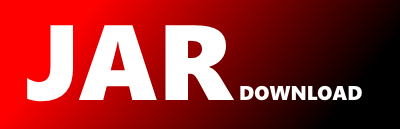
org.qas.qtest.api.services.attachment.model.AttachmentRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.attachment.model;
import org.qas.api.ApiServiceRequest;
import java.io.InputStream;
/**
* AttachmentRequest
*
* @author Dzung Nguyen
* @version $Id AttachmentRequest 2014-05-19 16:31:30z dungvnguyen $
* @since 1.0
*/
public class AttachmentRequest extends ApiServiceRequest {
//~ class properties ========================================================
private Long projectId;
private Long typeId;
private AttachmentType type;
private String fileName;
private String contentType;
private InputStream content;
//~ class members ===========================================================
/**
* @return the file name.
*/
public String getFileName() {
return fileName;
}
/**
* Sets the file name.
*
* @param fileName the given file name to upload.
*/
public void setFileName(String fileName) {
this.fileName = fileName;
}
/**
* Sets the file name.
*
* @param fileName the given file name to upload.
*/
public AttachmentRequest withFileName(String fileName) {
setFileName(fileName);
return this;
}
/**
* @return the content type.
*/
public String getContentType() {
return contentType;
}
/**
* Sets the content type.
*
* @param contentType the given content type value.
*/
public void setContentType(String contentType) {
this.contentType = contentType;
}
/**
* Sets the content type.
*
* @param contentType the given content type value to set.
* @return the attachment request instance.
*/
public AttachmentRequest withContentType(String contentType) {
setContentType(contentType);
return this;
}
/**
* @return the project identifier.
*/
public Long getProjectId() {
return projectId;
}
/**
* Sets the project identifier.
*
* @param projectId the given project identifier.
*/
public void setProjectId(Long projectId) {
this.projectId = projectId;
}
/**
* Sets the project identifier.
*
* @param projectId the given project identifier.
*/
public AttachmentRequest withProjectId(Long projectId) {
setProjectId(projectId);
return this;
}
/**
* @return the type identifier.
*/
public Long getTypeId() {
return this.typeId;
}
/**
* Sets the type identifier.
*
* @param typeId the given type identifier to set.
*/
public void setTypeId(Long typeId) {
this.typeId = typeId;
}
/**
* Sets the type identifier.
*
* @param typeId the given type identifier to set.
*/
public AttachmentRequest withTypeId(Long typeId) {
setTypeId(typeId);
return this;
}
/**
* @return the type such as (defect, test-case, requirement).
*/
public AttachmentType getType() {
return type;
}
/**
* Sets the type.
*
* @param type the given type to set.
*/
public void setType(AttachmentType type) {
this.type = type;
}
/**
* Sets the type.
*
* @param type the given type to set.
*/
public AttachmentRequest withType(AttachmentType type) {
this.setType(type);
return this;
}
/**
* @return the attachment request content.
*/
public InputStream getContent() {
return content;
}
/**
* Sets the content.
*
* @param content the given content to attachment.
*/
public void setContent(InputStream content) {
this.content = content;
}
/**
* Sets the content.
*
* @param content the given content to attachment.
*/
public AttachmentRequest withContent(InputStream content) {
setContent(content);
return this;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder(getClass().getName());
builder.append("{").append("\n")
.append("\tprojectId: ").append(projectId).append(", \n")
.append("\ttype: ").append(type).append(", \n")
.append("\ttypeId: ").append(typeId).append(", \n")
.append("\tfileName: ").append(fileName).append(", \n")
.append("\tcontentType: ").append(contentType).append("\n")
.append("}");
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy