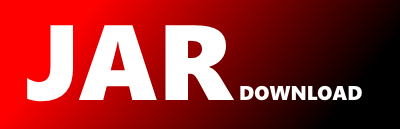
org.qas.qtest.api.services.authenticate.AuthenticateServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.authenticate;
import org.qas.api.*;
import org.qas.api.auth.NoNeedSigner;
import org.qas.api.http.*;
import org.qas.api.http.basic.HttpUrlConnectionAuthClient;
import org.qas.api.internal.util.google.base.Throwables;
import org.qas.api.internal.util.json.JsonObject;
import org.qas.api.transform.JsonErrorUnmarshaller;
import org.qas.api.transform.Unmarshaller;
import org.qas.qtest.api.internal.QTestApiWebServiceClient;
import org.qas.qtest.api.services.authenticate.model.*;
import org.qas.qtest.api.services.authenticate.model.transform.*;
import org.qas.qtest.api.services.user.model.User;
import org.qas.qtest.api.services.user.model.transform.UserJsonUnmarshaller;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* AuthenticateServiceClient
*
* @author Dzung Nguyen
* @version $Id AuthenticateServiceClient 2014-07-09 11:16:30z dungvnguyen $
* @since 1.0
*/
public class AuthenticateServiceClient extends QTestApiWebServiceClient
implements AuthenticateService {
/**
* Constructs a new client to invoke service method on AuthenticateService using
* the default qTest credentials provider and default client configuration options.
*/
public AuthenticateServiceClient() {
this(new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on AuthenticateService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to UserService
*/
public AuthenticateServiceClient(ClientConfiguration clientConfiguration) {
super(clientConfiguration);
init();
}
@Override
protected HttpAuthClient createHttpAuthClient(ClientConfiguration configuration) {
return new HttpUrlConnectionAuthClient(configuration);
}
private X invoke(Request request, HttpResponseHandler> responseHandler) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
for (Map.Entry entry : request.getOriginalRequest().copyPrivateRequestParameters().entrySet()) {
request.addParameter(entry.getKey(), entry.getValue());
}
ExecutionContext executionContext = createExecutionContext();
executionContext.setSigner(new NoNeedSigner());
executionContext.setCredentials(new Credentials() {});
// handle error.
List> exceptionsUnmarshallers = new ArrayList>();
exceptionsUnmarshallers.add(new JsonErrorUnmarshaller());
JsonErrorResponseHandler errorResponseHandler = new JsonErrorResponseHandler(exceptionsUnmarshallers);
return (X)client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@Override
public String authenticate(AuthenticateRequest authenticateRequest) throws AuthServiceException {
try {
// create request.
Request request = new AuthenticateRequestMarshaller().marshall(authenticateRequest);
// execute request.
return invoke(request, new StringResponseHandler());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown exception occurs during authenticate to qTest service.", ex);
}
}
@Override
public User validate(ValidateTokenRequest validateTokenRequest) throws AuthServiceException {
try {
// create request and invoke.
Request request = new ValidateTokenRequestMarshaller().marshall(validateTokenRequest);
return invoke(request, new JsonResponseHandler(UserJsonUnmarshaller.getInstance()));
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown exception occurs during validate token to qTest service.", ex);
}
}
@Override
public OAuthTokenResponse authenticate(OAuthAuthenticateRequest authenticateRequest) throws AuthServiceException {
try {
Request request = new OAuthAuthenticateRequestMarshaller().marshall(authenticateRequest);
// execute request.
return invoke(request, new JsonResponseHandler(OAuthTokenResponseJsonUnmarshaller.getInstance()));
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown exception occurs during authenticate to qTest service via OAuth.", ex);
}
}
@Override
public OAuthTokenStatusResponse status(OAuthTokenStatusRequest statusRequest) throws AuthServiceException {
try {
Request request = new OAuthTokenStatusRequestMarshaller().marshall(statusRequest);
// execute request.
return invoke(request, new JsonResponseHandler(OAuthTokenStatusResponseJsonUnmarshaller.getInstance()));
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown exception occurs during request status to qTest service via OAuth.", ex);
}
}
@Override
public OAuthTokenStatusResponse refresh(OAuthTokenRefreshRequest refreshRequest) throws AuthServiceException {
try {
Request request = new OAuthTokenRefreshRequestMarshaller().marshall(refreshRequest);
// execute request.
return invoke(request, new JsonResponseHandler(OAuthTokenStatusResponseJsonUnmarshaller.getInstance()));
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown exception occurs during refresh token to qTest service via OAuth.", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy