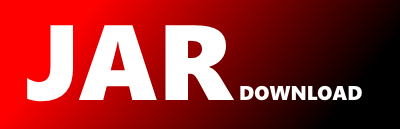
org.qas.qtest.api.internal.model.AllowedValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.internal.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.api.JsonMapper;
import org.qas.api.internal.PropertyContainer;
/**
* AllowedValue
*
* @author Dzung Nguyen
* @version $Id AllowedValue 2014-05-17 23:16:30z dungvnguyen $
* @since 1.0
*/
public class AllowedValue extends PropertyContainer {
@JsonProperty("value")
private Long value;
@JsonProperty("ext_value")
private String extValue;
@JsonProperty("label")
private String label;
@JsonProperty("order")
private Integer order;
@JsonProperty("is_default")
private Boolean isDefault;
@JsonProperty("is_active")
private Boolean active;
/**
* Creates {@link AllowedValue} instance.
*/
public AllowedValue() {
setActive(Boolean.TRUE);
}
/**
* @return the allowed value.
*/
public Long getValue() {
return value;
}
/**
* Sets the allowed value.
*
* @param value the given allowed value to set.
* @return this
*/
public AllowedValue setValue(Long value) {
this.value = value;
return this;
}
/**
* Sets the allowed value and return itself.
*
* @param value the given allowed value to set.
* @return the current instance.
*/
public AllowedValue withValue(Long value) {
setValue(value);
return this;
}
/**
* @return the allowed external value.
*/
public String getExtValue() {
return extValue;
}
/**
* Sets the allowed external value.
*
* @param value the given allowed external value to set.
* @return the current instance.
*/
public AllowedValue setExtValue(String value) {
this.extValue = value;
return this;
}
/**
* Sets the allowed external value and return itself.
*
* @param value the given allowed external value to set.
* @return the current instance.
*/
public AllowedValue withExtValue(String value) {
setExtValue(value);
return this;
}
/**
* @return the allowed value label.
*/
public String getLabel() {
return label;
}
/**
* Sets the allowed value lable.
*
* @param label the given allowed value label to set.
* @return the current instance.
*/
public AllowedValue setLabel(String label) {
this.label = label;
return this;
}
/**
* Sets the allowed value label and return the itself.
*
* @param label the given allowed value label to set.
* @return the current instance.
*/
public AllowedValue withLabel(String label) {
setLabel(label);
return this;
}
/**
* @return the allowed value order.
*/
public Integer getOrder() {
return order;
}
/**
* Sets allowed value order.
*
* @param order the given allowed value order to set.
* @return the current instance.
*/
public AllowedValue setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the allowed value order and return itself.
*
* @param order the given allowed value order to set.
* @return the current instance.
*/
public AllowedValue withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the flag that mark this allowed value is a default or not.
*/
public Boolean isDefault() {
return isDefault;
}
/**
* Sets the default flag.
*
* @param isDefault the default flag value to set.
* @return the current instance.
*/
public AllowedValue setDefault(Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
/**
* Sets the default flag value and return itself.
*
* @param isDefault the given default flag value to set.
* @return the allowed value instance.
*/
public AllowedValue withDefault(Boolean isDefault) {
setDefault(isDefault);
return this;
}
/**
* @return if the allowed value is actived.
*/
public Boolean isActive() {
return active;
}
/**
* Sets the allowed value active flag.
*
* @param active the given active flag to set.
* @return the current instance.
*/
public AllowedValue setActive(Boolean active) {
this.active = active;
return this;
}
/**
* Sets the allowed value active flag.
*
* @param active the given active flag to set.
* @return current allowed value instance.
*/
public AllowedValue withActive(Boolean active) {
setActive(active);
return this;
}
@Override
protected boolean isVisible(String key) {
if ("ext_value".equals(key)) return false;
return super.isVisible(key);
}
@Override
@JsonIgnore
public AllowedValue clone() {
AllowedValue that = new AllowedValue();
that.setPropertiesFrom(this);
return that;
}
@Override
@JsonIgnore
public String elementName() {
return "allowed-value";
}
@Override
public String toString() {
return JsonMapper.toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy