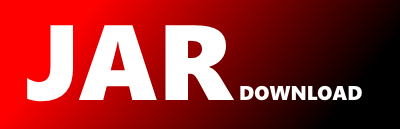
org.qas.qtest.api.Main Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api;
import org.qas.api.ClientConfiguration;
import org.qas.api.http.Protocol;
import org.qas.qtest.api.auth.BasicQTestCredentials;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.services.design.TestDesignServiceAsync;
import org.qas.qtest.api.services.design.TestDesignServiceAsyncClient;
import org.qas.qtest.api.services.design.model.ListTestCaseRequest;
import org.qas.qtest.api.services.design.model.TestCase;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.Properties;
/**
* Main
*
* @author Dzung Nguyen
* @version $Id Main 2015-06-04 16:21:30z dzungvnguyen $
* @since 1.0
*/
public class Main {
//~ class properties ========================================================
protected static final String CONFIG_FILE = "qtest-test.properties";
private static TestDesignServiceAsync testDesignService;
private static Long projectId = -1L;
//~ class members ===========================================================
public static void main(String[] args) throws Exception {
if (testDesignService == null) {
testDesignService = createTestDesignService();
}
ListTestCaseRequest testCaseRequest = new ListTestCaseRequest().withProjectId(20799L);
testCaseRequest.withPage(1L);
List testcases = testDesignService.listTestCase(testCaseRequest);
System.out.println("Size = " + testcases.size());
for (TestCase tc : testcases) {
System.out.println(tc);
}
}
protected static TestDesignServiceAsync createTestDesignService() throws IOException {
ClientConfiguration clientConfiguration = createClientConfiguration();
return new TestDesignServiceAsyncClient(getCredentials(clientConfiguration), clientConfiguration);
}
/**
* @return the {@link ClientConfiguration client configuration} object.
*/
protected static ClientConfiguration createClientConfiguration() throws IOException {
// load the configuration file.
InputStream inputStream = ClientConfiguration.class.getClassLoader().getResourceAsStream(CONFIG_FILE);
if (inputStream == null) {
throw new FileNotFoundException(CONFIG_FILE + " not found on classpath.");
}
// create client configuration.
Properties configuration = new Properties();
configuration.load(inputStream);
// construct the default client configuration.
ClientConfiguration clientConfig = new ClientConfiguration()
.withProtocol(Protocol.valueOf(configuration.getProperty("client.protocol", "HTTP")))
.withMaxConnections(Integer.parseInt(configuration.getProperty("client.max-connection", "10"), 10))
.withMaxErrorRetry(Integer.parseInt(configuration.getProperty("client.max-error-retry", "3"), 10))
.withProperty("service.endpoint", configuration.getProperty("service.endpoint", "nephele.qtestnet.com"))
.withProperty("qTest.projectId", Long.parseLong(configuration.getProperty("qTest.projectId", "-1"), 10))
.withProperty("qTest.token", configuration.getProperty("qTest.token"))
.withProperty("qTest.engineClass", configuration.getProperty("qTest.engineClass"));
projectId = (Long) clientConfig.getProperty("qTest.projectId");
return clientConfig;
}
/**
* @return the current project identifier.
*/
protected static Long getProjectId() {
return (projectId == null ? 1L : projectId);
}
/**
* @return the current qTest credentials.
*/
protected static QTestCredentials getCredentials(ClientConfiguration clientConfiguration) {
return new BasicQTestCredentials((String) clientConfiguration.getProperty("qTest.token"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy