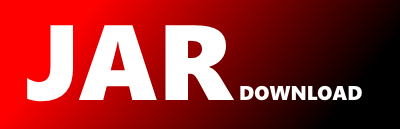
org.qas.api.http.basic.HttpUrlConnectionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.http.basic;
import org.qas.api.http.*;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* HttpUrlConnectionResponse
*
* @author Dzung Nguyen
* @version $Id HttpUrlConnectionResponse 2014-03-27 12:02:30z dungvnguyen $
* @since 1.0
*/
public final class HttpUrlConnectionResponse extends AbstractHttpResponse
implements HttpResponse {
//~ class properties ========================================================
private final HttpURLConnection connection;
private final HttpRequest request;
private final Map headers = new HashMap();
private int statusCode;
private String status;
private InputStream content;
//~ class members ===========================================================
/**
* Constructs the {@link HttpUrlConnectionResponse} associated with the specified
* connection and request.
*
* @param connection the associated connection that generated this response.
* @param request the associated request that generated this response.
*/
public HttpUrlConnectionResponse(final HttpURLConnection connection,
final HttpRequest request)
throws IOException {
this.connection = connection;
this.request = request;
// process the response.
this.statusCode = connection.getResponseCode();
this.status = connection.getResponseMessage();
Map> headerFields = connection.getHeaderFields();
if (headerFields != null && !headerFields.isEmpty()) {
for (Map.Entry> entry : headerFields.entrySet()) {
if (entry.getKey() != null && entry.getValue() != null && entry.getValue().size() > 0) {
headers.put(entry.getKey(), entry.getValue().get(0));
}
}
}
content = connection.getErrorStream();
if (content == null) {
content = connection.getInputStream();
}
}
@Override
public InputStream getContent() throws IOException {
return this.content;
}
@Override
public int getStatusCode() throws IOException {
return this.statusCode;
}
@Override
public String getStatus() throws IOException {
return this.status;
}
@Override
public HttpRequest getRequest() {
return this.request;
}
@Override
public HttpURLConnection getUnderlying() {
return this.connection;
}
@Override
public Map getHeaders() {
return Collections.unmodifiableMap(this.headers);
}
@Override
public void close() {
try {
connection.disconnect();
} catch (Exception ex) {}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy