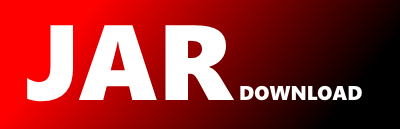
org.qas.qtest.api.services.defect.model.DefectLink Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.defect.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
/**
* DefectLink
*
* @author Dzung Nguyen
* @version $Id DefectLink 2014-03-28 06:28:30z dungvnguyen $
* @since 1.0
*/
public class DefectLink extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("external_defect_id")
private String externalDefectId;
@JsonProperty("conntection_id")
private Long connectionId;
@JsonProperty("external_project_id")
private String externalProjectId;
@JsonProperty("summary")
private String summary;
@JsonProperty("pid")
private String pid;
@JsonProperty("status")
private String status;
public DefectLink() {
}
/**
* @return the defect's link identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the defect's link identifier.
*
* @param id the given defect's link identifier to set.
* @return current instance.
*/
public DefectLink setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the defect's link identifier.
*
* @param id the given defect's link identifier to set.
* @return current defect's link object.
*/
public DefectLink withId(Long id) {
setId(id);
return this;
}
/**
* @return the id of defect in external defect tracking system.
*/
public String getExternalDefectId() {
return externalDefectId;
}
/**
* Sets the id of defect in external defect tracking system.
*
* @param externalDefectId the id of defect to set.
* @return current instance.
*/
public DefectLink setExternalDefectId(String externalDefectId) {
this.externalDefectId = externalDefectId;
return this;
}
/**
* Sets the id of defect in external defect tracking system.
*
* @param externalDefectId the id of defect to set.
* @return the current defect object.
*/
public DefectLink withExternalDefectId(String externalDefectId) {
setExternalDefectId(externalDefectId);
return this;
}
/**
* @return the id of defect tracking connection in qTest.
*/
public Long getConnectionId() {
return connectionId;
}
/**
* Sets the id of defect tracking connection in qTest.
*
* @param connectionId the given connection identifier to set.
* @return current instance.
*/
public DefectLink setConnectionId(Long connectionId) {
this.connectionId = connectionId;
return this;
}
/**
* Sets the id of defect tracking connection in qTest.
*
* @param connectionId the given connection identifier to set.
* @return the current defect object.
*/
public DefectLink withConnectionId(Long connectionId) {
setConnectionId(connectionId);
return this;
}
/**
* @return the external project identifier.
*/
public String getExternalProjectId() {
return externalProjectId;
}
/**
* Sets the external project identifier.
*
* @param externalProjectId the given external project identifier.
* @return current instance.
*/
public DefectLink setExternalProjectId(String externalProjectId) {
this.externalProjectId = externalProjectId;
return this;
}
/**
* Sets the external project identifier.
*
* @param externalProjectId the given external project identifier.
* @return current instance.
*/
public DefectLink withExternalProjectId(String externalProjectId) {
setExternalProjectId(externalProjectId);
return this;
}
/**
* Sets the defect link summary.
*
* @param summary the given defect link summary.
* @return current instance.
*/
public DefectLink setSummary(String summary) {
this.summary = summary;
return this;
}
/**
* Sets the defect link summary.
*
* @param summary the given summary.
* @return the current {@link DefectLink defect link} instance.
*/
public DefectLink withSummary(String summary) {
setSummary(summary);
return this;
}
/**
* @return the summary value.
*/
public String getSummary() {
return summary;
}
/**
* @return the defect PID.
*/
public String getPid() {
return pid;
}
/**
* Sets the PID.
*
* @param pid the given defect PID.
* @return current instance.
*/
public DefectLink setPid(String pid) {
this.pid = pid;
return this;
}
/**
* Sets the PID.
*
* @param pid the given defect PID.
* @return the current defect link instance.
*/
public DefectLink withPid(String pid) {
setPid(pid);
return this;
}
/**
* @return the defect status.
*/
public String getStatus() {
return status;
}
/**
* Sets status.
*
* @param status the given defect status to set.
* @return current instance.
*/
public DefectLink setStatus(String status) {
this.status = status;
return this;
}
/**
* Sets defect status.
*
* @param status the given defect status to set.
* @return the current instance.
*/
public DefectLink withStatus(String status) {
setStatus(status);
return this;
}
@Override
protected DefectLink clone() {
DefectLink that = new DefectLink();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "defect-link";
}
@Override
public String jsonElementName() {
return "defect-link";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy