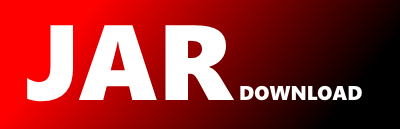
org.qas.qtest.api.services.authenticate.model.OAuthTokenResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.authenticate.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
/**
* OAuthTokenResponse
*
* @author Dzung Nguyen
* @version $Id OAuthTokenResponse 2016-03-07 18:18:30z dzungvnguyen $
* @since 1.0
*/
public final class OAuthTokenResponse extends QTestBaseModel {
@JsonProperty("access_token")
private String accessToken;
@JsonProperty("token_type")
private String tokenType;
@JsonProperty("refresh_token")
private String refreshToken;
@JsonProperty("expires_in")
private Integer expiredIn;
@JsonProperty("scope")
private String scope;
/**
* Creates {@link OAuthTokenResponse} instance.
*/
public OAuthTokenResponse() {
}
/**
* @return the access token value.
*/
public String getAccessToken() {
return accessToken;
}
/**
* Sets the access token.
*
* @param accessToken the given access token to set.
*/
public OAuthTokenResponse setAccessToken(String accessToken) {
this.accessToken = accessToken;
return this;
}
/**
* Sets the access token and return the current instance.
*
* @param accessToken the given access token to set.
* @return the current instance.
*/
public OAuthTokenResponse withAccessToken(String accessToken) {
setAccessToken(accessToken);
return this;
}
/**
* @return the token type.
*/
public String getTokenType() {
return tokenType;
}
/**
* Sets token type.
*
* @param tokenType the given token type to set.
*/
public OAuthTokenResponse setTokenType(String tokenType) {
this.tokenType = tokenType;
return this;
}
/**
* Sets the token type and return the current instance.
*
* @param tokenType the given token type to set.
* @return the current instance.
*/
public OAuthTokenResponse withTokenType(String tokenType) {
setTokenType(tokenType);
return this;
}
/**
* Obtains the refresh token.
*
* @return the refresh token.
*/
public String getRefreshToken() {
return refreshToken;
}
/**
* Sets the refresh token.
*
* @param refreshToken the refresh token.
*/
public OAuthTokenResponse setRefreshToken(String refreshToken) {
this.refreshToken = refreshToken;
return this;
}
/**
* Sets the refresh token.
*
* @param refreshToken the given refresh token to set.
* @return the current instance.
*/
public OAuthTokenResponse withRefreshToken(String refreshToken) {
setRefreshToken(refreshToken);
return this;
}
/**
* Obtains the expires in.
*
* @return the expires in.
*/
public Integer getExpiresIn() {
return expiredIn;
}
/**
* Sets the expires in.
*
* @param expiresIn the given expires in value.
*/
public OAuthTokenResponse setExpiresIn(Integer expiresIn) {
this.expiredIn = expiresIn;
return this;
}
/**
* Sets the expires in.
*
* @param expiresIn the given expires in.
* @return the {@link OAuthTokenResponse} instance.
*/
public OAuthTokenResponse withExpiresIn(Integer expiresIn) {
setExpiresIn(expiresIn);
return this;
}
/**
* Obtains the scope.
*
* @return the scope.
*/
public String getScope() {
return scope;
}
/**
* Sets the scope.
*
* @param scope the given scope to set.
*/
public OAuthTokenResponse setScope(String scope) {
this.scope = scope;
return this;
}
/**
* Sets the scope.
*
* @param scope the given scope.
* @return the {@link OAuthTokenResponse} instance.
*/
public OAuthTokenResponse withScope(String scope) {
setScope(scope);
return this;
}
@Override
public OAuthTokenResponse clone() {
OAuthTokenResponse that = new OAuthTokenResponse();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "token";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy