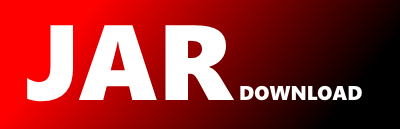
org.qas.qtest.api.internal.model.QTestBaseModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.internal.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.api.internal.PropertyContainer;
import org.qas.api.internal.util.StringInputStream;
import org.qas.api.internal.util.google.base.Throwables;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Collections;
import java.util.List;
/**
* QTestBaseModel
*
* @author Dzung Nguyen
* @version $Id QTestBaseModel 2014-03-28 05:16:30z dungvnguyen $
* @since 1.0
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
public abstract class QTestBaseModel> extends PropertyContainer {
@JsonProperty("links")
private List links;
@JsonProperty("web_url")
private String webUrl;
/**
* @return the list of relation's links.
*/
public List getLinks() {
if (links == null) {
return Collections.emptyList();
}
return links;
}
/**
* Sets the list of links relation.
*
* @param links the given list of relation's links to set.
* @return current instance.
*/
public T setLinks(List links) {
this.links = links;
return (T) this;
}
/**
* Sets the list of links relation.
*
* @param links the given list of relation's links to set.
* @return the current object.
*/
public QTestBaseModel withLinks(List links) {
setLinks(links);
return this;
}
/**
* @return the URL to access this object via web browser.
*/
public String getWebUrl() {
return webUrl;
}
/**
* Sets the object's web URL.
*
* @param webUrl the given URL to access object via web browser.
* @return current instance.
*/
public T setWebUrl(String webUrl) {
this.webUrl = webUrl;
return (T) this;
}
/**
* Sets the object's web URL and return itself.
*
* @param webUrl the given URL to access object via web browser.
* @return the current object.
*/
public QTestBaseModel withWebUrl(String webUrl) {
setWebUrl(webUrl);
return this;
}
/**
* @param content content
* @param markedSupport markedSupport
* @return the {@link InputStream content} as {@link InputStream} input stream.
* @throws IOException IOException
*/
protected byte[] getBinaryFromInputStream(InputStream content, boolean markedSupport) throws IOException {
try {
content = validateInputStream(content, markedSupport);
if (markedSupport) content.mark(-1);
ByteArrayOutputStream output = new ByteArrayOutputStream(1024);
byte[] buffer = new byte[8096];
int length;
while ((length = content.read(buffer)) > -1) {
output.write(buffer, 0, length);
}
output.close();
if (markedSupport) content.reset();
return output.toByteArray();
} catch (Exception ex) {
throw new IOException("Unable to read input stream: " + ex.getMessage(), ex);
}
}
/**
* @param content content
* @param markSupported markSupported
* @return InputStream
* @throws IOException IOException
*/
protected InputStream validateInputStream(InputStream content, boolean markSupported) throws IOException {
try {
if (content == null) return new ByteArrayInputStream(new byte[0]);
if (content instanceof StringInputStream) return content;
if (markSupported && !content.markSupported()) {
throw new IOException("Unable to read input stream. Not support mark/reset.");
}
return content;
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, IOException.class);
throw new IOException("Unable to read input stream: " + ex.getMessage(), ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy