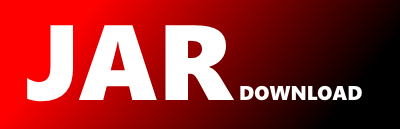
org.qas.qtest.api.services.execution.TestExecutionServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution;
import org.qas.api.*;
import org.qas.api.internal.util.google.base.Throwables;
import org.qas.api.transform.VoidJsonUnmarshaller;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.internal.QTestApiWebServiceClient;
import org.qas.qtest.api.services.execution.model.*;
import org.qas.qtest.api.services.execution.model.transform.*;
import java.util.List;
/**
* TestExecutionServiceClient
*
* @author Dzung Nguyen
* @version $Id TestExecutionServiceClient 2014-03-29 10:46:30z dungvnguyen $
* @since 1.0
*/
public class TestExecutionServiceClient extends QTestApiWebServiceClient
implements TestExecutionService {
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the default qTest credentials provider and default client configuration options.
*/
public TestExecutionServiceClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
*/
public TestExecutionServiceClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public TestExecutionServiceClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
*/
public TestExecutionServiceClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = new StaticQTestCredentialsProvider(credentials);
init();
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
*/
public TestExecutionServiceClient(QTestCredentialsProvider credentialsProvider) {
this(credentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestExecutionService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestExecutionService
*/
public TestExecutionServiceClient(QTestCredentialsProvider credentialsProvider, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = credentialsProvider;
init();
}
//~ implements methods ======================================================
@Override
public TestLog submitAutomationTestLog(AutomationTestLogRequest automationTestLogRequest) throws AuthServiceException {
try {
Request request = new AutomationTestLogRequestMarshaller().marshall(automationTestLogRequest);
return invoke(request, TestLogJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute request", ex);
}
}
@Override
public QueueProcessingResponse submitAutomationTestLogs(AutomationTestLogsRequest automationTestLogsRequest)
throws AuthServiceException {
try {
Request request = new AutomationTestLogsRequestMarshaller().marshall(automationTestLogsRequest);
return invoke(request, QueueProcessingResponseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute request", ex);
}
}
@Override
public QueueProcessingResponse submitAutomationTestLogsExtended(AutomationTestLogsRequest automationTestLogsRequest)
throws AuthServiceException {
try {
Request request = new AutomationTestLogsExtendedRequestMarshaller().marshall(automationTestLogsRequest);
return invoke(request, QueueProcessingResponseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute request", ex);
}
}
@Override
public QueueProcessingResponse getQueueProcessingResponse(
QueueProcessingResponseRequest queueProcessingResponseRequest)
throws AuthServiceException {
try {
Request request = new QueueProcessingResponseRequestMarshaller().marshall(queueProcessingResponseRequest);
return invoke(request, QueueProcessingResponseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during get a Batch Test Log Submission job's state", ex);
}
}
@Override
public TestLog submitTestLog(SubmitTestLogRequest submitTestLogRequest) throws AuthServiceException {
try {
Request request = new SubmitTestLogRequestMarshaller().marshall(submitTestLogRequest);
return invoke(request, TestLogJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute request", ex);
}
}
@Override
public TestLog getLastLog(GetLastLogRequest getLastLogRequest) throws AuthServiceException {
try {
Request request = new GetLastLogRequestMarshaller().marshall(getLastLogRequest);
return invoke(request, TestLogJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during get last log", ex);
}
}
@Override
public List listExecutionStatus(ListExecutionStatusRequest executionStatusRequest) throws AuthServiceException {
try {
Request request = new ListExecutionStatusRequestMarshaller().marshall(executionStatusRequest);
return invoke(request, ListExecutionStatusJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list execution status request", ex);
}
}
//~ override test-run methods ===============================================
@Override
public List listTestRun(ListTestRunRequest testRunRequest) throws AuthServiceException {
try {
Request request = new ListTestRunRequestMarshaller().marshall(testRunRequest);
return invoke(request, ListTestRunJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list test run request", ex);
}
}
@Override
public TestRun getTestRun(GetTestRunRequest getTestRunRequest) throws AuthServiceException {
try {
Request request = new GetTestRunRequestMarshaller().marshall(getTestRunRequest);
return invoke(request, TestRunJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during get test-run request", ex);
}
}
@Override
public TestRun createTestRun(CreateTestRunRequest testRunRequest) throws AuthServiceException {
try {
Request request = new CreateTestRunRequestMarshaller().marshall(testRunRequest);
return invoke(request, TestRunJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during create test-run request", ex);
}
}
@Override
public TestRun updateTestRun(UpdateTestRunRequest testRunRequest) throws AuthServiceException {
try {
Request request = new UpdateTestRunRequestMarshaller().marshall(testRunRequest);
return invoke(request, TestRunJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during update test-run request", ex);
}
}
@Override
public TestRun moveTestRun(MoveTestRunRequest testRunRequest) throws AuthServiceException {
try {
Request request = new MoveTestRunRequestMarshaller().marshall(testRunRequest);
return invoke(request, TestRunJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during move test-run request", ex);
}
}
@Override
public void deleteTestRun(DeleteTestRunRequest testRunRequest) throws AuthServiceException {
try {
Request request = new DeleteTestRunRequestMarshaller().marshall(testRunRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during delete test-run request", ex);
}
}
//~ override test-suite methods =============================================
@Override
public TestSuite createTestSuite(CreateTestSuiteRequest testSuiteRequest) throws AuthServiceException {
try {
Request request = new CreateTestSuiteRequestMarshaller().marshall(testSuiteRequest);
return invoke(request, TestSuiteJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute create test suite request", ex);
}
}
@Override
public TestSuite updateTestSuite(UpdateTestSuiteRequest testSuiteRequest) throws AuthServiceException {
try {
Request request = new UpdateTestSuiteRequestMarshaller().marshall(testSuiteRequest);
return invoke(request, TestSuiteJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute update test suite request", ex);
}
}
@Override
public TestSuite moveTestSuite(MoveTestSuiteRequest testSuiteRequest) throws AuthServiceException {
try {
Request request = new MoveTestSuiteRequestMarshaller().marshall(testSuiteRequest);
return invoke(request, TestSuiteJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute move test suite request", ex);
}
}
@Override
public TestSuite getTestSuite(GetTestSuiteRequest testSuiteRequest) throws AuthServiceException {
try {
Request request = new GetTestSuiteRequestMarshaller().marshall(testSuiteRequest);
return invoke(request, TestSuiteJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute get test suite request", ex);
}
}
@Override
public List listTestSuite(ListTestSuiteRequest testSuiteRequest) throws AuthServiceException {
try {
Request request = new ListTestSuiteRequestMarshaller().marshall(testSuiteRequest);
return invoke(request, ListTestSuiteJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list test suite request", ex);
}
}
@Override
public void deleteTestSuite(DeleteTestSuiteRequest testSuiteRequest) throws AuthServiceException {
try {
Request request = new DeleteTestSuiteRequestMarshaller().marshall(testSuiteRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute delete test suite request", ex);
}
}
//~ override test-cycle methods =============================================
@Override
public TestCycle getTestCycle(GetTestCycleRequest testCycleRequest) throws AuthServiceException {
try {
Request request = new GetTestCycleRequestMarshaller().marshall(testCycleRequest);
return invoke(request, TestCycleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute get test cycle request", ex);
}
}
@Override
public List listTestCycle(ListTestCycleRequest testCycleRequest) throws AuthServiceException {
try {
Request request = new ListTestCycleRequestMarshaller().marshall(testCycleRequest);
return invoke(request, ListTestCycleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list test cycle request", ex);
}
}
@Override
public TestCycle createTestCycle(CreateTestCycleRequest testCycleRequest) throws AuthServiceException {
try {
Request request = new CreateTestCycleRequestMarshaller().marshall(testCycleRequest);
return invoke(request,TestCycleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute create test cycle request", ex);
}
}
@Override
public TestCycle updateTestCycle(UpdateTestCycleRequest testCycleRequest) throws AuthServiceException {
try {
Request request = new UpdateTestCycleRequestMarshaller().marshall(testCycleRequest);
return invoke(request, TestCycleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute update test cycle request", ex);
}
}
@Override
public TestCycle moveTestCycle(MoveTestCycleRequest testCycleRequest) throws AuthServiceException {
try {
Request request = new MoveTestCycleRequestMarshaller().marshall(testCycleRequest);
return invoke(request, TestCycleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute move test cycle request", ex);
}
}
@Override
public void deleteTestCycle(DeleteTestCycleRequest testCycleRequest) throws AuthServiceException {
try {
Request request = new DeleteTestCycleRequestMarshaller().marshall(testCycleRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute delete test cycle request", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy