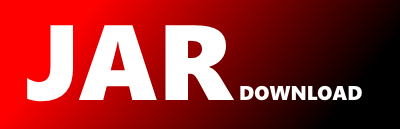
org.qas.qtest.api.services.host.HostServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.host;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.Request;
import org.qas.api.internal.util.google.base.Throwables;
import org.qas.api.transform.VoidJsonUnmarshaller;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.internal.QTestApiWebServiceClient;
import org.qas.qtest.api.services.host.model.*;
import org.qas.qtest.api.services.host.model.transform.*;
import java.util.List;
/**
* HostServiceClient
*
* @author Thong Nguyen
* @version $Id HostServiceClient 2015-03-14 19:09:30z thongnguyen $
* @since 1.0
*/
public class HostServiceClient extends QTestApiWebServiceClient implements HostService {
/**
* Constructs a new client to invoke service method on HostServiceClient using
* the default qTest credentials provider and default client configuration options.
*/
public HostServiceClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on HostServiceClient using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestDesignService
*/
public HostServiceClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service method on HostServiceClient using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public HostServiceClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on HostServiceClient using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestDesignService
*/
public HostServiceClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = new StaticQTestCredentialsProvider(credentials);
init();
}
/**
* Constructs a new client to invoke service method on HostServiceClient using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
*/
public HostServiceClient(QTestCredentialsProvider credentialsProvider) {
this(credentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on HostServiceClient using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestDesignService
*/
public HostServiceClient(QTestCredentialsProvider credentialsProvider, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = credentialsProvider;
init();
}
@Override
public AutomationHost registerAutomationHost(CreateAutomationHostRequest automationHostRequest)
throws AuthServiceException {
try {
Request request = new CreateAutomationHostRequestMarshaller().marshall(automationHostRequest);
return invoke(request, AutomationHostJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during create automation host request", ex);
}
}
@Override
public AutomationHost updateAutomationHost(UpdateAutomationHostRequest automationHostRequest)
throws AuthServiceException {
try {
Request request = new UpdateAutomationHostRequestMarshaller().marshall(automationHostRequest);
return invoke(request, AutomationHostJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during create automation host request", ex);
}
}
@Override
public AutomationAgent registerAutomationAgent(CreateAutomationAgentRequest automationAgentRequest)
throws AuthClientException {
try {
Request request = new CreateAutomationAgentRequestMarshaller().marshall(automationAgentRequest);
return invoke(request, AutomationAgentJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during register automation agent request", ex);
}
}
@Override
public AutomationAgent updateAutomationAgent(UpdateAutomationAgentRequest automationAgentRequest)
throws AuthClientException {
try {
Request request = new UpdateAutomationAgentRequestMarshaller().marshall(automationAgentRequest);
return invoke(request, AutomationAgentJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during update automation agent request", ex);
}
}
@Override
public void deleteAutomationAgent(DeleteAutomationAgentRequest deleteAutomationAgentRequest)
throws AuthClientException {
try {
Request request = new DeleteAutomationAgentRequestMarshaller().marshall(deleteAutomationAgentRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during delete automation agent request", ex);
}
}
@Override
public void activateAutomationAgent(ActivateAutomationAgentRequest activateAgentRequest) throws AuthClientException {
try {
Request request = new ActivateAutomationAgentRequestMarshaller().marshall(activateAgentRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during activating agent.", ex);
}
}
@Override
public List listJobs(ListJobRequest jobRequest) throws AuthClientException {
try {
Request request = new ListJobRequestMarshaller().marshall(jobRequest);
return invoke(request, ListJobJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute create module request", ex);
}
}
@Override
public PongMessage pingHost(PingAutomationHostRequest pingRequest) throws AuthClientException {
try {
Request request = new PingAutomationHostRequestMarshaller().marshall(pingRequest);
return invoke(request, PongMessageJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during ping automation host request", ex);
}
}
@Override
public void updateJobStatus(UpdateJobStatusRequest updateJobStatusRequest) throws AuthServiceException {
try {
Request request = new UpdateJobStatusRequestMarshaller().marshall(updateJobStatusRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute update job status request.", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy