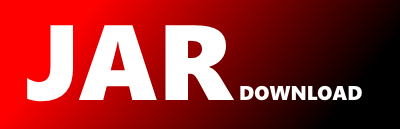
org.qas.qtest.api.services.host.model.AutomationHost Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.host.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
/**
* AutomationHost
*
* @author Thong Nguyen
* @version $Id AutomationHost 2015-03-14 21:48:30z thongnguyen $
* @since 1.0
*/
public class AutomationHost extends QTestBaseModel {
@JsonProperty("host_guid")
private String hostGuid;
@JsonProperty("host_name")
private String hostName;
@JsonProperty("ip_address")
private String ipAddress;
@JsonProperty("mac_address")
private String macAddress;
@JsonProperty("status")
private Boolean status;
@JsonProperty("status_expired_time")
private Long statusExpiredTime;
@JsonProperty("host_server_id")
private Long hostServerId;
@JsonProperty("os")
private String os;
public AutomationHost() {
}
/**
* @return the client host identifier.
*/
public String getHostGuid() {
return hostGuid;
}
/**
* Sets the client host identifier.
*
* @param hostGuid the given client host identifier to set.
* @return current instance.
*/
public AutomationHost setHostGuid(String hostGuid) {
this.hostGuid = hostGuid;
return this;
}
/**
* Sets the client host identifier.
*
* @param hostGuid the given client host identifier to set.
* @return current instance.
*/
public AutomationHost withHostGuid(String hostGuid) {
setHostGuid(hostGuid);
return this;
}
/**
* @return the automation host name.
*/
public String getHostName() {
return hostName;
}
/**
* Sets the host name.
*
* @param hostName the host name to set.
* @return current instance.
*/
public AutomationHost setHostName(String hostName) {
this.hostName = hostName;
return this;
}
/**
* Sets the host name.
*
* @param hostname the given host name to set.
* @return the current instance.
*/
public AutomationHost withHostName(String hostname) {
setHostName(hostname);
return this;
}
/**
* @return the host IP address.
*/
public String getIpAddress() {
return ipAddress;
}
/**
* Sets the IP address.
*
* @param ipAddress the given IP address to set.
* @return current instance.
*/
public AutomationHost setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* Sets the IP address.
*
* @param ipAddress the given IP address to set.
* @return the current instance.
*/
public AutomationHost withIpAddress(String ipAddress) {
setIpAddress(ipAddress);
return this;
}
/**
* @return the host MAC address.
*/
public String getMacAddress() {
return macAddress;
}
/**
* Sets the host MAC address.
*
* @param macAddress the host MAC address to set.
* @return current instance.
*/
public AutomationHost setMacAddress(String macAddress) {
this.macAddress = macAddress;
return this;
}
/**
* Sets the host MAC address.
*
* @param macAddress the host MAC address to set.
* @return the current instance.
*/
public AutomationHost withMacAddress(String macAddress) {
setMacAddress(macAddress);
return this;
}
/**
* @return the host status.
*/
public Boolean getStatus() {
return status;
}
/**
* Sét the host status.
*
* @param status the host status.
* @return current instance.
*/
public AutomationHost setStatus(Boolean status) {
this.status = status;
return this;
}
/**
* Sets the host status.
*
* @param status the host status to set.
* @return the current instance.
*/
public AutomationHost withStatus(Boolean status) {
setStatus(status);
return this;
}
/**
* @return the status expired time.
*/
public Long getStatusExpiredTime() {
return statusExpiredTime;
}
/**
* Sets the status expired time.
*
* @param statusExpiredTime the status expired time to set.
* @return current instance.
*/
public AutomationHost setStatusExpiredTime(Long statusExpiredTime) {
this.statusExpiredTime = statusExpiredTime;
return this;
}
/**
* Sets the status expired time.
*
* @param statusExpiredTime the status expired time to set.
* @return the current instance.
*/
public AutomationHost withStatusExpiredTime(Long statusExpiredTime) {
setStatusExpiredTime(statusExpiredTime);
return this;
}
/**
* @return the server host identifier.
*/
public Long getHostServerId() {
return hostServerId;
}
/**
* Sets the qtest server host identifier.
*
* @param hostServerId the given server host identifier.
* @return current instance.
*/
public AutomationHost setHostServerId(Long hostServerId) {
this.hostServerId = hostServerId;
return this;
}
/**
* Sets the host server identifier.
*
* @param hostServerId the given host server identifier to set.
* @return the current instance.
*/
public AutomationHost withHostServerId(Long hostServerId) {
setHostServerId(hostServerId);
return this;
}
public String getOs() {
return os;
}
public void setOs(String os) {
this.os = os;
}
public AutomationHost withOs(String os) {
setOs(os);
return this;
}
@Override
public String elementName() {
return "host";
}
@Override
public String jsonElementName() {
return "host";
}
@Override
protected AutomationHost clone() {
AutomationHost copy = new AutomationHost();
copy.setPropertiesFrom(this);
return copy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy