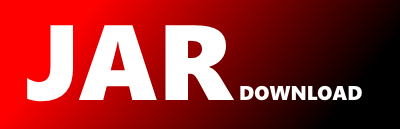
org.qas.qtest.api.internal.QTestApiWebServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.internal;
import org.qas.api.*;
import org.qas.api.auth.Signer;
import org.qas.api.http.ExecutionContext;
import org.qas.api.http.JsonErrorResponseHandler;
import org.qas.api.http.JsonResponseHandler;
import org.qas.api.internal.util.json.JsonObject;
import org.qas.api.transform.JsonErrorUnmarshaller;
import org.qas.api.transform.JsonUnmarshallerContext;
import org.qas.api.transform.Unmarshaller;
import org.qas.qtest.api.auth.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ExecutorService;
/**
* QTestApiWebServiceClient
*
* @author Dzung Nguyen
* @version $Id QTestApiWebServiceClient 2014-03-27 17:47:30z dungvnguyen $
* @since 1.0
*/
public abstract class QTestApiWebServiceClient>
extends ApiWebServiceClient {
protected QTestCredentialsProvider credentialsProvider;
protected Signer signer;
protected final List> exceptionUnmarshallers = new ArrayList<>();
protected ExecutorService executorService;
/**
* Constructs a new client to invoke service method on DefectService using
* the default qTest credentials provider and default client configuration options.
*/
public QTestApiWebServiceClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on DefectService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to DefectService
*/
public QTestApiWebServiceClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public QTestApiWebServiceClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to DefectService
*/
public QTestApiWebServiceClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = new StaticQTestCredentialsProvider(credentials);
init();
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
*/
public QTestApiWebServiceClient(QTestCredentialsProvider credentialsProvider) {
this(credentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to DefectService
*/
public QTestApiWebServiceClient(QTestCredentialsProvider credentialsProvider, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = credentialsProvider;
init();
}
protected void init() {
exceptionUnmarshallers.add(new JsonErrorUnmarshaller());
// set the endpoint.
String endpoint = (String) configuration.getProperty("service.endpoint");
if (endpoint == null || "".equals(endpoint.trim())) {
endpoint = "nephele.qtestnet.com";
}
setEndpoint(endpoint);
// set qTest1 signer
signer = new QTest1Signer();
}
protected X invoke(Request request, Unmarshaller unmarshaller) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
for (Map.Entry entry : request.getOriginalRequest().copyPrivateRequestParameters().entrySet()) {
request.addParameter(entry.getKey(), entry.getValue());
}
QTestCredentials credentials = credentialsProvider.getCredentials();
ApiServiceRequest originalRequest = request.getOriginalRequest();
if (originalRequest != null && originalRequest.getCredentials() != null) {
credentials = (QTestCredentials) originalRequest.getCredentials();
}
ExecutionContext executionContext = createExecutionContext();
executionContext.setSigner(signer);
executionContext.setCredentials(credentials);
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
JsonErrorResponseHandler errorResponseHandler = new JsonErrorResponseHandler(exceptionUnmarshallers);
return (X) client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
/**
* Sets the service endpoint.
*
* @param endpoint the given service endpoint URL.
* @return the current web service client.
* @throws IllegalArgumentException if an error occurs during setting the endpoint.
*/
public QTestApiWebServiceClient withEndpoint(String endpoint) throws IllegalArgumentException {
setEndpoint(endpoint);
return this;
}
@Override
public void shutdown() {
if (null != executorService) {
executorService.shutdown();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy