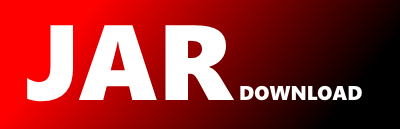
org.qas.qtest.api.services.host.model.Job Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.host.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import org.qas.api.DateTimeDeserializer;
import org.qas.api.DateTimeSerializer;
import org.qas.api.internal.util.google.collect.Lists;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.Collections;
import java.util.Date;
import java.util.List;
/**
* Job
*
* @author chuongle
* @version $Id Job 2015-03-17 17:58:30z chuongle $
* @since 1.0
*/
public class Job extends QTestBaseModel {
@JsonProperty("start_date")
private Date startDate;
@JsonProperty("end_date")
private Date endDate;
@JsonProperty("status")
private Status status;
@JsonProperty("agent_server_id")
private Long agentServerId;
@JsonProperty("agent_id")
private Long agentId;
@JsonProperty("schedule_id")
private Long scheduleId;
@JsonProperty("scheduled_by")
private Long scheduledBy;
@JsonProperty("automation_materials")
private List automationMaterials;
@JsonProperty("job_id")
private Long jobId;
@JsonProperty("host_server_id")
private Long hostServerId;
@JsonProperty("project_id")
private Long projectId;
@JsonProperty("parameters")
private JobParameter parameters;
public Job() {
}
/**
* @return the start date.
*/
@JsonSerialize(using = DateTimeSerializer.class, typing = JsonSerialize.Typing.STATIC, include = JsonSerialize.Inclusion.NON_NULL)
public Date getStartDate() {
return startDate;
}
/**
* Sets the start date.
*
* @param startDate the given start date to set.
* @return current instance.
*/
@JsonDeserialize(using = DateTimeDeserializer.class)
public Job setStartDate(Date startDate) {
this.startDate = startDate;
return this;
}
/**
* Sets the start date.
*
* @param startDate the given start date to set.
* @return the current job execution instance.
*/
public Job withStartDate(Date startDate) {
setStartDate(startDate);
return this;
}
/**
* @return the end date.
*/
@JsonSerialize(using = DateTimeSerializer.class, typing = JsonSerialize.Typing.STATIC, include = JsonSerialize.Inclusion.NON_NULL)
public Date getEndDate() {
return endDate;
}
/**
* Sets the job execution end date.
*
* @param endDate the given execution end date to set.
* @return current instance.
*/
@JsonDeserialize(using = DateTimeDeserializer.class)
public Job setEndDate(Date endDate) {
this.endDate = endDate;
return this;
}
/**
* Sets the job execution end date.
*
* @param endDate the given execution end date to set.
* @return the job execution instance.
*/
public Job withEndDate(Date endDate) {
setEndDate(endDate);
return this;
}
/**
* @return the job execution status.
*/
public Status getStatus() {
return status;
}
/**
* Sets the job execution status.
*
* @param status the given job execution status to set.
* @return current instance.
*/
public Job setStatus(Status status) {
this.status = status;
return this;
}
/**
* Sets the job execution status.
*
* @param status the given job execution status to set.
* @return the given job execution instance.
*/
public Job withStatus(Status status) {
setStatus(status);
return this;
}
/**
* @return the agent server identifier.
*/
public Long getAgentServerId() {
return agentServerId;
}
/**
* Sets the agent server identifier.
*
* @param id the given agent server identifier to set.
* @return current instance.
*/
public Job setAgentServerId(Long id) {
this.agentServerId = id;
return this;
}
/**
* Sets the agent server identifier.
*
* @param id the given agent server identifier to set.
* @return the job execution instance.
*/
public Job withAgentServerId(Long id) {
setAgentServerId(id);
return this;
}
/**
* @return the client agent identifier.
*/
public Long getAgentId() {
return agentId;
}
/**
* Sets the client agent identifier.
*
* @param id the given client agent identifier.
* @return current instance.
*/
public Job setAgentId(Long id) {
this.agentId = id;
return this;
}
/**
* Sets the client agent identifier.
*
* @param id the given client agent identifier.
* @return the job execution instance.
*/
public Job withAgentId(Long id) {
setAgentId(id);
return this;
}
/**
* @return the schedule identifier.
*/
public Long getScheduleId() {
return scheduleId;
}
/**
* Sets the schedule identifier.
*
* @param id the given schedule identifier.
* @return current instance.
*/
public Job setScheduleId(Long id) {
this.scheduleId = id;
return this;
}
/**
* Sets the schedule identifier.
*
* @param id the given schedule identifier.
* @return the job execution instance.
*/
public Job withScheduleId(Long id) {
setScheduleId(id);
return this;
}
/**
* @return the list of {@link AutomationMaterial automation material} objects.
*/
public List getAutomationMaterials() {
if (automationMaterials == null) {
return Collections.emptyList();
}
return automationMaterials;
}
/**
* Sets the automation test runs.
*
* @param automationMaterials the given list of automation material objects to set.
* @return current instance.
*/
public Job setAutomationMaterials(List automationMaterials) {
this.automationMaterials = automationMaterials;
return this;
}
/**
* Sets the automation test runs.
*
* @param automationMaterials the given list of automation material objects to set.
* @return the job execution instance.
*/
public Job withAutomationMaterials(List automationMaterials) {
setAutomationMaterials(automationMaterials);
return this;
}
/**
* Add the automation material to job execution.
*
* @param automationMaterial the given automation material instance to set.
* @return job execution instance.
*/
public Job addAutomationMaterial(AutomationMaterial automationMaterial) {
if (automationMaterials == null) {
automationMaterials = Lists.newLinkedList();
}
automationMaterials.add(automationMaterial);
return this;
}
/**
* @return the job identifier.
*/
public Long getJobId() {
return jobId;
}
/**
* Sets the job identifier.
*
* @param jobId the given job identifier to set.
* @return current instance.
*/
public Job setJobId(Long jobId) {
this.jobId = jobId;
return this;
}
/**
* Sets the job identifier.
*
* @param jobId the given job identifier to set.
* @return current job execution instance.
*/
public Job withJobId(Long jobId) {
setJobId(jobId);
return this;
}
/**
* @return the host server identifier.
*/
public Long getHostServerId() {
return hostServerId;
}
/**
* Sets the host server identifier.
*
* @param hostServerId the given host server identifier.
* @return current instance.
*/
public Job setHostServerId(Long hostServerId) {
this.hostServerId = hostServerId;
return this;
}
/**
* Sets the host server identifier.
*
* @param hostServerId the given host server identifier.
* @return current instance
*/
public Job withHostServerId(Long hostServerId) {
setHostServerId(hostServerId);
return this;
}
/**
* @return the project identifier.
*/
public Long getProjectId() {
return projectId;
}
/**
* Sets the project identifier.
*
* @param projectId the given project identifier to set.
* @return current instance.
*/
public Job setProjectId(Long projectId) {
this.projectId = projectId;
return this;
}
public Long getScheduledBy() {
return scheduledBy;
}
public Job setScheduledBy(Long scheduledBy) {
this.scheduledBy = scheduledBy;
return this;
}
/**
* Sets the project identifier.
*
* @param projectId the given project identifier to set.
* @return current instance
*/
public Job withProjectId(Long projectId) {
setProjectId(projectId);
return this;
}
public JobParameter getParameters() {
return parameters;
}
public void setParameters(JobParameter parameters) {
this.parameters = parameters;
}
@Override
protected Job clone() {
Job copy = new Job();
copy.setPropertiesFrom(this);
return copy;
}
@Override
public String elementName() {
return "job";
}
@Override
public String jsonElementName() {
return "job";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy