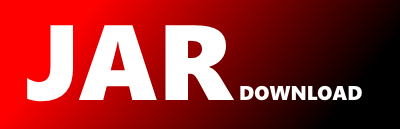
org.qas.qtest.api.services.execution.model.AutomationTestStepLog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution.model;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.api.internal.util.google.collect.Lists;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import org.qas.qtest.api.services.attachment.model.Attachment;
import org.qas.qtest.api.services.defect.model.DefectLink;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* AutomationTestStepLog
*
* @author Dzung Nguyen
* @version $Id AutomationTestStepLog 2015-01-20 10:14:30z dzungvnguyen $
* @since 1.0
*/
public final class AutomationTestStepLog extends QTestBaseModel {
@JsonProperty("description")
private String description;
@JsonProperty("status")
private String status;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonProperty("expected_result")
private String expected;
@JsonProperty("actual_result")
private String actualResult;
@JsonProperty("order")
private Integer order;
@JsonProperty("attachments")
private List attachments;
@JsonProperty("defects")
private List defectLinks;
public AutomationTestStepLog() {
}
/**
* @return the test step description.
*/
public String getDescription() {
return description;
}
/**
* Sets the test step description.
*
* @param description the given test step description to set.
*/
public AutomationTestStepLog setDescription(String description) {
this.description = description;
return this;
}
/**
* Sets the test step description.
*
* @param description the given test step description to set.
*/
public AutomationTestStepLog withDescription(String description) {
setDescription(description);
return this;
}
/**
* @return the test step log status.
*/
public String getStatus() {
return status;
}
/**
* Sets the test step log status.
*
* @param status the given test step log status to set.
*/
public AutomationTestStepLog setStatus(String status) {
this.status = status;
return this;
}
/**
* Sets the test step log status.
*
* @param status the given test step log status to set.
* @return current automation test-step-log.
*/
public AutomationTestStepLog withStatus(String status) {
setStatus(status);
return this;
}
/**
* @return the expected result of an individual test step.
*/
public String getExpected() {
return expected;
}
/**
* Sets the expected result of an individual test step.
*
* @param expected the given expected result to set.
*/
public AutomationTestStepLog setExpected(String expected) {
this.expected = expected;
return this;
}
/**
* Sets the expected result of an individual test step.
*
* @param expected the given expected result to set.
*/
public AutomationTestStepLog withExpected(String expected) {
setExpected(expected);
return this;
}
/**
* @return the test step log actual result.
*/
public String getActualResult() {
return actualResult;
}
/**
* Sets the actual result.
*
* @param actualResult the given actual result to set.
*/
public AutomationTestStepLog setActualResult(String actualResult) {
this.actualResult = actualResult;
return this;
}
/**
* Sets the actual result.
*
* @param actualResult the given actual result to set.
*/
public AutomationTestStepLog withActualResult(String actualResult) {
setActualResult(actualResult);
return this;
}
/**
* @return the order of test step log in test case.
*/
public Integer getOrder() {
return order;
}
/**
* Sets the order of test step log in test case.
*
* @param order the given test step log's order to set.
*/
public AutomationTestStepLog setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the order of test step log in test case.
*
* @param order the given test step log's order to set.
*/
public AutomationTestStepLog withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the list of test step's attachments.
*/
public List getAttachments() {
if (null == attachments) {
return Collections.emptyList();
}
return attachments;
}
/**
* Sets the list of test step's attachments.
*
* @param attachments the given list of test step's attachment to set.
*/
public AutomationTestStepLog setAttachments(List attachments) {
this.attachments = attachments;
return this;
}
/**
* Sets the list of test step's attachments.
*
* @param attachments the given list of test step's attachment to set.
*/
public AutomationTestStepLog withAttachments(List attachments) {
setAttachments(attachments);
return this;
}
/**
* Adds attachment to test step.
*
* @param attachment the given attachment to add to test step.
* @return the {@link AutomationTestStepLog} object.
*/
public AutomationTestStepLog addAttachment(Attachment attachment) {
if (attachments == null) {
attachments = new ArrayList<>();
}
attachments.add(attachment);
return this;
}
/**
* @return the list of defects.
*/
public List getDefects() {
if (defectLinks == null) {
return Collections.emptyList();
}
return defectLinks;
}
/**
* Sets the list of defectLinks.
*
* @param defectLinks the given list of defectLinks to set.
*/
public AutomationTestStepLog setDefects(List defectLinks) {
this.defectLinks = defectLinks;
return this;
}
/**
* Sets the list of defectLinks.
*
* @param defectLinks the given list of defectLinks to set.
*/
public AutomationTestStepLog withDefects(List defectLinks) {
setDefects(defectLinks);
return this;
}
/**
* Add the defectLink to test step log.
*
* @param defectLink the given defectLink to add.
* @return the current test step log instance.
*/
public AutomationTestStepLog addDefect(DefectLink defectLink) {
if (defectLinks == null) {
defectLinks = Lists.newLinkedList();
}
defectLinks.add(defectLink);
return this;
}
@Override
protected AutomationTestStepLog clone() {
AutomationTestStepLog that = new AutomationTestStepLog();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "test-step-log";
}
@Override
public String jsonElementName() {
return "test_step_log";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy