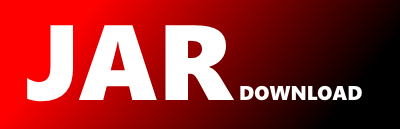
org.qas.api.internal.util.Dates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.internal.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import java.util.SimpleTimeZone;
import java.util.TimeZone;
/**
* Dates
*
* @author Dzung Nguyen
* @version $Id Dates 2014-03-27 09:27:30z dungvnguyen $
* @since 1.0
*/
public final class Dates {
//~ class properties ========================================================
protected final SimpleDateFormat urlDateFormat = new SimpleDateFormat("yyyyMMdd");
protected final SimpleDateFormat iso8601DateFormat = new SimpleDateFormat("yyyy-MM-dd");
protected final SimpleDateFormat iso8601DateTimeFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss.SSSZ");
protected final SimpleDateFormat alternateIso8601DateTimeFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ssZ");
protected final SimpleDateFormat alternateIso8601DateTimeFormatWithoutZ = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss");
protected final SimpleDateFormat rfc822DateFormat = new SimpleDateFormat("EEE, dd MMM yyyy HH:mm:ss Z", Locale.US);
//~ class members ===========================================================
private Dates() {
iso8601DateFormat.setTimeZone(new SimpleTimeZone(0, "GMT"));
iso8601DateTimeFormat.setTimeZone(new SimpleTimeZone(0, "GMT"));
alternateIso8601DateTimeFormat.setTimeZone(new SimpleTimeZone(0, "GMT"));
alternateIso8601DateTimeFormatWithoutZ.setTimeZone(new SimpleTimeZone(0, "GMT"));
rfc822DateFormat.setTimeZone(new SimpleTimeZone(0, "GMT"));
}
public static Dates getInstance() {
return new Dates();
}
public Date parseIso8601Date(String source) throws ParseException {
if (source == null || "".equals(source)) return null;
// check include timezone.
int tzPos = source.indexOf("+");
if (tzPos > 0 && (source.charAt(tzPos + 3) == ':') && (source.length() > tzPos + 4)) {
source = source.substring(0, tzPos + 3) + source.substring(tzPos + 4);
}
try {
synchronized (iso8601DateTimeFormat) {
return iso8601DateTimeFormat.parse(source);
}
} catch (ParseException ex) {
try {
synchronized (alternateIso8601DateTimeFormat) {
return alternateIso8601DateTimeFormat.parse(source);
}
} catch (ParseException pex) {
try {
synchronized (alternateIso8601DateTimeFormatWithoutZ) {
return alternateIso8601DateTimeFormatWithoutZ.parse(source);
}
} catch (ParseException ppex) {
synchronized (iso8601DateFormat) {
return iso8601DateFormat.parse(source);
}
}
}
}
}
public Date parseRfc822Date(String source) throws ParseException {
synchronized (rfc822DateFormat) {
return rfc822DateFormat.parse(source);
}
}
public String formatAlternateIso8601Date(Date date) {
synchronized (alternateIso8601DateTimeFormat) {
return alternateIso8601DateTimeFormat.format(date);
}
}
public String formatIso8601DateTime(Date date) {
synchronized (iso8601DateTimeFormat) {
return iso8601DateTimeFormat.format(date);
}
}
public String formatIso8601Date(Date date) {
synchronized (iso8601DateFormat) {
return iso8601DateFormat.format(date);
}
}
public String formaturlDate(Date date) {
synchronized (urlDateFormat) {
return urlDateFormat.format(date);
}
}
public String formatRfc822Date(Date date) {
synchronized (rfc822DateFormat) {
return rfc822DateFormat.format(date);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy