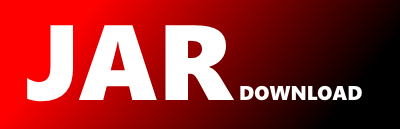
org.qas.qtest.api.services.attachment.AttachmentServiceAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.attachment;
import org.qas.api.AuthClientException;
import org.qas.api.ClientConfiguration;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.services.attachment.model.Attachment;
import org.qas.qtest.api.services.attachment.model.AttachmentRequest;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
/**
* AttachmentServiceAsyncClient
*
* @author Dzung Nguyen
* @version $Id AttachmentServiceAsyncClient 2014-05-19 17:19:30z dungvnguyen $
* @since 1.0
*/
public class AttachmentServiceAsyncClient extends AttachmentServiceClient
implements AttachmentServiceAsync {
/**
* Constructs a new client to invoke service method on AttachmentService using
* the default qTest credentials provider and default client configuration options.
*/
public AttachmentServiceAsyncClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the default qTest credentials provider and default client configuration options.
*
* @param executorService the executor service for executing asynchronous request.
*/
public AttachmentServiceAsyncClient(ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AttachmentService
*/
public AttachmentServiceAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AttachmentService.
* @param executorService the executor service for executing asynchronous request.
*/
public AttachmentServiceAsyncClient(ClientConfiguration clientConfiguration, ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public AttachmentServiceAsyncClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param executorService the executor service for executing asynchronous request.
*/
public AttachmentServiceAsyncClient(QTestCredentials credentials, ExecutorService executorService) {
this(credentials, new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AttachmentService
*/
public AttachmentServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
this(credentials, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AttachmentService
* @param executorService the executor service for executing asynchronous request.
*/
public AttachmentServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration,
ExecutorService executorService) {
this(new StaticQTestCredentialsProvider(credentials), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AttachmentService
*/
public AttachmentServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration) {
this(credentialsProvider, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on AttachmentService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AttachmentService
* @param executorService the executor service for executing asynchronous request.
*/
public AttachmentServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(credentialsProvider, clientConfiguration);
this.executorService = executorService;
}
//~ implements methods ======================================================
@Override
public Future attachAsync(final AttachmentRequest attachmentRequest) throws AuthClientException {
return executorService.submit(() -> attach(attachmentRequest));
}
@Override
public Future attachAsync(final AttachmentRequest attachmentRequest,
final AsyncHandler asyncHandler)
throws AuthClientException {
return executorService.submit(() -> {
Attachment attachment;
try {
attachment = attach(attachmentRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(attachmentRequest, attachment);
return attachment;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy