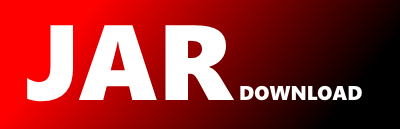
org.qas.qtest.api.services.host.model.PingAutomationHostRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.host.model;
import org.qas.api.ApiServiceRequest;
import java.util.Date;
/**
* AutomationHost
*
* @author Thong Nguyen
* @version $Id AutomationHost 2015-03-19 10:35:30z thongnguyen $
* @since 1.0
*/
public class PingAutomationHostRequest extends ApiServiceRequest {
//~ class properties ========================================================
private Long hostServerId;
private String hostGuid;
private Date pingTime;
private Long pollingFrequency;
private boolean override;
private String hostVersion;
private String os;
private String hostConfig;
private int portConfig;
//~ class members ===========================================================
/**
* @return the host server identifier.
*/
public Long getHostServerId() {
return hostServerId;
}
/**
* Sets the host server identifier.
*
* @param serverId the given host server identifier to set.
*/
public void setHostServerId(Long serverId) {
this.hostServerId = serverId;
}
/**
* Sets the server identifier.
*
* @param serverId the given server identifier to set.
* @return the ping host request instance.
*/
public PingAutomationHostRequest withHostServerId(Long serverId) {
setHostServerId(serverId);
return this;
}
/**
* @return the ping time.
*/
public Date getPingTime() {
return pingTime;
}
/**
* Sets the ping time.
*
* @param pingTime the given ping time to set.
*/
public void setPingTime(Date pingTime) {
this.pingTime = pingTime;
}
/**
* Sets the ping time.
*
* @param pingTime the given ping time to set.
* @return the ping host request instance.
*/
public PingAutomationHostRequest withPingTime(Date pingTime) {
setPingTime(pingTime);
return this;
}
public Long getPollingFrequency() {
return pollingFrequency;
}
public void setPollingFrequency(Long pollingFrequency) {
this.pollingFrequency = pollingFrequency;
}
public PingAutomationHostRequest withPollingFrequency(Long pollingFrequency) {
setPollingFrequency(pollingFrequency);
return this;
}
/**
* @return the host guid.
*/
public String getHostGuid() {
return hostGuid;
}
/**
* Sets the host GUID value.
*
* @param hostGuid the given host GUID value to set.
*/
public void setHostGuid(String hostGuid) {
this.hostGuid = hostGuid;
}
/**
* Sets the host GUID value.
*
* @param hostGuid the given host GUID value to set.
* @return the host GUID request instance.
*/
public PingAutomationHostRequest withHostGuid(String hostGuid) {
setHostGuid(hostGuid);
return this;
}
public boolean isOverride() {
return override;
}
public void setOverride(boolean override) {
this.override = override;
}
public PingAutomationHostRequest withOverride(boolean override) {
setOverride(override);
return this;
}
public String getHostVersion() {
return hostVersion;
}
public void setHostVersion(String hostVersion) {
this.hostVersion = hostVersion;
}
public PingAutomationHostRequest withHostVersion(String hostVersion) {
setHostVersion(hostVersion);
return this;
}
public String getOs() {
return os;
}
public void setOs(String os) {
this.os = os;
}
public PingAutomationHostRequest withOs(String os) {
setOs(os);
return this;
}
public String getHostConfig() {
return hostConfig;
}
public void setHostConfig(String hostConfig) {
this.hostConfig = hostConfig;
}
public PingAutomationHostRequest withHostConfig(String hostConfig) {
setHostConfig(hostConfig);
return this;
}
public int getPortConfig() {
return portConfig;
}
public void setPortConfig(int portConfig) {
this.portConfig = portConfig;
}
public PingAutomationHostRequest withPortConfig(int portConfig) {
setPortConfig(portConfig);
return this;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("PingAutomationHostRequest{");
sb.append("hostServerId=").append(hostServerId);
sb.append(", hostGuid='").append(hostGuid).append('\'');
sb.append(", pingTime=").append(pingTime);
sb.append(", pollingFrequency=").append(pollingFrequency);
sb.append(", override=").append(override);
sb.append(", hostVersion=").append(hostVersion);
sb.append(", os=").append(os);
sb.append(", hostConfig=").append(hostConfig);
sb.append(", portConfig=").append(portConfig);
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy