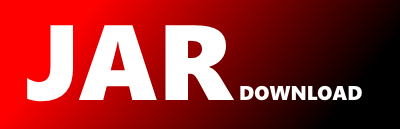
org.qas.qtest.api.services.attachment.model.Attachment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.attachment.model;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import org.qas.api.AuthClientException;
import org.qas.api.internal.util.Encoders;
import org.qas.api.internal.util.google.io.BaseEncoding;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
/**
* Attachment
*
* @author Dzung Nguyen
* @version $Id Attachment 2014-03-28 05:34:30z dungvnguyen $
* @since 1.0
*/
public class Attachment extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("name")
private String name;
@JsonProperty("content_type")
private String contentType;
@JsonProperty("data")
private String content;
@JsonIgnore
private InputStream inputStream;
//~ class members ===========================================================
public Attachment() {
}
/**
* @return the attachment identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the attachment identifier.
*
* @param id the given attachment identifier.
*/
public Attachment setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the attachment identifier.
*
* @param id the given attachment identifier to set.
* @return current {@link Attachment} object.
*/
public Attachment withId(Long id) {
setId(id);
return this;
}
/**
* @return the attachment name.
*/
public String getName() {
return name;
}
/**
* Sets the attachment name.
*
* @param name the given attachment name to set.
*/
public Attachment setName(String name) {
this.name = name;
return this;
}
/**
* Sets the attachment name.
*
* @param name the given attachment name to set.
* @return the current {@link Attachment} object.
*/
public Attachment withName(String name) {
setName(name);
return this;
}
/**
* @return attachment content type.
*/
public String getContentType() {
return contentType;
}
/**
* Sets the attachment content type.
*
* @param contentType the given attachment content type.
*/
public Attachment setContentType(String contentType) {
this.contentType = contentType;
return this;
}
/**
* Sets the attachment content type.
*
* @param contentType the given attachment content type.
*/
public Attachment withContentType(String contentType) {
setContentType(contentType);
return this;
}
/**
* @return
*/
@JsonGetter("data")
public String getContent() {
if (content == null) {
if (inputStream == null) {
return null;
}
try {
content = BaseEncoding.base64().encode(getBinaryFromInputStream(inputStream, false));
} catch (IOException e) {
throw new AuthClientException("Error while converting input stream", e);
}
}
return content;
}
@JsonSetter("data")
public Attachment setContent(String content) {
this.content = content;
if (null != content) {
this.inputStream = new ByteArrayInputStream(Encoders.base64DecodeText(content));
}
return this;
}
/**
* @return the attachment data.
*/
public InputStream getData() {
return inputStream;
}
/**
* Set attachment data.
*
* @param content the given attachment content to set.
*/
public Attachment setData(InputStream content) {
this.inputStream = content;
return this;
}
/**
* Set attachment data.
*
* @param content the given attachment content to set.
* @return current object.
*/
public Attachment withData(InputStream content) {
setData(content);
return this;
}
@Override
protected Attachment clone() {
Attachment that = new Attachment();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "attachment";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy