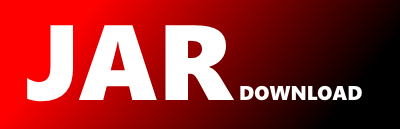
org.qas.qtest.api.services.execution.model.TestCycle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.List;
/**
* TestCycle
*
* @author Dzung Nguyen
* @version $Id TestCycle 2015-05-26 14:05:30z dzungvnguyen $
* @since 1.0
*/
public class TestCycle extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("name")
private String name;
@JsonProperty("pid")
private String pid;
@JsonProperty("description")
private String description;
@JsonProperty("order")
private Integer order;
@JsonProperty("target_build_id")
private Long targetBuildId;
@JsonProperty("test-cycles")
private List testCycles;
@JsonProperty("test-suites")
private List testSuites;
public TestCycle() {
}
/**
* @return the test cycle identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the test cycle identifier.
*
* @param id the given test cycle id to set.
*/
public TestCycle setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the test cycle identifier.
*
* @param id the given test cycle id to set.
*/
public TestCycle withId(Long id) {
setId(id);
return this;
}
/**
* @return the test cycle project based identifier.
*/
public String getPid() {
return pid;
}
/**
* Sets the test cycle project based identifier.
*
* @param pid the given test cycle project based id to set.
*/
public TestCycle setPid(String pid) {
this.pid = pid;
return this;
}
/**
* Sets the test cycle project based identifier.
*
* @param pid the given test cycle project based id to set.
*/
public TestCycle withId(String pid) {
setPid(pid);
return this;
}
/**
* @return the test cycle name.
*/
public String getName() {
return name;
}
/**
* Sets test cycle name.
*
* @param name the given test cycle name to set.
*/
public TestCycle setName(String name) {
this.name = name;
return this;
}
/**
* Sets test cycle name.
*
* @param name the given test cycle name to set.
*/
public TestCycle withName(String name) {
setName(name);
return this;
}
/**
* @return the test cycle description.
*/
public String getDescription() {
return description;
}
/**
* Sets test cycle description.
*
* @param description the given test cycle description to set.
*/
public TestCycle setDescription(String description) {
this.description = description;
return this;
}
/**
* Sets test cycle description.
*
* @param description the given test cycle description to set.
*/
public TestCycle withDescription(String description) {
setDescription(description);
return this;
}
/**
* @return the test cycle order.
*/
public Integer getOrder() {
return order;
}
/**
* Sets the test cycle order.
*
* @param order the given test cycle order to set.
*/
public TestCycle setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the test cycle order and return current test cycle.
*
* @param order the given test cycle order to set.
* @return the current test cycle instance.
*/
public TestCycle withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the target build identifier.
*/
public Long getTargetBuildId() {
return targetBuildId;
}
/**
* Sets the target build identifier.
*
* @param targetBuildId the given target build identifier to set.
*/
public TestCycle setTargetBuildId(Long targetBuildId) {
this.targetBuildId = targetBuildId;
return this;
}
/**
* Sets the target build identifier.
*
* @param targetBuildId the given target build identifier.
* @return the current test cycle instance.
*/
public TestCycle withTargetBuildId(Long targetBuildId) {
setTargetBuildId(targetBuildId);
return this;
}
/**
* @return the list of test-cycles in this cycle.
*/
public List getTestCycles() {
return testCycles;
}
/**
* Sets the list of test-cycles to current cycles.
*
* @param testCycles the given list of test-cycles to set.
*/
public TestCycle setTestCycles(List testCycles) {
this.testCycles = testCycles;
return this;
}
/**
* Sets the list of test-cycles to current cycles.
*
* @param testCycles the given list of test-cycles to set.
* @return the current test-cycles.
*/
public TestCycle withTestCycles(List testCycles) {
setTestCycles(testCycles);
return this;
}
/**
* @return the list of test-cycles in this cycle.
*/
public List getTestSuites() {
return testSuites;
}
/**
* Sets the list of test-suites to current cycles.
*
* @param testSuites the given list of test-suites to set.
*/
public TestCycle setTestSuites(List testSuites) {
this.testSuites = testSuites;
return this;
}
/**
* Sets the list of test-suites to current cycles.
*
* @param testSuites the given list of test-suites to set.
* @return the current test-cycle.
*/
public TestCycle withTestSuites(List testSuites) {
setTestSuites(testSuites);
return this;
}
@Override
protected TestCycle clone() {
TestCycle that = new TestCycle();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "test-cycle";
}
@Override
public String jsonElementName() {
return "test_cycle";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy