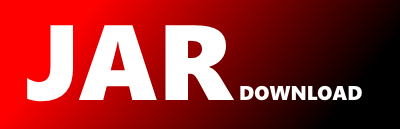
org.qas.api.http.JsonErrorResponseHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.http;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.api.JsonMapper;
import org.qas.api.internal.util.json.JsonObject;
import org.qas.api.transform.Unmarshaller;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.List;
import java.util.Map;
/**
* JsonErrorResponseHandler
*
* @author Dzung Nguyen
* @version $Id JsonErrorResponseHandler 2014-03-27 11:37:30z dungvnguyen $
* @since 1.0
*/
public class JsonErrorResponseHandler implements HttpResponseHandler {
//~ class properties ========================================================
/**
* The list of error response unmarshallers to try to apply to error
* responses.
*/
private List> unmarshallerList;
//~ class members ===========================================================
public JsonErrorResponseHandler(List> exceptionUnmarshallers) {
this.unmarshallerList = exceptionUnmarshallers;
}
public AuthServiceException handle(HttpResponse response) throws Exception {
String streamContents = JsonMapper.toString(response.getContent());
JsonObject jsonErrorMessage;
try {
String s = (streamContents != null ? streamContents.trim() : "");
if (s.length() == 0) {
s = "{}";
} else if (!s.startsWith("{") || !s.endsWith("}")) {
s = new JsonObject().accumulate("message", s).toString();
}
jsonErrorMessage = new JsonObject(s);
} catch (Exception e) {
throw new AuthClientException("Unable to parse error response: '" + streamContents + "'", e);
}
AuthServiceException ase = runErrorUnmarshallers(response, jsonErrorMessage);
if (ase == null) return null;
ase.setServiceName(response.getRequest().getServiceName());
ase.setStatusCode(response.getStatusCode());
if (response.getStatusCode() < 500) {
ase.setErrorType(AuthServiceException.ErrorType.Client);
} else {
ase.setErrorType(AuthServiceException.ErrorType.Service);
}
for (Map.Entry headerEntry : ((Map) response.getHeaders()).entrySet()) {
if (headerEntry.getKey().equalsIgnoreCase("x-qtest-request-id")) {
ase.setRequestId(headerEntry.getValue());
}
}
return ase;
}
protected AuthServiceException runErrorUnmarshallers(HttpResponse errorResponse, JsonObject json) throws Exception {
/*
* We need to select which exception unmarshaller is the correct one to
* use from all the possible exceptions this operation can throw.
* Currently we rely on the unmarshallers to return null if they can't
* unmarshall the response, but we might need something a little more
* sophisticated in the future.
*/
for (Unmarshaller unmarshaller : unmarshallerList) {
AuthServiceException ase = unmarshaller.unmarshall(json);
if (ase != null) {
ase.setStatusCode(errorResponse.getStatusCode());
return ase;
}
}
return null;
}
public boolean needsConnectionLeftOpen() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy