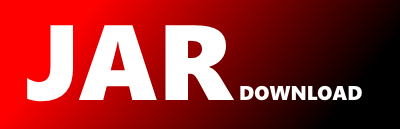
org.qas.qtest.api.services.execution.model.TestStepLog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.execution.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.api.internal.util.google.collect.Lists;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import org.qas.qtest.api.services.defect.model.DefectLink;
import org.qas.qtest.api.services.design.model.TestStep;
import java.util.Collections;
import java.util.List;
/**
* TestStepLog
*
* @author Dzung Nguyen
* @version $Id TestStepLog 2014-03-28 07:11:30z dungvnguyen $
* @since 1.0
*/
public class TestStepLog extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("test_step_id")
private Long testStepId;
@JsonProperty("status")
private ExecutionStatus status;
@JsonProperty("actual_result")
private String actualResult;
@JsonProperty("order")
private Integer order;
@JsonProperty("group")
private Integer group;
@JsonProperty("called_test_case_name")
private String calledTestCaseName;
@JsonProperty("called_test_case_id")
private Long calledTestCaseId;
@JsonProperty("test_step")
private TestStep testStep;
@JsonProperty("defects")
private List defectLinks;
@JsonProperty("parent_test_step_id")
private Long parentTestStepId;
public TestStepLog() {
}
/**
* @return the test step log identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the test step log id.
*
* @param id the given test step log id to set.
*/
public TestStepLog setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the test step log id.
*
* @param id the given test step log id to set.
*/
public TestStepLog withId(Long id) {
setId(id);
return this;
}
/**
* @return the test step id.
*/
public Long getTestStepId() {
return testStepId;
}
/**
* Sets the test step id.
*
* @param testStepId the given test step id to set.
*/
public TestStepLog setTestStepId(Long testStepId) {
this.testStepId = testStepId;
return this;
}
/**
* Sets the test step id.
*
* @param testStepId the given test step id to set.
*/
public TestStepLog withTestStepId(Long testStepId) {
setTestStepId(testStepId);
return this;
}
/**
* @return the test step log status.
*/
public ExecutionStatus getStatus() {
return status;
}
/**
* Sets the test step log status.
*
* @param status the given test step log status to set.
*/
public TestStepLog setStatus(ExecutionStatus status) {
this.status = status;
return this;
}
/**
* Sets the test step log status.
*
* @param status the given test step log status to set.
*/
public TestStepLog withStatus(ExecutionStatus status) {
setStatus(status);
return this;
}
/**
* @return the test step log actual result.
*/
public String getActualResult() {
return actualResult;
}
/**
* Sets the actual result.
*
* @param actualResult the given actual result to set.
*/
public TestStepLog setActualResult(String actualResult) {
this.actualResult = actualResult;
return this;
}
/**
* Sets the actual result.
*
* @param actualResult the given actual result to set.
*/
public TestStepLog withActualResult(String actualResult) {
setActualResult(actualResult);
return this;
}
/**
* @return the order of test step log in test case.
*/
public Integer getOrder() {
return order;
}
/**
* Sets the order of test step log in test case.
*
* @param order the given test step log's order to set.
*/
public TestStepLog setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the order of test step log in test case.
*
* @param order the given test step log's order to set.
*/
public TestStepLog withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the test step log group.
*/
public Integer getGroup() {
return group;
}
/**
* Sets the test step log group.
*
* @param group the given test step log group.
*/
public TestStepLog setGroup(Integer group) {
this.group = group;
return this;
}
/**
* Sets the test step log group.
*
* @param group the given test step log group.
*/
public TestStepLog withGroup(Integer group) {
setGroup(group);
return this;
}
/**
* @return the name of called test case if this step is a called test step log.
*/
public String getCalledTestCaseName() {
return calledTestCaseName;
}
/**
* Sets called test case name.
*
* @param calledTestCaseName the given called test case name to set.
*/
public TestStepLog setCalledTestCaseName(String calledTestCaseName) {
this.calledTestCaseName = calledTestCaseName;
return this;
}
/**
* Sets called test case name.
*
* @param calledTestCaseName the given called test case name to set.
*/
public TestStepLog withCalledTestCaseName(String calledTestCaseName) {
setCalledTestCaseName(calledTestCaseName);
return this;
}
/**
* @return the called test case id.
*/
public Long getCalledTestCaseId() {
return calledTestCaseId;
}
/**
* Sets the called test case id.
*
* @param calledTestCaseId the given called test case id to set.
*/
public TestStepLog setCalledTestCaseId(Long calledTestCaseId) {
this.calledTestCaseId = calledTestCaseId;
return this;
}
/**
* Sets the called test case id.
*
* @param calledTestCaseId the given called test case id to set.
*/
public TestStepLog withCalledTestCaseId(Long calledTestCaseId) {
setCalledTestCaseId(calledTestCaseId);
return this;
}
/**
* @return the {@link TestStep} instance.
*/
public TestStep getTestStep() {
return testStep;
}
/**
* Sets test step instance.
*
* @param testStep the given test step instance to set.
*/
public TestStepLog setTestStep(TestStep testStep) {
this.testStep = testStep;
return this;
}
/**
* Sets test step instance.
*
* @param testStep the given test step instance to set.
*/
public TestStepLog withTestStep(TestStep testStep) {
setTestStep(testStep);
return this;
}
/**
* @return the list of defects.
*/
public List getDefects() {
if (null == defectLinks) {
return Collections.emptyList();
}
return defectLinks;
}
/**
* Sets the list of defectLinks.
*
* @param defectLinks the given list of defectLinks to set.
*/
public TestStepLog setDefects(List defectLinks) {
this.defectLinks = defectLinks;
return this;
}
/**
* Sets the list of defectLinks.
*
* @param defectLinks the given list of defectLinks to set.
*/
public TestStepLog withDefects(List defectLinks) {
setDefects(defectLinks);
return this;
}
/**
* Add the defectLink to test step log.
*
* @param defectLink the given defectLink to add.
* @return the current test step log instance.
*/
public TestStepLog addDefect(DefectLink defectLink) {
if (defectLinks == null) {
defectLinks = Lists.newLinkedList();
}
defectLinks.add(defectLink);
return this;
}
public Long getParentTestStepId() {
return parentTestStepId;
}
public TestStepLog setParentTestStepId(Long parentTestStepId) {
this.parentTestStepId = parentTestStepId;
return this;
}
public TestStepLog withParentTestStepId(Long parentTestStepId) {
this.parentTestStepId = parentTestStepId;
return this;
}
@Override
protected TestStepLog clone() {
TestStepLog that = new TestStepLog();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "test-step-log";
}
@Override
public String jsonElementName() {
return "test_step_log";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy