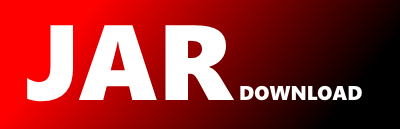
org.qas.api.internal.util.Encoders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.internal.util;
import org.qas.api.internal.util.google.base.Charsets;
import org.qas.api.internal.util.google.io.BaseEncoding;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
/**
* Encoders
*
* @author Dzung Nguyen
* @version $Id Encoders 2014-03-27 09:25:30z dungvnguyen $
* @since 1.0
*/
public final class Encoders {
//~ class properties ========================================================
private static final char[] HEX_DIGIT = {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F'};
private static final String LINE_SEPARATORS = "\r\n";
//~ class members ===========================================================
private Encoders() {
throw new AssertionError("The Encoders utilities must not be instantiated.");
}
/**
* @param source source
* @return the utf8 encode of the given {@code source} as string.
*/
public static byte[] utf8encode(String source) {
if (source == null) return null;
if (source.length() == 0) return new byte[0];
return source.getBytes(Charsets.UTF_8);
}
/**
* @param source source
* @return the utf8 decode of the given {@code source} as bytes array.
*/
public static String utf8decode(byte[] source) {
if (source == null) return null;
if (source.length == 0) return "";
return new String(source, Charsets.UTF_8);
}
/**
* @param source source
* @return the base64 encode of the given {@code source} as bytes array.
*/
public static byte[] base64Encode(byte[] source) {
return utf8encode(BaseEncoding.base64().encode(source));
}
/**
* @param source source
* @return the base64 decode of the given {@code source} as bytes array.
*/
public static byte[] base64Decode(byte[] source) {
return BaseEncoding.base64().decode(utf8decode(source));
}
/**
* @param source source
* @return the base64 encode of the given {@code source} as bytes array.
*/
public static String base64EncodeText(byte[] source) {
return BaseEncoding.base64().encode(source);
}
/**
* @param source source
* @return the base64 encode of the given {@code source} as bytes array.
*/
public static String base64UrlEncodeText(byte[] source) {
return BaseEncoding.base64Url().encode(source);
}
/**
* @param source source
* @return the base64 decode of the given {@code source} as string.
*/
public static byte[] base64DecodeText(String source) {
return BaseEncoding.base64().decode(source);
}
/**
* @param source source
* @return the base64 decode of the given {@code source} as string.
*/
public static byte[] base64UrlDecodeText(String source) {
return BaseEncoding.base64Url().decode(source);
}
/**
* @param bytes source
* @return the bytes array as hex string as lowercase character.
*/
public static String bytesToHex(byte[] bytes) {
return bytesToHex(bytes, true);
}
/**
* Creates Base64 MIME encoding stream from the underlying output stream.
*
* @param output the underlying output stream.
* @return the Base64 MIME encoding stream.
*/
public static OutputStream createMimeEncodingStream(OutputStream output) {
return BaseEncoding.base64().withSeparator(LINE_SEPARATORS, 76).encodingStream(new OutputStreamWriter(output));
}
/**
* Creates Base64 MIME decoding stream from the underlying input stream.
*
* @param input the underlying input stream.
* @return the Base64 MIME encoding stream.
*/
public static InputStream createMimeDecodingStream(InputStream input) {
return BaseEncoding.base64().withSeparator(LINE_SEPARATORS, 76).decodingStream(new InputStreamReader(input));
}
/**
* Creates Base64 PEM encoding stream from the underlying stream.
*
* @param output the underlying stream.
* @return the Base64 PEM encoding stream.
*/
public static OutputStream createPemEncodingStream(OutputStream output) {
return BaseEncoding.base64().withSeparator(LINE_SEPARATORS, 64).encodingStream(new OutputStreamWriter(output));
}
/**
* Creates Base64 PEM encoding stream from the underlying stream.
*
* @param input the underlying stream.
* @return the Base64 PEM encoding stream.
*/
public static InputStream createPemDecodingStream(InputStream input) {
return BaseEncoding.base64().withSeparator(LINE_SEPARATORS, 64).decodingStream(new InputStreamReader(input));
}
/**
* @param bytes bytes
* @param lowercase lowercase
* @return the bytes array as hex string dependence on the {@code lowercase} flag.
*/
public static String bytesToHex(byte[] bytes, boolean lowercase) {
char[] hexChars = new char[bytes.length * 2];
int value;
// move step.
int ms = lowercase ? 0 : 16;
for (int index = 0; index < bytes.length; index++) {
value = bytes[index] & 0x0FF;
hexChars[index * 2] = HEX_DIGIT[((value >>> 4) | ms)];
hexChars[index * 2 + 1] = HEX_DIGIT[((value & 0x0F) | ms)];
}
return new String(hexChars);
}
/**
*
* @param hex hex
* @return the hex string as bytes array.
*/
public static byte[] hexToBytes(String hex) {
int len = hex.length();
if (len % 2 != 0) throw new IllegalArgumentException("The hex value: [" + hex + "] is not valid.");
byte[] bytes = new byte[len / 2];
for (int index = 0; index < len; index += 2) {
bytes[index / 2] = (byte) ((Character.digit(hex.charAt(index), 16) << 4) | Character.digit(hex.charAt(index + 1), 16));
}
return bytes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy