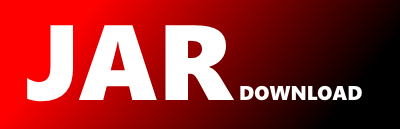
org.qas.api.http.StringResponseHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.http;
import org.qas.api.ApiServiceResponse;
import org.qas.api.ResponseMetadata;
import org.qas.api.transform.StringUnmarshaller;
import org.qas.api.transform.Unmarshaller;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* StringResponseHandler
*
* @author Dzung Nguyen
* @version $Id StringResponseHandler 2014-07-09 10:46:30z dungvnguyen $
* @since 1.0
*/
public class StringResponseHandler implements HttpResponseHandler> {
//~ class properties ========================================================
/* shared logger for profiling information. */
private static final Logger LOG = Logger.getLogger(StringResponseHandler.class.getName());
/* the response unmarshaller. */
private Unmarshaller responseUnmarshaller;
//~ class members ===========================================================
/**
* Creates {@link StringResponseHandler} to handle response.
*/
public StringResponseHandler() {
this.responseUnmarshaller = new StringUnmarshaller();
}
/**
* Creates {@link StringResponseHandler} to handle response.
*
* @param responseUnmarshaller the given response unmarshaller.
*/
public StringResponseHandler(Unmarshaller responseUnmarshaller) {
this.responseUnmarshaller = responseUnmarshaller;
/*
* Even if the invoked operation just returns null, we still need an
* unmarshaller to run so we can pull out response metadata.
*
* We might want to pass this in through the client class so that we
* don't have to do this check here.
*/
if (responseUnmarshaller == null) {
this.responseUnmarshaller = new StringUnmarshaller();
}
}
public ApiServiceResponse handle(HttpResponse> httpResponse) throws Exception {
if (LOG.isLoggable(Level.ALL)) {
LOG.log(Level.ALL, "Handle the service response.");
}
// read response content.
InputStream content = httpResponse.getContent();
try {
ApiServiceResponse awsResponse = new ApiServiceResponse();
// process content.
if (content != null) {
awsResponse.setResult(responseUnmarshaller.unmarshall(content));
} else {
awsResponse.setResult(null);
}
// read metadata.
Map metadata = new HashMap();
metadata.put(ResponseMetadata.REQUEST_ID, httpResponse.getHeaders().get("x-qtest-request-id"));
awsResponse.setMetadata(new ResponseMetadata(metadata));
// aws response.
return awsResponse;
} finally {
}
}
public boolean needsConnectionLeftOpen() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy