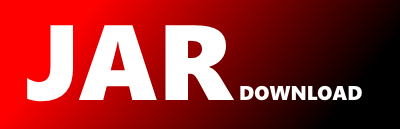
org.qas.api.transform.JsonErrorUnmarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.api.transform;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.api.internal.util.json.JsonObject;
import java.util.logging.Logger;
/**
* JsonErrorUnmarshaller
*
* @author Dzung Nguyen
* @version $Id JsonErrorUnmarshaller 2014-03-27 11:12:30z dungvnguyen $
* @since 1.0
*/
public class JsonErrorUnmarshaller extends AbstractErrorUnmarshaller {
private static final Logger LOG = Logger.getLogger(JsonErrorUnmarshaller.class.getName());
//~ class members ===========================================================
public JsonErrorUnmarshaller() {}
protected JsonErrorUnmarshaller(Class extends AuthServiceException> exceptionClass) {
super(exceptionClass);
}
public AuthServiceException unmarshall(JsonObject json) throws Exception {
LOG.info("Unmarshall " + json);
String message = parseMessage(json);
String errorCode = parseErrorCode(json);
String requestId = parseRequestId(json);
String type = parseType(json);
if ((null == message || message.isEmpty()) && (null == errorCode || errorCode.isEmpty())) {
throw new AuthClientException("Neither error message nor error code is found in the error response payload.");
} else {
AuthServiceException ase = newException(message);
ase.setErrorCode(errorCode);
ase.setRequestId(requestId);
if ("Client".equals(type)) {
ase.setErrorType(AuthServiceException.ErrorType.Client);
} else if ("Server".equals(type)) {
ase.setErrorType(AuthServiceException.ErrorType.Service);
} else {
ase.setErrorType(AuthServiceException.ErrorType.Unknown);
}
return ase;
}
}
protected String parseMessage(JsonObject json) throws Exception {
String message = "";
if (json.has("message")) {
message = json.getString("message");
} else if (json.has("Message")) {
message = json.getString("Message");
} else if (json.has("error_description")) {
message = json.getString("error_description");
}
return message;
}
protected String parseErrorCode(JsonObject json) throws Exception {
if (json.has("code")) {
return json.getString("code");
} else if (json.has("error")) {
return json.getString("error");
}
return null;
}
protected String parseRequestId(JsonObject json) throws Exception {
if (json.has("request_id")) {
return json.getString("request_id");
}
return null;
}
protected String parseType(JsonObject json) throws Exception {
if (json.has("type")) {
return json.getString("type");
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy