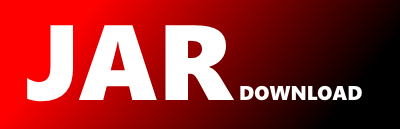
org.qas.qtest.api.services.design.model.TestStep Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.design.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.api.StringUtil;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import org.qas.qtest.api.services.attachment.model.Attachment;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* TestStep
*
* @author Dzung Nguyen
* @version $Id TestStep 2014-03-28 06:42:30z dungvnguyen $
* @since 1.0
*/
public class TestStep extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("description")
private String description;
@JsonProperty("expected")
private String expected;
@JsonProperty("order")
private Integer order;
@JsonProperty("group")
private Integer group;
@JsonProperty("called_test_case_name")
private String calledTestCaseName;
@JsonProperty("root_called_test_case_id")
private Long rootCalledTestCaseId;
@JsonProperty("attachments")
private List attachments;
@JsonProperty("parent_test_step_id")
private Long parentTestStepId;
public TestStep() {
setExpected(" ");
}
/**
* @return the test step id.
*/
public Long getId() {
return id;
}
/**
* Sets the test step id.
*
* @param id the given test step identifier to set.
*
* @return this
*/
public TestStep setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the test step id.
*
* @param id the given test step identifier to set.
*
* @return this
*/
public TestStep withId(Long id) {
setId(id);
return this;
}
/**
* @return the test step description.
*/
public String getDescription() {
return description;
}
/**
* Sets the test step description.
*
* @param description the given test step description to set.
*
* @return this
*/
public TestStep setDescription(String description) {
this.description = description;
return this;
}
/**
* Sets the test step description.
*
* @param description the given test step description to set.
*
* @return this
*/
public TestStep withDescription(String description) {
setDescription(description);
return this;
}
/**
* @return the expected result of an individual test step.
*/
public String getExpected() {
return expected;
}
/**
* Sets the expected result of an individual test step.
*
* @param expected the given expected result to set.
*
* @return this
*/
public TestStep setExpected(String expected) {
this.expected = StringUtil.isNullOrEmpty(expected) ? " " : expected;
return this;
}
/**
* Sets the expected result of an individual test step.
*
* @param expected the given expected result to set.
*
* @return this
*/
public TestStep withExpected(String expected) {
setExpected(expected);
return this;
}
/**
* @return the order of test step in test case.
*/
public Integer getOrder() {
return order;
}
/**
* Sets the order of test step in test case.
*
* @param order the given test step's order to set.
*
* @return this
*/
public TestStep setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the order of test step in test case.
*
* @param order the given test step's order to set.
*
* @return this
*/
public TestStep withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the test step group.
*/
public Integer getGroup() {
return group;
}
/**
* Sets the test step group.
*
* @param group the given test step group.
*
* @return this
*/
public TestStep setGroup(Integer group) {
this.group = group;
return this;
}
/**
* Sets the test step group.
*
* @param group the given test step group.
*
* @return this
*/
public TestStep withGroup(Integer group) {
setGroup(group);
return this;
}
/**
* @return the name of called test case if this step is a called test step.
*/
public String getCalledTestCaseName() {
return calledTestCaseName;
}
/**
* Sets called test case name.
*
* @param calledTestCaseName the given called test case name to set.
*
* @return this
*/
public TestStep setCalledTestCaseName(String calledTestCaseName) {
this.calledTestCaseName = calledTestCaseName;
return this;
}
/**
* Sets called test case name.
*
* @param calledTestCaseName the given called test case name to set.
*
* @return this
*/
public TestStep withCalledTestCaseName(String calledTestCaseName) {
setCalledTestCaseName(calledTestCaseName);
return this;
}
/**
* @return the called test case id.
*/
public Long getRootCalledTestCaseId() {
return rootCalledTestCaseId;
}
/**
* Sets the called test case id.
*
* @param rootCalledTestCaseId the given called test case id to set.
*
* @return this
*/
public TestStep setRootCalledTestCaseId(Long rootCalledTestCaseId) {
this.rootCalledTestCaseId = rootCalledTestCaseId;
return this;
}
/**
* Sets the called test case id.
*
* @param rootCalledTestCaseId the given called test case id to set.
*
* @return this
*/
public TestStep withRootCalledTestCaseId(Long rootCalledTestCaseId) {
setRootCalledTestCaseId(rootCalledTestCaseId);
return this;
}
/**
* @return the list of teststep's attachments.
*/
public List getAttachments() {
if (null == attachments) {
return Collections.emptyList();
}
return attachments;
}
/**
* Sets the list of teststep's attachments.
*
* @param attachments the given list of teststep's attachment to set.
*
* @return this
*/
public TestStep setAttachments(List attachments) {
this.attachments = attachments;
return this;
}
/**
* Sets the list of teststep's attachments.
*
* @param attachments the given list of teststep's attachment to set.
*
* @return this
*/
public TestStep withAttachments(List attachments) {
setAttachments(attachments);
return this;
}
/**
* Adds attachment to test step.
*
* @param attachment the given attachment to add to test step.
*
* @return the {@link TestStep} object.
*/
public TestStep addAttachment(Attachment attachment) {
if (attachments == null) {
attachments = new ArrayList<>();
}
attachments.add(attachment);
return this;
}
public Long getParentTestStepId() {
return parentTestStepId;
}
public TestStep setParentTestStepId(Long parentTestStepId) {
this.parentTestStepId = parentTestStepId;
return this;
}
public TestStep withParentTestStepId(Long parentTestStepId) {
this.parentTestStepId = parentTestStepId;
return this;
}
@Override
protected TestStep clone() {
TestStep that = new TestStep();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "test-step";
}
@Override
public String jsonElementName() {
return "test_step";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy