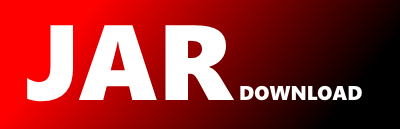
org.qas.qtest.api.internal.model.Field Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.internal.model;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.api.JsonMapper;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
/**
* Field
*
* @author Dzung Nguyen
* @version $Id Field 2014-05-18 14:01:30z dungvnguyen $
* @since 1.0
*/
public class Field extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("label")
private String label;
@JsonProperty("original_name")
private String originName;
@JsonProperty("required")
private Boolean required;
@JsonProperty("constrained")
private Boolean constrained;
@JsonProperty("searchable")
private Boolean searchable;
@JsonProperty("free_text_search")
private Boolean freeTextSearch;
@JsonProperty("search_key")
private String searchKey;
@JsonProperty("data_type")
private int dataTypeId;
@JsonProperty("order")
private Integer order;
@JsonProperty("multiple")
private Boolean multiple;
@JsonProperty("attribute_type")
private String attributeType;
@JsonProperty("system_field")
private Boolean systemField;
@JsonProperty("allowed_values")
List allowedValues;
@JsonProperty("is_active")
private Boolean active;
@JsonIgnore
private DataType dataType;
/**
* Creates the field instance.
*/
public Field() {
// set default value.
setMultiple(Boolean.FALSE);
setRequired(Boolean.FALSE);
setConstrained(Boolean.FALSE);
}
/**
* @return the field identifier value.
*/
public Long getId() {
return id;
}
/**
* Sets the field identifier value.
*
* @param id the given field identifier value to set.
* @return the current instance.
*/
public Field setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the field identifier value and return itself.
*
* @param id the given field identifier value to set.
* @return the field instance.
*/
public Field withId(Long id) {
setId(id);
return this;
}
/**
* @return the field label value.
*/
public String getLabel() {
return label;
}
/**
* Sets field label.
*
* @param label the given field label value to set.
* @return the current instance.
*/
public Field setLabel(String label) {
this.label = label;
return this;
}
/**
* Sets the field label value and return itself.
*
* @param label the given field label value.
* @return the current field instance.
*/
public Field withLabel(String label) {
setLabel(label);
return this;
}
/**
* @return originName
*/
public String getOriginName() {
return originName;
}
/**
* @param originName originName
* @return the current instance.
*/
public Field setOriginName(String originName) {
this.originName = originName;
return this;
}
/**
* @param originName originName
* @return the current instance.
*/
public Field withOriginName(String originName) {
this.originName = originName;
return this;
}
/**
* @return the flag that mark this field is required.
*/
public Boolean isRequired() {
return required;
}
/**
* Sets the required flag.
*
* @param required the given required flag value to set.
* @return the current instance.
*/
public Field setRequired(Boolean required) {
this.required = required;
return this;
}
/**
* Sets the required flag and return itself.
*
* @param required the givne required flag value to set.
* @return the current field istance.
*/
public Field withRequired(Boolean required) {
return setRequired(required);
}
/**
* @return the field constrained flag.
*/
public Boolean isConstrained() {
return constrained;
}
/**
* Sets the field constrained value.
*
* @param constrained the given field constrained value to set.
* @return the current instance.
*/
public Field setConstrained(Boolean constrained) {
this.constrained = constrained;
return this;
}
/**
* Sets the field constrained value and return itself.
*
* @param constrained the given field constrained value to set.
* @return the field instance.
*/
public Field withConstrained(Boolean constrained) {
setConstrained(constrained);
return this;
}
/**
* @return if the field can searchable.
*/
public Boolean isSearchable() {
return searchable;
}
/**
* Sets the field searchable flag value.
*
* @param searchable the given searchable flag value to set.
* @return the current instance.
*/
public Field setSearchable(Boolean searchable) {
this.searchable = searchable;
return this;
}
/**
* Sets the field searchable flag value and return itself.
*
* @param searchable the given searchable flag value to set.
* @return the current field instance.
*/
public Field withSearchable(Boolean searchable) {
setSearchable(searchable);
return this;
}
/**
* @return if the field support free text search.
*/
public Boolean isFreeTextSearch() {
return freeTextSearch;
}
/**
* Sets the free text search flag value.
*
* @param freeTextSearch the given free text search flag value to set.
* @return the current instance.
*/
public Field setFreeTextSearch(Boolean freeTextSearch) {
this.freeTextSearch = freeTextSearch;
return this;
}
/**
* Sets the free text search flag value and return current field instance.
*
* @param freeTextSearch the given free text search flag value to set.
* @return current field instance.
*/
public Field withFreeTextSearch(Boolean freeTextSearch) {
setFreeTextSearch(freeTextSearch);
return this;
}
/**
* @return the field search key.
*/
public String getSearchKey() {
return searchKey;
}
/**
* Sets the field search key value.
*
* @param searchKey the given search key value to set.
* @return the current instance.
*/
public Field setSearchKey(String searchKey) {
this.searchKey = searchKey;
return this;
}
/**
* Sets the field search key value and return itself.
*
* @param searchKey the given search key value to set.
* @return current field instance.
*/
public Field withSearchKey(String searchKey) {
setSearchKey(searchKey);
return this;
}
public int getDataTypeId() {
if (null != dataType) {
return dataType.getId();
}
return dataTypeId;
}
/**
* @return the current field data type.
*/
@JsonGetter("data_type_name")
public DataType getDataType() {
return dataType;
}
public Field setDataTypeId(int dataTypeId) {
this.dataTypeId = dataTypeId;
this.dataType = DataType.matches(dataTypeId);
return this;
}
/**
* Sets the field data type.
*
* @param dataType the given field data type.
* @return the current instance.
*/
public Field setDataType(DataType dataType) {
this.dataType = dataType;
if (null != dataType) {
dataTypeId = dataType.getId();
}
return this;
}
/**
* Sets the field data type and return current instance.
*
* @param dataType the given field data type.
* @return the current instance.
*/
public Field withDataType(DataType dataType) {
setDataType(dataType);
return this;
}
/**
* @return the field order.
*/
public Integer getOrder() {
return order;
}
/**
* Sets the field order.
*
* @param order the given field order value to set.
* @return the current instance.
*/
public Field setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the field order and return itself.
*
* @param order the given field order value to set.
* @return the current field instance.
*/
public Field withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the multiple value.
*/
public Boolean isMultiple() {
return multiple;
}
/**
* Sets the multiple flag value.
*
* @param multiple the given multiple flag value to set.
* @return the current instance.
*/
public Field setMultiple(Boolean multiple) {
this.multiple = multiple;
return this;
}
/**
* Sets the multiple flag value and return itself.
*
* @param multiple the given multiple flag value to set.
* @return the current field instance.
*/
public Field withMultiple(Boolean multiple) {
setMultiple(multiple);
return this;
}
/**
* @return the field attribute type.
*/
public String getAttributeType() {
return attributeType;
}
/**
* Sets the attribute type.
*
* @param attributeType the given attribute type to set.
* @return the current instance.
*/
public Field setAttributeType(String attributeType) {
this.attributeType = attributeType;
return this;
}
/**
* Sets the attribute type value and return itself.
*
* @param attributeType the given attribute type value to set.
* @return the field instance.
*/
public Field withAttributeType(String attributeType) {
setAttributeType(attributeType);
return this;
}
/**
* @return if the field is system field.
*/
public Boolean isSystemField() {
return systemField;
}
/**
* Sets the system field flag.
*
* @param systemField the given flag that mark the field is system field or not.
* @return the current instance.
*/
public Field setSystemField(Boolean systemField) {
this.systemField = systemField;
return this;
}
/**
* Sets the system field flag and return field instance.
*
* @param systemField the given flag that mark the field is system field or not.
* @return current field instance.
*/
public Field withSystemField(Boolean systemField) {
setSystemField(systemField);
return this;
}
/**
* @return
*/
public Boolean isActive() {
return active;
}
/**
* @param active active
* @return the current instance.
*/
public Field setActive(Boolean active) {
this.active = active;
return this;
}
/**
* @param active active
* @return the current instance.
*/
public Field withActive(Boolean active) {
this.active = active;
return this;
}
/**
* Return the list of allowed values.
*
* @return
*/
public List getAllowedValues() {
if (null == allowedValues) {
return Collections.emptyList();
}
return allowedValues;
}
/**
* Sets the list of allowed value objects.
*
* @param allowedValues the given the list of allowed value objects to set.
* @return the current instance.
*/
public Field setAllowedValues(List allowedValues) {
this.allowedValues = allowedValues;
return this;
}
/**
* Sets the list of allowed value objects and return itself.
*
* @param allowedValues the given list of allowed value objects to set.
* @return the current field instance.
*/
public Field withAllowedValues(List allowedValues) {
setAllowedValues(allowedValues);
return this;
}
/**
* Add allowed value to field.
*
* @param allowedValue the given allowed value object to add.
* @return the current field instance.
*/
public Field addAllowedValue(AllowedValue allowedValue) {
if (allowedValues == null) {
allowedValues = new LinkedList<>();
}
allowedValues.add(allowedValue);
return this;
}
@Override
@JsonIgnore
public Field clone() {
Field that = new Field();
that.setPropertiesFrom(this);
return that;
}
@Override
@JsonIgnore
public String elementName() {
return "field";
}
@Override
public String toString() {
return JsonMapper.toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy