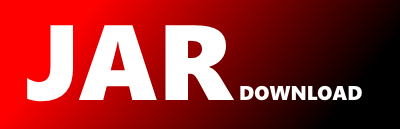
org.qas.qtest.api.services.host.model.AutomationMaterial Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.host.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
/**
* AutomationMaterial
*
* @author chuongle
* @version $Id AutomationMaterial Mar 17, 2015 6:32:51 PM chuongle $
* @since 1.0
*/
public class AutomationMaterial extends QTestBaseModel {
@JsonProperty("test_run_id")
private Long testRunId;
@JsonProperty("test_case_id")
private Long testCaseId;
@JsonProperty("test_case_name")
private String testCaseName;
@JsonProperty("test_case_version_id")
private Long testCaseVersionId;
@JsonProperty("automation_content")
private String automationContent;
public AutomationMaterial() {
}
/**
* @return test run identifier
*/
public Long getTestRunId() {
return testRunId;
}
/**
* set test run identifier
*
* @param id test run identifier
* @return current instance.
*/
public AutomationMaterial setTestRunId(Long id) {
this.testRunId = id;
return this;
}
/**
* set test run identifier
*
* @param id test run identifier
* @return current {@link AutomationMaterial} instance
*/
public AutomationMaterial withTestRunId(Long id) {
setTestRunId(id);
return this;
}
/**
* @return test case identifier
*/
public Long getTestCaseId() {
return testCaseId;
}
/**
* set test case identifier
*
* @param id test case identifier
* @return current instance.
*/
public AutomationMaterial setTestCaseId(Long id) {
this.testCaseId = id;
return this;
}
/**
* set test case identifier
*
* @param id test case identifier
* @return current {@link AutomationMaterial} instance
*/
public AutomationMaterial withTestCaseId(Long id) {
setTestRunId(id);
return this;
}
/**
* @return test case name
*/
public String getTestCaseName() {
return testCaseName;
}
/**
* set test case name
*
* @param name test case name
* @return current instance.
*/
public AutomationMaterial setTestCaseName(String name) {
this.testCaseName = name;
return this;
}
/**
* set test case name
*
* @param name test case name
* @return current {@link AutomationMaterial} instance
*/
public AutomationMaterial withTestCaseName(String name) {
setTestCaseName(name);
return this;
}
/**
* @return test case version id
*/
public Long getTestCaseVersionId() {
return testCaseVersionId;
}
/**
* set test case version id
*
* @param version test case version id
* @return current instance.
*/
public AutomationMaterial setTestCaseVersionId(Long version) {
this.testCaseVersionId = version;
return this;
}
/**
* set test case version id
*
* @param version test case version id
* @return current {@link AutomationMaterial} instance
*/
public AutomationMaterial withTestCaseVersionId(Long version) {
setTestCaseVersionId(version);
return this;
}
/**
* @return automation content
*/
public String getAutomationContent() {
return automationContent;
}
/**
* set automation content
*
* @param content automation content
* @return current instance.
*/
public AutomationMaterial setAutomationContent(String content) {
this.automationContent = content;
return this;
}
/**
* set automation content
*
* @param content automation content
* @return current {@link AutomationMaterial} instance
*/
public AutomationMaterial withAutomationContent(String content) {
setAutomationContent(content);
return this;
}
@Override
public String elementName() {
return "automation-material";
}
@Override
public String jsonElementName() {
return "automation_material";
}
@Override
protected AutomationMaterial clone() {
AutomationMaterial copy = new AutomationMaterial();
copy.setPropertiesFrom(this);
return copy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy