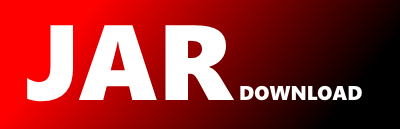
org.qas.qtest.api.services.execution.model.AutomationTestLogRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution.model;
import org.qas.api.ApiServiceRequest;
import java.util.Date;
/**
* AutomationTestLogRequest
*
* @author Dzung Nguyen
* @version $Id AutomationTestLogRequest 2014-03-29 10:17:30z dungvnguyen $
* @since 1.0
*/
public class AutomationTestLogRequest extends ApiServiceRequest {
//~ class properties ========================================================
private Long projectId;
private Long testRunId;
private AutomationTestLog automationTestLog;
private boolean suitePerDay;
private Date suiteDate;
private Boolean forceUpdateVersion;
private Long scheduledBy;
public Boolean getForceUpdateVersion() {
return forceUpdateVersion;
}
public void setForceUpdateVersion(Boolean forceUpdateVersion) {
this.forceUpdateVersion = forceUpdateVersion;
}
//~ class members ===========================================================
/**
* Sets the automation test log.
*
* @param automationTestLog the given automation test log.
*/
public void setAutomationTestLog(AutomationTestLog automationTestLog) {
this.automationTestLog = automationTestLog;
}
/**
* @return the automation test log instance.
*/
public AutomationTestLog getAutomationTestLog() {
return this.automationTestLog;
}
/**
* Sets the automation test log.
*
* @param automationTestLog the given automation test log.
* @return the current {@link org.qas.qtest.api.services.execution.model.AutomationTestLogRequest}
* object.
*/
public AutomationTestLogRequest withAutomationTestLog(AutomationTestLog automationTestLog) {
setAutomationTestLog(automationTestLog);
return this;
}
/**
* @return the project id.
*/
public Long getProjectId() {
return projectId;
}
/**
* Sets the project identifier.
*
* @param projectId the given project id to set.
*/
public void setProjectId(Long projectId) {
this.projectId = projectId;
}
/**
* Sets the project identifier.
*
* @param projectId the given project id to set.
*/
public AutomationTestLogRequest withProjectId(Long projectId) {
setProjectId(projectId);
return this;
}
/**
* @return the test run id.
*/
public Long getTestRunId() {
return testRunId;
}
/**
* Sets the test run id.
*
* @param testRunId the given test run identifier to set.
*/
public void setTestRunId(Long testRunId) {
this.testRunId = testRunId;
}
/**
* Sets the test run id.
*
* @param testRunId the given test run identifier to set.
* @return the {@link AutomationTestLogRequest} object.
*/
public AutomationTestLogRequest withTestRunId(Long testRunId) {
setTestRunId(testRunId);
return this;
}
/**
* @return {@code true} if user want create the suite per day.
*/
public boolean isSuitePerDay() {
return suitePerDay;
}
/**
* Sets the suite-per-day flag.
*
* @param suitePerDay the given suite-per-day flag to set.
*/
public void setSuitePerDay(boolean suitePerDay) {
this.suitePerDay = suitePerDay;
}
/**
* Sets the suite-per-day flag.
*
* @param suitePerDay the given suite-per-day flag to set.
* @return the {@link AutomationTestLogRequest} instance.
*/
public AutomationTestLogRequest withSuitePerDay(boolean suitePerDay) {
setSuitePerDay(suitePerDay);
return this;
}
/**
* @return the given date to create the suite;
*/
public Date getSuiteDate() {
return suiteDate;
}
/**
* Sets the suite date; if the suite-date is not set, we will use the current
* date if the suite-per-day is true.
*
* @param suiteDate the given suite-date.
*/
public void setSuiteDate(Date suiteDate) {
this.suiteDate = suiteDate;
}
/**
* Sets the suite date; if the suite-date is not set, we will use the current
* date if the suite-per-day is true.
*
* @param suiteDate the given suite-date.
* @return the {@link AutomationTestLogRequest automation test log request} instance.
*/
public AutomationTestLogRequest withSuiteDate(Date suiteDate) {
setSuiteDate(suiteDate);
return this;
}
public Long getScheduledBy() {
return scheduledBy;
}
public void setScheduledBy(Long scheduledBy) {
this.scheduledBy = scheduledBy;
}
public AutomationTestLogRequest withScheduledBy(Long scheduledBy) {
setScheduledBy(scheduledBy);
return this;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append(this.getClass().getName())
.append("{").append("\n")
.append("\tprojectId: ").append(projectId).append("\n")
.append("\ttestRunId: ").append(testRunId).append("\n")
.append("\tautomationTestLog:\n").append(automationTestLog).append("\n")
.append("\tsuitePerDay:\n").append(suitePerDay).append("\n")
.append("\tsuiteDate:\n").append(suiteDate).append("\n")
.append("}");
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy