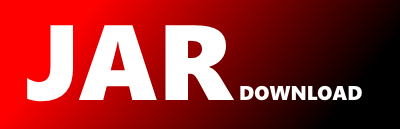
org.qas.qtest.api.services.execution.model.AutomationTestLog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import org.qas.api.DateTimeDeserializer;
import org.qas.api.DateTimeSerializer;
import org.qas.api.internal.util.google.collect.Lists;
import org.qas.qtest.api.internal.model.FieldValue;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import org.qas.qtest.api.services.attachment.model.Attachment;
import org.qas.qtest.api.services.design.model.TestCase;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
/**
* AutomationTestLog
*
* @author Dzung Nguyen
* @version $Id AutomationTestLog 2014-03-28 20:10:30z dungvnguyen $
* @since 1.0
*/
public class AutomationTestLog extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("name")
private String name;
@JsonProperty("status")
private String status;
@JsonProperty("system_name")
private String systemName;
@JsonProperty("test_case_version_id")
private Long testCaseVersionId;
@JsonProperty("exe_start_date")
private Date executionStartDate;
@JsonProperty("exe_end_date")
private Date executionEndDate;
@JsonProperty("user_id")
private Long userId;
@JsonProperty("note")
private String note;
@JsonProperty("automation_content")
private String automationContent;
@JsonProperty("test_case")
private TestCase testCase;
@JsonProperty("test_case_id")
private Long testCaseId;
@JsonProperty("attachments")
private List attachments;
@JsonProperty("test_step_logs")
private List testStepLogs;
@JsonProperty("build_number")
private String buildNumber;
@JsonProperty("build_url")
private String buildUrl;
@JsonProperty("module_names")
private List moduleNames;
@JsonProperty("properties")
private List fieldValues;
@JsonProperty("order")
private Long order;
public AutomationTestLog() {
}
/**
* @return the test log identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the test log identifier.
*
* @param id the given test log identifier to set.
*/
public AutomationTestLog setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the test log identifier.
*
* @param id the given test log identifier to set.
*/
public AutomationTestLog withId(Long id) {
setId(id);
return this;
}
/**
* @return the automation name.
*/
public String getName() {
return name;
}
/**
* Sets the automation name.
*
* @param name the given automation name.
*/
public AutomationTestLog setName(String name) {
this.name = name;
return this;
}
/**
* Sets the automation name.
*
* @param name the given automation name.
*/
public AutomationTestLog withName(String name) {
setName(name);
return this;
}
/**
* @return the test log status.
*/
public String getStatus() {
return status;
}
/**
* Sets the test log status.
*
* @param status the given test log status to set.
*/
public AutomationTestLog setStatus(String status) {
this.status = status;
return this;
}
/**
* Sets the test log status.
*
* @param status the given test log status to set.
*/
public AutomationTestLog withStatus(String status) {
setStatus(status);
return this;
}
/**
* @return the system name.
*/
public String getSystemName() {
return systemName;
}
/**
* Sets the system name.
*
* @param systemName the given system name to set.
*/
public AutomationTestLog setSystemName(String systemName) {
this.systemName = systemName;
return this;
}
/**
* Sets the system name.
*
* @param systemName the given system name to set.
*/
public AutomationTestLog withSystemName(String systemName) {
setSystemName(systemName);
return this;
}
/**
* @return the test case version id.
*/
public Long getTestCaseVersionId() {
return testCaseVersionId;
}
/**
* Sets test case version id.
*
* @param testCaseVersionId the given test case version id to set.
*/
public AutomationTestLog setTestCaseVersionId(Long testCaseVersionId) {
this.testCaseVersionId = testCaseVersionId;
return this;
}
/**
* Sets test case version id.
*
* @param testCaseVersionId the given test case version id to set.
*/
public AutomationTestLog withTestCaseVersionId(Long testCaseVersionId) {
setTestCaseVersionId(testCaseVersionId);
return this;
}
/**
* @return the time when starting running test run.
*/
@JsonSerialize(using = DateTimeSerializer.class, typing = JsonSerialize.Typing.STATIC, include = JsonSerialize.Inclusion.NON_NULL)
public Date getExecutionStartDate() {
return executionStartDate;
}
/**
* Sets the time when starting running test run.
*
* @param executionStartDate the given time when starting running test run to set.
*/
@JsonDeserialize(using = DateTimeDeserializer.class)
public AutomationTestLog setExecutionStartDate(Date executionStartDate) {
this.executionStartDate = executionStartDate;
return this;
}
/**
* Sets the time when starting running test run.
*
* @param executionStartDate the given time when starting running test run to set.
*/
public AutomationTestLog withExecutionStartDate(Date executionStartDate) {
setExecutionStartDate(executionStartDate);
return this;
}
/**
* @return the time when finishing running test run.
*/
@JsonSerialize(using = DateTimeSerializer.class, typing = JsonSerialize.Typing.STATIC, include = JsonSerialize.Inclusion.NON_NULL)
public Date getExecutionEndDate() {
return executionEndDate;
}
/**
* Sets the execution end date.
*
* @param executionEndDate the given execution end date.
*/
@JsonDeserialize(using = DateTimeDeserializer.class)
public AutomationTestLog setExecutionEndDate(Date executionEndDate) {
this.executionEndDate = executionEndDate;
return this;
}
/**
* Sets the execution end date.
*
* @param executionEndDate the given execution end date.
*/
public AutomationTestLog withExecutionEndDate(Date executionEndDate) {
setExecutionEndDate(executionEndDate);
return this;
}
/**
* @return id of user who ran test run.
*/
public Long getUserId() {
return userId;
}
/**
* Sets the id of user who ran test run.
*
* @param userId the given id of user who ran test run.
*/
public AutomationTestLog setUserId(Long userId) {
this.userId = userId;
return this;
}
/**
* Sets the id of user who ran test run.
*
* @param userId the given id of user who ran test run.
*/
public AutomationTestLog withUserId(Long userId) {
setUserId(userId);
return this;
}
/**
* @return the test log note.
*/
public String getNote() {
return note;
}
/**
* Sets the test log note.
*
* @param note the given test log note to set.
*/
public AutomationTestLog setNote(String note) {
this.note = note;
return this;
}
/**
* Sets the test log note.
*
* @param note the given test log note to set.
*/
public AutomationTestLog withNote(String note) {
setNote(note);
return this;
}
/**
* @return the automation content associated to test suite.
*/
public String getAutomationContent() {
return automationContent;
}
/**
* Sets the automation content associated to test suite.
*
* @param automationContent the given automation content to set.
*/
public AutomationTestLog setAutomationContent(String automationContent) {
this.automationContent = automationContent;
return this;
}
/**
* Sets the automation content associated to test suite.
*
* @param automationContent the given automation content to set.
*/
public AutomationTestLog withAutomationContent(String automationContent) {
setAutomationContent(automationContent);
return this;
}
/**
* @return the test case instance.
*/
public TestCase getTestCase() {
return testCase;
}
/**
* Sets the test case instance.
*
* @param testCase the given test case to set.
*/
public AutomationTestLog setTestCase(TestCase testCase) {
this.testCase = testCase;
return this;
}
/**
* Sets the test case instance.
*
* @param testCase the given test case to set.
*/
public AutomationTestLog withTestCase(TestCase testCase) {
setTestCase(testCase);
return this;
}
/**
* @return the test case id.
*/
public Long getTestCaseId() {
return testCaseId;
}
/**
* Sets test case id.
*
* @param testCaseId the given test case id to set.
*/
public AutomationTestLog setTestCaseId(Long testCaseId) {
this.testCaseId = testCaseId;
return this;
}
/**
* Sets test case id.
*
* @param testCaseId the given test case id to set.
*/
public AutomationTestLog withTestCaseId(Long testCaseId) {
setTestCaseId(testCaseId);
return this;
}
/**
* @return the list of testlog's attachments.
*/
public List getAttachments() {
if (null == attachments) {
return Collections.emptyList();
}
return attachments;
}
/**
* @return the list of {@link TestStepLog} objects.
*/
public List getTestStepLogs() {
if (null == testStepLogs) {
return Collections.emptyList();
}
return testStepLogs;
}
/**
* Sets the test step logs.
*
* @param testStepLogs the given list of test step logs to set.
*/
public AutomationTestLog setTestStepLogs(List testStepLogs) {
this.testStepLogs = testStepLogs;
return this;
}
/**
* Sets the test step logs.
*
* @param testStepLogs the given list of test step logs to set.
*/
public AutomationTestLog withTestStepLogs(List testStepLogs) {
setTestStepLogs(testStepLogs);
return this;
}
/**
* Add the test step log.
*
* @param testStepLog the given test step log to add.
* @return current {@link AutomationTestLog automation test log} object.
*/
public AutomationTestLog addTestStepLog(AutomationTestStepLog testStepLog) {
if (testStepLogs == null) {
testStepLogs = Lists.newLinkedList();
}
testStepLogs.add(testStepLog);
return this;
}
/**
* Sets the list of testlog's attachments.
*
* @param attachments the given list of testlog's attachment to set.
*/
public AutomationTestLog setAttachments(List attachments) {
this.attachments = attachments;
return this;
}
/**
* Sets the list of testlog's attachments.
*
* @param attachments the given list of testlog's attachment to set.
*/
public AutomationTestLog withAttachments(List attachments) {
setAttachments(attachments);
return this;
}
/**
* Adds attachment to test log.
*
* @param attachment the given attachment to add to test log.
* @return the {@link TestLog} object.
*/
public AutomationTestLog addAttachment(Attachment attachment) {
if (attachments == null) {
attachments = new ArrayList<>();
}
attachments.add(attachment);
return this;
}
@Override
protected TestLog clone() {
TestLog that = new TestLog();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "automation-test-log";
}
@Override
public String jsonElementName() {
return "automation_test_log";
}
/**
* Get build number
*
* @return build number value
*/
public String getBuildNumber() {
return buildNumber;
}
/**
* Set build number to test log
*
* @param buildNumber build number value
* @return this instance
*/
public AutomationTestLog setBuildNumber(String buildNumber) {
this.buildNumber = buildNumber;
return this;
}
/**
* Set build number to test log
*
* @param buildNumber build number value
* @return this instance
*/
public AutomationTestLog withBuildNumber(String buildNumber) {
return this.setBuildNumber(buildNumber);
}
/**
* Get build url
*
* @return build url
*/
public String getBuildUrl() {
return buildUrl;
}
/**
* Set build url
*
* @param buildUrl build url value
* @return this instance
*/
public AutomationTestLog setBuildUrl(String buildUrl) {
this.buildUrl = buildUrl;
return this;
}
/**
* Set build url
*
* @param buildUrl build url
* @return this instance
*/
public AutomationTestLog withBuildUrl(String buildUrl) {
return setBuildUrl(buildUrl);
}
public List getModuleNames() {
return moduleNames;
}
public void setModuleNames(List moduleNames) {
this.moduleNames = moduleNames;
}
public AutomationTestLog withModuleNames(List moduleNames) {
setModuleNames(moduleNames);
return this;
}
public AutomationTestLog addModuleName(String moduleName) {
if (null == this.moduleNames) {
this.moduleNames = new ArrayList<>();
}
this.moduleNames.add(moduleName);
return this;
}
public List getFieldValues() {
return fieldValues;
}
public void setFieldValues(List fieldValues) {
this.fieldValues = fieldValues;
}
public AutomationTestLog withFieldValues(List fieldValues) {
setFieldValues(fieldValues);
return this;
}
public AutomationTestLog addFieldValue(FieldValue fieldValue) {
if (null == this.fieldValues) {
this.fieldValues = new ArrayList<>();
}
this.fieldValues.add(fieldValue);
return this;
}
public Long getOrder() {
return order;
}
public void setOrder(Long order) {
this.order = order;
}
public AutomationTestLog withOrder(Long order) {
setOrder(order);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy