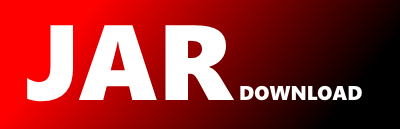
org.qas.qtest.api.services.client.ClientServiceAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.client;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.services.client.model.Client;
import org.qas.qtest.api.services.client.model.GetClientRequest;
import org.qas.qtest.api.services.client.model.SetSessionUrlRequest;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
/**
* ClientServiceAsyncClient
*
* @author Dzung Nguyen
* @version $Id ClientServiceAsyncClient 2014-11-11 13:48:30z dungvnguyen $
* @since 1.0
*/
public class ClientServiceAsyncClient extends ClientServiceClient
implements ClientServiceAsync {
/**
* Constructs a new client to invoke service method on ClientService using
* the default qTest credentials provider and default client configuration options.
*/
public ClientServiceAsyncClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ClientService using
* the default qTest credentials provider and default client configuration options.
*
* @param executorService the executor service for executing asynchronous request.
*/
public ClientServiceAsyncClient(ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on ClientService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to ClientService
*/
public ClientServiceAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ClientService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to ClientService.
* @param executorService the executor service for executing asynchronous request.
*/
public ClientServiceAsyncClient(ClientConfiguration clientConfiguration, ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on ClientService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public ClientServiceAsyncClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ClientService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param executorService the executor service for executing asynchronous request.
*/
public ClientServiceAsyncClient(QTestCredentials credentials, ExecutorService executorService) {
this(credentials, new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on ClientService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ClientService
*/
public ClientServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
this(credentials, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ClientService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ClientService
* @param executorService the executor service for executing asynchronous request.
*/
public ClientServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration,
ExecutorService executorService) {
this(new StaticQTestCredentialsProvider(credentials), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on ClientService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ClientService
*/
public ClientServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration) {
this(credentialsProvider, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ClientService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ClientService
* @param executorService the executor service for executing asynchronous request.
*/
public ClientServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(credentialsProvider, clientConfiguration);
this.executorService = executorService;
}
@Override
public Future getClientAsync(final GetClientRequest getClientRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Client call() throws Exception {
return getClient(getClientRequest);
}
});
}
@Override
public Future getClientAsync(final GetClientRequest getClientRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Client call() throws Exception {
Client result;
try {
result = getClient(getClientRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getClientRequest, result);
return result;
}
});
}
@Override
public Future setSessionUrlAsync(final SetSessionUrlRequest setSessionUrlRequest)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Void call() throws Exception {
setSessionUrl(setSessionUrlRequest);
return null;
}
});
}
@Override
public Future setSessionUrlAsync(final SetSessionUrlRequest setSessionUrlRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Void call() throws Exception {
try {
setSessionUrl(setSessionUrlRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(setSessionUrlRequest, null);
return null;
}
});
}
@Override
public void shutdown() {
super.shutdown();
executorService.shutdown();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy