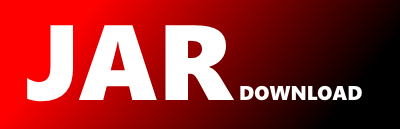
org.qas.qtest.api.services.defect.model.Defect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.defect.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.FieldValue;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import org.qas.qtest.api.services.attachment.model.Attachment;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* Defect
*
* @author Dzung Nguyen
* @version $Id Defect 2014-07-16 16:09:30z dungvnguyen $
* @since 1.0
*/
public class Defect extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("pid")
private String pid;
@JsonProperty("properties")
private List fieldValues;
@JsonProperty("attachments")
private List attachments;
public Defect() {
}
/**
* @return the defect identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the defect identifier.
*
* @param id the given defect identifier.
* @return current instance.
*/
public Defect setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the defect identifier and return itself.
*
* @param id the given defect identifier value to set.
* @return the defect instance.
*/
public Defect withId(Long id) {
setId(id);
return this;
}
/**
* @return the defect pid value.
*/
public String getPid() {
return pid;
}
/**
* Sets the defect pid value.
*
* @param pid the given defect pid value to set.
* @return current instance.
*/
public Defect setPid(String pid) {
this.pid = pid;
return this;
}
/**
* Sets the defect pid value.
*
* @param pid the given defect pid value to set.
* @return the defect instance.
*/
public Defect withPid(String pid) {
setPid(pid);
return this;
}
/**
* @return the list of field value objects.
*/
public List getFieldValues() {
if (null == fieldValues) {
return Collections.emptyList();
}
return fieldValues;
}
/**
* Sets the list of field value objects.
*
* @param values the given field value objects to set.
* @return current instance.
*/
public Defect setFieldValues(List values) {
this.fieldValues = values;
return this;
}
/**
* Sets the list of field value objects.
*
* @param values the given field value objects to set.
* @return the defect instance.
*/
public Defect withFieldValues(List values) {
setFieldValues(values);
return this;
}
/**
* Adds the property value to defect and return itself.
*
* @param value the given field value to add.
* @return the defect instance.
*/
public Defect addFieldValue(FieldValue value) {
if (value == null || value.getValue() == null) {
return this;
}
if (fieldValues == null) {
fieldValues = new ArrayList<>();
}
fieldValues.add(value);
return this;
}
/**
* @return the list of testcase's attachments.
*/
public List getAttachments() {
if (null == attachments) {
return Collections.emptyList();
}
return attachments;
}
/**
* Sets the list of defect's attachments.
*
* @param attachments the given list of defect's attachment to set.
* @return current instance.
*/
public Defect setAttachments(List attachments) {
this.attachments = attachments;
return this;
}
/**
* Sets the list of defect's attachments.
*
* @param attachments the given list of defect's attachment to set.
* @return current instance.
*/
public Defect withAttachments(List attachments) {
setAttachments(attachments);
return this;
}
/**
* Adds attachment to defect.
*
* @param attachment the given attachment to add to defect.
* @return the {@link Defect} object.
*/
public Defect addAttachment(Attachment attachment) {
if (attachments == null) {
attachments = new ArrayList<>();
}
attachments.add(attachment);
return this;
}
@Override
public Defect clone() {
Defect that = new Defect();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "defect";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy