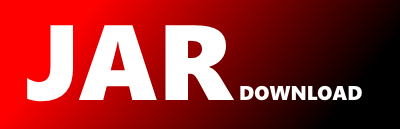
org.qas.api.http.JsonResponseHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.http;
import org.qas.api.ApiServiceResponse;
import org.qas.api.JsonMapper;
import org.qas.api.ResponseMetadata;
import org.qas.api.transform.JsonUnmarshallerContext;
import org.qas.api.transform.Unmarshaller;
import org.qas.api.transform.VoidJsonUnmarshaller;
import java.net.HttpURLConnection;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* JsonResponseHandler
*
* @author Dzung Nguyen
* @version $Id JsonResponseHandler 2014-03-27 11:25:30z dungvnguyen $
* @since 1.0
*/
public class JsonResponseHandler implements HttpResponseHandler> {
//~ class properties ========================================================
/**
* The JSON unmarshaller to use when handling the response
*/
private Unmarshaller responseUnmarshaller;
/**
* Shared logger for profiling information
*/
private static final Logger LOG = Logger.getLogger(JsonResponseHandler.class.getName());
public boolean needsConnectionLeftOpen = false;
//~ class members ===========================================================
/**
* Constructs a new response handler that will use the specified StAX
* unmarshaller to unmarshall the service response and uses the specified
* response element path to find the root of the business data in the
* service's response.
*
* @param responseUnmarshaller The StAX unmarshaller to use on the response.
*/
public JsonResponseHandler(Unmarshaller responseUnmarshaller) {
this.responseUnmarshaller = responseUnmarshaller;
/*
* Even if the invoked operation just returns null, we still need an
* unmarshaller to run so we can pull out response metadata.
*
* We might want to pass this in through the client class so that we
* don't have to do this check here.
*/
if (this.responseUnmarshaller == null) {
this.responseUnmarshaller = new VoidJsonUnmarshaller();
}
}
public ApiServiceResponse handle(HttpResponse response) throws Exception {
if (LOG.isLoggable(Level.ALL)) {
LOG.log(Level.ALL, "Parsing service response JSON");
}
String data = null;
if (responseUnmarshaller.isParseJson()) {
data = JsonMapper.toString(((HttpURLConnection) response.getUnderlying()).getInputStream());
}
try {
ApiServiceResponse awsResponse = new ApiServiceResponse();
T result = responseUnmarshaller.parse(data);
awsResponse.setResult(result);
Map metadata = new HashMap(response.getHeaders());
metadata.put(ResponseMetadata.REQUEST_ID, getRequestId(response));
awsResponse.setMetadata(new ResponseMetadata(metadata));
return awsResponse;
} finally {
}
}
/**
* Hook for subclasses to override in order to collect additional metadata
* from service responses.
*
* @param unmarshallerContext The unmarshaller context used to process a service's response
* data.
*/
protected void registerAdditionalMetadataExpressions(JsonUnmarshallerContext unmarshallerContext) {
}
/**
* Since this response handler completely consumes all the data from the
* underlying HTTP connection during the handle method, we don't need to
* keep the HTTP connection open.
*/
public boolean needsConnectionLeftOpen() {
return needsConnectionLeftOpen;
}
/**
* @return the response request header.
*/
protected String getRequestId(HttpResponse response) {
return ((Map) response.getHeaders()).get("x-qtest-request-id");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy