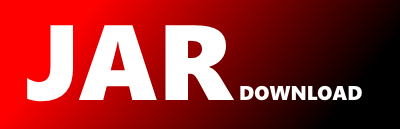
org.qas.qtest.api.services.design.TestDesignServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.design;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.Request;
import org.qas.api.internal.util.google.base.Throwables;
import org.qas.api.transform.VoidJsonUnmarshaller;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.internal.QTestApiWebServiceClient;
import org.qas.qtest.api.internal.model.CreateObjectCommentRequest;
import org.qas.qtest.api.internal.model.Field;
import org.qas.qtest.api.internal.model.transform.CreateObjectCommentRequestMarshaller;
import org.qas.qtest.api.internal.model.transform.ListFieldJsonUnmarshaller;
import org.qas.qtest.api.services.design.model.*;
import org.qas.qtest.api.services.design.model.transform.*;
import java.util.List;
/**
* TestDesignServiceClient
*
* @author Dzung Nguyen
* @version $Id TestDesignServiceClient 2014-04-04 05:04:30z dungvnguyen $
* @since 1.0
*/
public class TestDesignServiceClient extends QTestApiWebServiceClient
implements TestDesignService {
/**
* Constructs a new client to invoke service method on TestDesignService using
* the default qTest credentials provider and default client configuration options.
*/
public TestDesignServiceClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestDesignService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestDesignService
*/
public TestDesignServiceClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service method on TestDesignService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public TestDesignServiceClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestDesignService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestDesignService
*/
public TestDesignServiceClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = new StaticQTestCredentialsProvider(credentials);
init();
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
*/
public TestDesignServiceClient(QTestCredentialsProvider credentialsProvider) {
this(credentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestDesignService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestDesignService
*/
public TestDesignServiceClient(QTestCredentialsProvider credentialsProvider, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = credentialsProvider;
init();
}
//~ implements methods ======================================================
@Override
public TestCase getTestCase(GetTestCaseRequest testCaseRequest) throws AuthServiceException {
try {
Request request = new GetTestCaseRequestMarshaller().marshall(testCaseRequest);
return invoke(request, TestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute get test case request", ex);
}
}
@Override
public List listTestStep(ListTestStepRequest testStepRequest) throws AuthServiceException {
try {
Request request = new ListTestStepRequestMarshaller().marshall(testStepRequest);
return invoke(request, ListTestStepJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list test step request", ex);
}
}
@Override
public TestStep getTestStep(GetTestStepRequest testStepRequest) throws AuthServiceException {
try {
Request request = new GetTestStepRequestMarshaller().marshall(testStepRequest);
return invoke(request, TestStepJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute get test step request", ex);
}
}
@Override
public TestCase createTestCase(CreateTestCaseRequest testCaseRequest) throws AuthServiceException {
try {
Request request = new CreateTestCaseRequestMarshaller().marshall(testCaseRequest);
return invoke(request, TestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute create test case request", ex);
}
}
@Override
public TestStep createTestStep(CreateTestStepRequest testStepRequest) throws AuthServiceException {
try {
Request request = new CreateTestStepRequestMarshaller().marshall(testStepRequest);
return invoke(request, TestStepJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during executing create test step request", ex);
}
}
@Override
public List listTestCase(ListTestCaseRequest listTestCaseRequest) throws AuthServiceException {
try {
Request request = new ListTestCaseRequestMarshaller().marshall(listTestCaseRequest);
return invoke(request, ListTestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during executing listing test case request", ex);
}
}
@Override
public List getTestCaseFields(GetTestCaseFieldsRequest getTestCaseFieldsRequest) throws AuthServiceException {
try {
Request request = new GetTestCaseFieldsRequestMarshaller().marshall(getTestCaseFieldsRequest);
return invoke(request, ListFieldJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during getting testcase field", ex);
}
}
@Override
public TestCase addComment(CreateObjectCommentRequest createObjectCommentRequest) throws AuthServiceException {
try {
Request request = new CreateObjectCommentRequestMarshaller("TestDesignService").marshall(createObjectCommentRequest);
return invoke(request, TestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during creating testcase comment", ex);
}
}
@Override
public AutomationTestCase createAutomationTestCase(CreateAutomationTestCaseRequest automationTestCaseRequest)
throws AuthServiceException {
try {
Request request = new CreateAutomationTestCaseRequestMarshaller().marshall(automationTestCaseRequest);
return invoke(request, AutomationTestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute create test case request", ex);
}
}
@Override
public void deleteTestCase(DeleteTestCaseRequest testCaseRequest) throws AuthServiceException {
try {
Request request = new DeleteTestCaseRequestMarshaller().marshall(testCaseRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute delete test case request", ex);
}
}
@Override
public TestCase updateTestCase(UpdateTestCaseRequest testCaseRequest) throws AuthServiceException {
try {
Request request = new UpdateTestCaseRequestMarshaller().marshall(testCaseRequest);
return invoke(request, TestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute update test case request", ex);
}
}
@Override
public TestCase moveTestCase(MoveTestCaseRequest testCaseRequest) throws AuthServiceException {
try {
Request request = new MoveTestCaseRequestMarshaller().marshall(testCaseRequest);
return invoke(request, TestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute move test case request", ex);
}
}
@Override
public TestCase approveTestCase(ApproveTestCaseRequest testCaseRequest) throws AuthServiceException {
try {
Request request = new ApproveTestCaseRequestMarshaller().marshall(testCaseRequest);
return invoke(request, TestCaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during approve test case request", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy