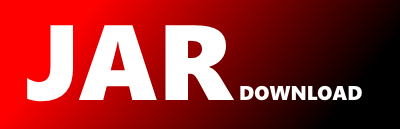
org.qas.qtest.api.services.execution.TestExecutionServiceAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution;
import org.qas.api.AuthServiceException;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.services.execution.model.*;
import java.util.List;
import java.util.concurrent.Future;
/**
* TestExecutionServiceAsync
*
* @author Dzung Nguyen
* @version $Id TestExecutionServiceAsync 2014-03-29 11:07:30z dungvnguyen $
* @since 1.0
*/
public interface TestExecutionServiceAsync extends TestExecutionService {
/**
* Submits automation test log to test execution service.
*
* @param automationTestLogRequest the given automation test log request.
* @return the {@link TestLog} instance.
* @throws org.qas.api.AuthServiceException if an error occurs during submitting automation
* test log to test execution service.
*/
Future submitAutomationTestLogAsync(AutomationTestLogRequest automationTestLogRequest)
throws AuthServiceException;
/**
* Submits automation test log to test execution service.
*
* @param automationTestLogRequest the given automation test log request.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestLog} instance.
* @throws org.qas.api.AuthServiceException if an error occurs during submitting automation
* test log to test execution service.
*/
Future submitAutomationTestLogAsync(AutomationTestLogRequest automationTestLogRequest,
AsyncHandler asyncHandler)
throws AuthServiceException;
/**
* Submits test log to test execution service.
*
* @param submitTestLogRequest the given test log request.
* @return the {@link TestLog} instance.
* @throws org.qas.api.AuthServiceException if an error occurs during submitting automation
* test log to test execution service.
*/
Future submitTestLogAsync(SubmitTestLogRequest submitTestLogRequest)
throws AuthServiceException;
/**
* Submits test log to test execution service.
*
* @param submitTestLogRequest the given test log request.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestLog} instance.
* @throws org.qas.api.AuthServiceException if an error occurs during submitting automation
* test log to test execution service.
*/
Future submitTestLogAsync(SubmitTestLogRequest submitTestLogRequest,
AsyncHandler asyncHandler)
throws AuthServiceException;
/**
* Gets test run information.
*
* @param getLastLogRequest the given {@link GetLastLogRequest} instance.
* @return the {@link TestLog} instance.
* @throws AuthServiceException if an error occurs during getting test-run information.
*/
Future getLastLogAsync(GetLastLogRequest getLastLogRequest) throws AuthServiceException;
/**
* Gets test run information.
*
* @param getLastLogRequest the given {@link GetLastLogRequest} instance.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestLog} instance.
* @throws AuthServiceException if an error occurs during getting test-run information.
*/
Future getLastLogAsync(GetLastLogRequest getLastLogRequest,
AsyncHandler asyncHandler) throws AuthServiceException;
/**
* List all execution status request from test execution service.
*
* @param executionStatusRequest the given {@link ListExecutionStatusRequest} object.
* @return the list of {@link ExecutionStatus execution status} instance.
* @throws AuthServiceException if an error occurs during listing all execution statuses.
*/
Future> listExecutionStatusAsync(ListExecutionStatusRequest executionStatusRequest) throws AuthServiceException;
/**
* List all execution status request from test execution service.
*
* @param executionStatusRequest the given {@link org.qas.qtest.api.services.execution.model.ListExecutionStatusRequest} object.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the list of {@link org.qas.qtest.api.services.execution.model.ExecutionStatus execution status} instance.
* @throws AuthServiceException if an error occurs during listing all execution statuses.
*/
Future> listExecutionStatusAsync(ListExecutionStatusRequest executionStatusRequest,
AsyncHandler> asyncHandler)
throws AuthServiceException;
/**
* Lists all test runs from the given {@link org.qas.qtest.api.services.execution.model.ListTestRunRequest list test run request}.
*
* @param testRunRequest the given {@link org.qas.qtest.api.services.execution.model.ListTestRunRequest list test run request} object.
* @return the list of {@link TestRun test run} objects.
* @throws AuthServiceException if an error occurs during listing all test run.
*/
Future> listTestRunAsync(ListTestRunRequest testRunRequest) throws AuthServiceException;
/**
* Lists all test runs from the given {@link org.qas.qtest.api.services.execution.model.ListTestRunRequest list test run request}.
*
* @param testRunRequest the given {@link org.qas.qtest.api.services.execution.model.ListTestRunRequest list test run request} object.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the list of {@link TestRun test run} objects.
* @throws AuthServiceException if an error occurs during listing all test run.
*/
Future> listTestRunAsync(ListTestRunRequest testRunRequest,
AsyncHandler> asyncHandler) throws AuthServiceException;
/**
* Gets test run information.
*
* @param getTestRunRequest the given {@link GetTestRunRequest} instance.
* @return the {@link TestRun} instance.
* @throws AuthServiceException if an error occurs during getting test-run information.
*/
Future getTestRunAsync(GetTestRunRequest getTestRunRequest) throws AuthServiceException;
/**
* Gets test run information.
*
* @param getTestRunRequest the given {@link GetTestRunRequest} instance.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the {@link TestRun} instance.
* @throws AuthServiceException if an error occurs during getting test-run information.
*/
Future getTestRunAsync(GetTestRunRequest getTestRunRequest,
AsyncHandler asyncHandler) throws AuthServiceException;
/**
* Lists all test suites from the given {@link ListTestSuiteRequest list test suite request}.
*
* @param testSuiteRequest the given {@link ListTestSuiteRequest list test suite request} object.
* @return the list of {@link TestSuite test suite} objects.
* @throws AuthServiceException if an error occurs during listing all test suites.
*/
Future> listTestSuiteAsync(ListTestSuiteRequest testSuiteRequest) throws AuthServiceException;
/**
* Lists all test suites from the given {@link ListTestSuiteRequest list test suite request}.
*
* @param testSuiteRequest the given {@link ListTestSuiteRequest list test suite request} object.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the list of {@link TestSuite test suite} objects.
* @throws AuthServiceException if an error occurs during listing all test suites.
*/
Future> listTestSuiteAsync(ListTestSuiteRequest testSuiteRequest,
AsyncHandler> asyncHandler) throws AuthServiceException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy