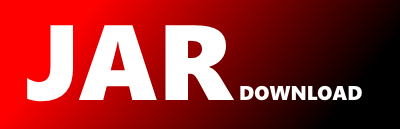
org.qas.qtest.api.services.defect.DefectServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.defect;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.Request;
import org.qas.api.internal.util.google.base.Throwables;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.internal.QTestApiWebServiceClient;
import org.qas.qtest.api.internal.model.CreateObjectCommentRequest;
import org.qas.qtest.api.internal.model.transform.CreateObjectCommentRequestMarshaller;
import org.qas.qtest.api.services.defect.model.CreateDefectRequest;
import org.qas.qtest.api.services.defect.model.Defect;
import org.qas.qtest.api.services.defect.model.transform.CreateDefectRequestMarshaller;
import org.qas.qtest.api.services.defect.model.transform.DefectJsonUnmarshaller;
/**
* DefectServiceClient
*
* @author Dzung Nguyen
* @version $Id DefectServiceClient 2014-07-16 17:30:30z dungvnguyen $
* @since 1.0
*/
public class DefectServiceClient extends QTestApiWebServiceClient
implements DefectService {
/**
* Constructs a new client to invoke service method on DefectService using
* the default qTest credentials provider and default client configuration options.
*/
public DefectServiceClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on DefectService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to DefectService
*/
public DefectServiceClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public DefectServiceClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to DefectService
*/
public DefectServiceClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = new StaticQTestCredentialsProvider(credentials);
init();
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
*/
public DefectServiceClient(QTestCredentialsProvider credentialsProvider) {
this(credentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on DefectService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to DefectService
*/
public DefectServiceClient(QTestCredentialsProvider credentialsProvider, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = credentialsProvider;
init();
}
@Override
public Defect createDefect(CreateDefectRequest createDefectRequest) throws AuthServiceException {
try {
Request request = new CreateDefectRequestMarshaller().marshall(createDefectRequest);
return invoke(request, DefectJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during creating request", ex);
}
}
@Override
public Defect addComment(CreateObjectCommentRequest createObjectCommentRequest) throws AuthServiceException {
try {
Request request = new CreateObjectCommentRequestMarshaller("DefectService").marshall(createObjectCommentRequest);
return invoke(request, DefectJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during creating defect comment", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy