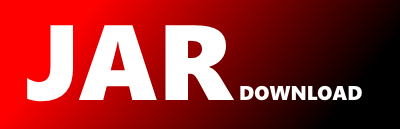
org.qas.api.internal.PropertyContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.internal;
import com.fasterxml.jackson.annotation.*;
import org.qas.api.JsonMapper;
import org.qas.api.JsonModel;
import org.qas.api.internal.util.json.JsonException;
import org.qas.api.internal.util.json.JsonObject;
import java.util.*;
import java.util.regex.Pattern;
/**
* PropertyContainer
*
* @author: Dzung Nguyen
* @version: $Id PropertyContainer 2014-01-13 17:50:30z dungvnguyen $
* @since 1.0
*/
@JsonModel
@JsonInclude(JsonInclude.Include.NON_NULL)
public class PropertyContainer implements Cloneable {
//~ class properties ========================================================
// the pattern that recognizing the reserved name.
protected static final Pattern RESERVED_NAME = Pattern.compile("^__.*__$");
protected static final String NL = System.getProperty("line.separator");
protected final Map properties = new HashMap<>();
//~ class members ===========================================================
/**
* Gets the property with the specified name. The value returned may not be
* the same type as originally set via {@link #setProperty(String, Object)}
*
* @param name the property name.
* @return the property corresponding to {@code name}
*/
@JsonGetter
public Object getProperty(String name) {
return properties.get(name);
}
/**
* Gets all of the properties belonging to this container.
*
* @return an unmodifiable {@code Map} of properties.
*/
@JsonIgnore
public Map getProperties() {
Map props = new HashMap(properties.size());
// copy the object.
for (Map.Entry entry : properties.entrySet()) {
props.put(entry.getKey(), entry.getValue());
}
return Collections.unmodifiableMap(props);
}
/**
* Returns {@code true} if a property has been set. This function can be used to
* test if property has been specifically set to {@code null}.
*
* @param name the given property name.
* @return {@code true} iff the property named {@code name} exists.
*/
@JsonIgnore
public boolean hasProperty(String name) {
return properties.containsKey(name);
}
/**
* Removes the property which the specified name. If the is no property with this
* name set, simply does nothing.
*
* @param name the given property name.
*/
public void removeProperty(String name) {
properties.remove(name);
}
/**
* Sets the property named, {@code name}, to {@code value}
*
* @param name the given property name.
* @param value the given property value.
*/
@JsonAnySetter
public void setProperty(String name, Object value) {
properties.put(name, value);
}
/**
* A convenience method that populates properties from those in the given container.
*
* @param src the container from which we will populate ourself.
*/
public void setPropertiesFrom(PropertyContainer src) {
for (Map.Entry entry : src.getPropertyMap().entrySet()) {
String name = entry.getKey();
Object entryValue = entry.getValue();
if (entryValue instanceof Collection>) {
Collection> srcCol = (Collection>) entryValue;
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy