*/
void setHeader(String name, String value);
/**
* Adds the specified header to this request and returns the updated
* HttpRequest object.
*
* @param name the name of the header to add.
* @param value the header's value.
*
* @return the updated {@link HttpRequest} object with a new header.
*/
HttpRequest withHeader(String name, String value);
/**
* @return A map of all headers include in this request.
*/
Map getHeaders();
/**
* Sets all headers, clearing any existing ones.
*
* @param headers the given map of headers to set.
*/
void setHeaders(Map headers);
/**
* Sets all headers, clearing any existing ones and returns the updated
* {@link HttpRequest} object.
*
* @param headers the given map of headers to set.
* @return the updated {@link HttpRequest} object with a new headers.
*/
HttpRequest withHeaders(Map headers);
/**
* @return the path to the resource being requested.
*/
String getResourcePath();
/**
* Sets the path to the resource being requested.
*
* @param resourcePath the path to the resource being requested.
*/
void setResourcePath(String resourcePath);
/**
* Sets the path to the resource being requested and returns the updated
* {@link HttpRequest} object.
*
* @param resourcePath the path to the resource being requested.
* @return the updated {@link HttpRequest} object with a new resource path.
*/
HttpRequest withResourcePath(String resourcePath);
/**
* Adds the specified request parameter to this request.
*
* @param name the name of the request parameter.
* @param value the value of the request parameter.
*/
void addParameter(String name, String value);
/**
* Adds the specified request parameter to this request and returns the
* updated {@link HttpRequest} object.
*
* @param name the name of the request parameter.
* @param value the value of the request parameter.
* @return the updated {@link HttpRequest} object with a new parameter.
*/
HttpRequest withParameter(String name, String value);
/**
* @return A map of all parameters in this request.
*/
Map getParameters();
/**
* Sets all parameters, clearing any existing values.
*
* @param parameters the map of parameters to set.
*/
void setParameters(Map parameters);
/**
* Sets all parameters, clearing any existing values and return the updated
* {@link HttpRequest} object.
*
* @param parameters the map of parameters to set.
* @return the updated {@link HttpRequest} object with new map of parameters.
*/
HttpRequest withParameters(Map parameters);
/**
* @return the service endpoint to which this request should be sent.
*/
URI getEndpoint();
/**
* @return the HTTP method to use when sending this request.
*/
HttpMethod getHttpMethod();
/**
* Sets the HTTP method to use when sending this request.
*
* @param httpMethod the HTTP method to use when sending this request.
*/
void setHttpMethod(HttpMethod httpMethod);
/**
* Sets the HTTP method to use when sending this request and returns the
* updated {@link HttpRequest} object.
*
* @param httpMethod the HTTP method to use when sending this request.
* @return the updated {@link HttpRequest} object with a new http method.
*/
HttpRequest withHttpMethod(HttpMethod httpMethod);
/**
* @return The optional stream containing the payload data to include for
* this request.
*/
InputStream getContent();
/**
* Sets the optional stream containing the payload data to include for this
* request. Not all requests will contain payload data.
*
* @param content the optional stream containing the payload data to include
* for request.
*/
void setContent(InputStream content);
/**
* Sets the optional stream containing the payload data to include for this
* request. Not all requests will contain payload data and returns the
* updated {@link HttpRequest} object.
*
* @param content the optional stream containing the payload data to include
* for request.
* @return the updated {@link HttpRequest} object with a new content.
*/
HttpRequest withContent(InputStream content);
/**
* @return the name of the service this request is for.
*/
String getServiceName();
/**
* @return optional value for time offset for this request.
*/
int getTimeOffset();
/**
* Sets the optional value for time offset for this request. This will be
* used by the signer to adjust for potential clock skew. Value is in
* seconds, positive values imply the current clock is "fast", negative
* values imply clock is slow.
*
* @param timeOffset The optional value for time offset for this request.
*/
void setTimeOffset(int timeOffset);
/**
* Sets the optional value for time offset for this request. This will be
* used by the signer to adjust for potential clock skew. Value is in
* seconds, positive values imply the current clock is "fast", negative
* values imply clock is slow and returns the updated {@link HttpRequest} object.
*
* @param timeOffset The optional value for time offset for this request.
* @return the updated {@link HttpRequest} object with a new time offset.
*/
HttpRequest withTimeOffset(int timeOffset);
/**
* Returns the original, user facing request object which this basic
* request object is representing.
*
* @return the original, user facing request object which this basic
* request object is representing.
*/
ApiServiceRequest getOriginalRequest();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy