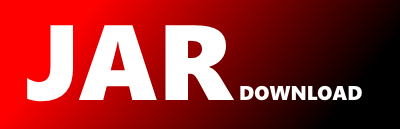
org.qas.qtest.api.services.execution.TestExecutionServiceAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.services.execution.model.*;
import org.qas.qtest.api.services.host.model.UpdateJobStatusRequest;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
/**
* TestExecutionServiceAsyncClient
*
* @author Dzung Nguyen
* @version $Id TestExecutionServiceAsyncClient 2014-03-29 11:10:30z dungvnguyen $
* @since 1.0
*/
public class TestExecutionServiceAsyncClient extends TestExecutionServiceClient
implements TestExecutionServiceAsync {
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the default qTest credentials provider and default client configuration options.
*/
public TestExecutionServiceAsyncClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the default qTest credentials provider and default client configuration options.
*
* @param executorService the executor service for executing asynchronous request.
*/
public TestExecutionServiceAsyncClient(ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
*/
public TestExecutionServiceAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService.
* @param executorService the executor service for executing asynchronous request.
*/
public TestExecutionServiceAsyncClient(ClientConfiguration clientConfiguration, ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public TestExecutionServiceAsyncClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param executorService the executor service for executing asynchronous request.
*/
public TestExecutionServiceAsyncClient(QTestCredentials credentials, ExecutorService executorService) {
this(credentials, new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
*/
public TestExecutionServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
this(credentials, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
* @param executorService the executor service for executing asynchronous request.
*/
public TestExecutionServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration,
ExecutorService executorService) {
this(new StaticQTestCredentialsProvider(credentials), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
*/
public TestExecutionServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration) {
this(credentialsProvider, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
* @param executorService the executor service for executing asynchronous request.
*/
public TestExecutionServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(credentialsProvider, clientConfiguration);
this.executorService = executorService;
}
@Override
public Future submitAutomationTestLogAsync(final AutomationTestLogRequest automationTestLogRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestLog call() throws Exception {
return submitAutomationTestLog(automationTestLogRequest);
}
});
}
@Override
public Future submitAutomationTestLogAsync(final AutomationTestLogRequest automationTestLogRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestLog call() throws Exception {
TestLog result;
try {
result = submitAutomationTestLog(automationTestLogRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(automationTestLogRequest, result);
return result;
}
});
}
@Override
public Future submitTestLogAsync(final SubmitTestLogRequest submitTestLogRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestLog call() throws Exception {
return submitTestLog(submitTestLogRequest);
}
});
}
@Override
public Future submitTestLogAsync(final SubmitTestLogRequest submitTestLogRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestLog call() throws Exception {
TestLog result;
try {
result = submitTestLog(submitTestLogRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(submitTestLogRequest, result);
return result;
}
});
}
@Override
public Future getLastLogAsync(final GetLastLogRequest getLastLogRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestLog call() throws Exception {
return getLastLog(getLastLogRequest);
}
});
}
@Override
public Future getLastLogAsync(final GetLastLogRequest getLastLogRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestLog call() throws Exception {
TestLog result;
try {
result = getLastLog(getLastLogRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getLastLogRequest, result);
return result;
}
});
}
@Override
public Future> listExecutionStatusAsync(final ListExecutionStatusRequest executionStatusRequest)
throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
return listExecutionStatus(executionStatusRequest);
}
});
}
@Override
public Future> listExecutionStatusAsync(final ListExecutionStatusRequest executionStatusRequest,
final AsyncHandler> asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
List result;
try {
result = listExecutionStatus(executionStatusRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(executionStatusRequest, result);
return result;
}
});
}
@Override
public Future> listTestRunAsync(final ListTestRunRequest testRunRequest) throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
return listTestRun(testRunRequest);
}
});
}
@Override
public Future> listTestRunAsync(final ListTestRunRequest testRunRequest, final AsyncHandler> asyncHandler) throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
List result;
try {
result = listTestRun(testRunRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(testRunRequest, result);
return result;
}
});
}
@Override
public Future getTestRunAsync(final GetTestRunRequest getTestRunRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestRun call() throws Exception {
return getTestRun(getTestRunRequest);
}
});
}
@Override
public Future getTestRunAsync(final GetTestRunRequest getTestRunRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public TestRun call() throws Exception {
TestRun result;
try {
result = getTestRun(getTestRunRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getTestRunRequest, result);
return result;
}
});
}
@Override
public Future> listTestSuiteAsync(final ListTestSuiteRequest testSuiteRequest) throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
return listTestSuite(testSuiteRequest);
}
});
}
@Override
public Future> listTestSuiteAsync(final ListTestSuiteRequest testSuiteRequest,
final AsyncHandler> asyncHandler) throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
List result;
try {
result = listTestSuite(testSuiteRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(testSuiteRequest, result);
return result;
}
});
}
@Override
public void shutdown() {
super.shutdown();
executorService.shutdown();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy