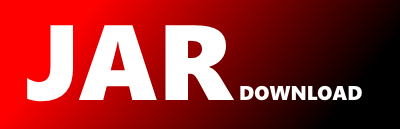
org.qas.qtest.api.services.design.TestDesignServiceAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.design;
import org.qas.api.AuthServiceException;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.internal.model.CreateObjectCommentRequest;
import org.qas.qtest.api.internal.model.Field;
import org.qas.qtest.api.services.design.model.*;
import org.qas.qtest.api.services.project.model.GetFieldsRequest;
import java.util.List;
import java.util.concurrent.Future;
/**
* TestDesignServiceAsync
*
* @author Dzung Nguyen
* @version $Id TestDesignServiceAsync 2014-04-06 05:10:30z dungvnguyen $
* @since 1.0
*/
public interface TestDesignServiceAsync extends TestDesignService {
/**
* Lists all test case in the current project.
*
* @param listTestCaseRequest the given {@link ListTestCaseRequest list test case request}
* object.
* @return the list of {@link TestCase test case} object.
* @throws AuthServiceException if an error occurs during listing all test case objects.
* @deprecated replace with {@link org.qas.qtest.api.services.project.ProjectServiceAsync#getFieldsAsync(GetFieldsRequest)}
*/
@Deprecated
Future> listTestCaseAsync(ListTestCaseRequest listTestCaseRequest) throws AuthServiceException;
/**
* Lists all test case in the current project.
*
* @param listTestCaseRequest the given {@link ListTestCaseRequest list test case request}
* object.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the list of {@link TestCase test case} object.
* @throws AuthServiceException if an error occurs during listing all test case objects.
* @deprecated replace with {@link org.qas.qtest.api.services.project.ProjectServiceAsync#getFieldsAsync(GetFieldsRequest, AsyncHandler)}
*/
@Deprecated
Future> listTestCaseAsync(ListTestCaseRequest listTestCaseRequest,
AsyncHandler> asyncHandler) throws AuthServiceException;
/**
* Gets {@link TestCase} from the given {@link GetTestCaseRequest test case request}
*
* @param testCaseRequest the given {@link GetTestCaseRequest test case request}
* @return the {@link TestCase} instance.
* @throws AuthServiceException if an error occurs during getting the test case.
*/
Future getTestCaseAsync(GetTestCaseRequest testCaseRequest) throws AuthServiceException;
/**
* Gets {@link TestCase} from the given {@link GetTestCaseRequest test case request}
*
* @param testCaseRequest the given {@link GetTestCaseRequest test case request}
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestCase} instance.
* @throws AuthServiceException if an error occurs during getting the test case.
*/
Future getTestCaseAsync(GetTestCaseRequest testCaseRequest,
AsyncHandler asyncHandler)
throws AuthServiceException;
/**
* Gets {@link TestStep} from the given {@link GetTestStepRequest test step request}
*
* @param testStepRequest the given {@link GetTestStepRequest test step request}
* @return the {@link TestStep} instance.
* @throws AuthServiceException if an error occurs during getting the test case.
*/
Future getTestStepAsync(GetTestStepRequest testStepRequest) throws AuthServiceException;
/**
* Gets {@link TestStep} from the given {@link GetTestStepRequest test step request}
*
* @param testStepRequest the given {@link GetTestStepRequest test step request}
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestStep} instance.
* @throws AuthServiceException if an error occurs during getting the test case.
*/
Future getTestStepAsync(GetTestStepRequest testStepRequest,
AsyncHandler asyncHandler)
throws AuthServiceException;
/**
* Gets list of {@link TestStep} from the given {@link org.qas.qtest.api.services.design.model.ListTestStepRequest test step request}
*
* @param testStepRequest the given {@link org.qas.qtest.api.services.design.model.ListTestStepRequest test step request}
* @return the list of {@link TestStep} instance.
* @throws AuthServiceException if an error occurs during getting the test step.
*/
Future> listTestStepAsync(ListTestStepRequest testStepRequest) throws AuthServiceException;
/**
* Gets list of {@link TestStep} from the given {@link org.qas.qtest.api.services.design.model.ListTestStepRequest test step request}
*
* @param testStepRequest the given {@link org.qas.qtest.api.services.design.model.ListTestStepRequest test step request}
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the list of {@link TestStep} instance.
* @throws AuthServiceException if an error occurs during getting the test step.
*/
Future> listTestStepAsync(ListTestStepRequest testStepRequest,
AsyncHandler> asyncHandler)
throws AuthServiceException;
/**
* Creates the {@link TestCase test case} from the given
* {@link CreateTestCaseRequest create test case request}
*
* @param testCaseRequest the given {@link CreateTestCaseRequest create test case request}
* instance.
* @return the {@link TestCase test case} instance.
* @throws AuthServiceException if an error occurs during creating test case instance.
*/
Future createTestCaseAsync(CreateTestCaseRequest testCaseRequest) throws AuthServiceException;
/**
* Creates the {@link TestCase test case} from the given
* {@link CreateTestCaseRequest create test case request}
*
* @param testCaseRequest the given {@link CreateTestCaseRequest create test case request}
* instance.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestCase test case} instance.
* @throws AuthServiceException if an error occurs during creating test case instance.
*/
Future createTestCaseAsync(CreateTestCaseRequest testCaseRequest,
AsyncHandler asyncHandler)
throws AuthServiceException;
/**
* Creates the {@link TestStep test step} from the given {@link CreateTestStepRequest create test step request}
*
* @param testStepRequest the given {@link CreateTestStepRequest create test step request}
* instance.
* @return the {@link TestStep test step} instance.
* @throws AuthServiceException if an error occurs during creating test step instance.
*/
Future createTestStepAsync(CreateTestStepRequest testStepRequest) throws AuthServiceException;
/**
* Creates the {@link TestStep test step} from the given {@link CreateTestStepRequest create test step request}
*
* @param testStepRequest the given {@link CreateTestStepRequest create test step request}
* instance.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestStep test step} instance.
* @throws AuthServiceException if an error occurs during creating test step instance.
*/
Future createTestStepAsync(CreateTestStepRequest testStepRequest,
AsyncHandler asyncHandler) throws AuthServiceException;
/**
* Gets the test-case custom fields.
*
* @param getTestCaseFieldsRequest the given {@link GetTestCaseFieldsRequest get testcase field request}
* information
* @return the list of test-case custom fields.
* @throws AuthServiceException if an error occurs during getting test-case custom fields.
*/
Future> getTestCaseFieldsAsync(GetTestCaseFieldsRequest getTestCaseFieldsRequest)
throws AuthServiceException;
/**
* Gets the test-case custom fields.
*
* @param getTestCaseFieldsRequest the given {@link GetTestCaseFieldsRequest get testcase field request}
* information.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the list of test-case custom fields.
* @throws AuthServiceException if an error occurs during getting test-case custom fields.
*/
Future> getTestCaseFieldsAsync(GetTestCaseFieldsRequest getTestCaseFieldsRequest,
AsyncHandler> asyncHandler)
throws AuthServiceException;
/**
* Creates testcase comment from the given request.
*
* @param createObjectCommentRequest the given {@link CreateObjectCommentRequest create testcase comment request}
* instance.
* @return the given {@link TestCase} instance.
* @throws AuthServiceException if an error occurs during adding comment.
*/
Future addCommentAsync(CreateObjectCommentRequest createObjectCommentRequest) throws AuthServiceException;
/**
* Creates testcase comment from the given request.
*
* @param createObjectCommentRequest the given {@link CreateObjectCommentRequest create testcase comment request}
* instance.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the given {@link TestCase} instance.
* @throws AuthServiceException if an error occurs during adding comment.
*/
Future addCommentAsync(CreateObjectCommentRequest createObjectCommentRequest,
AsyncHandler asyncHandler) throws AuthServiceException;
/**
* Creates the {@link CreateAutomationTestCaseRequest automation test case} from the given
* {@link CreateTestCaseRequest create automation test case request}
*
* @param automationTestCaseRequest the given {@link CreateAutomationTestCaseRequest create automation test case request}
* instance.
* @return the {@link AutomationTestCase automation test case} instance.
* @throws AuthServiceException if an error occurs during creating test case instance.
*/
Future createAutomationTestCaseAsync(CreateAutomationTestCaseRequest automationTestCaseRequest) throws AuthServiceException;
/**
* Creates the {@link CreateAutomationTestCaseRequest automation test case} from the given
* {@link CreateTestCaseRequest create automation test case request}
*
* @param automationTestCaseRequest the given {@link CreateAutomationTestCaseRequest create automation test case request}
* instance.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link AutomationTestCase automation test case} instance.
* @throws AuthServiceException if an error occurs during creating test case instance.
*/
Future createAutomationTestCaseAsync(CreateAutomationTestCaseRequest automationTestCaseRequest,
AsyncHandler asyncHandler) throws AuthServiceException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy