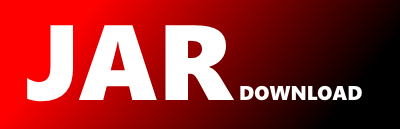
org.qas.qtest.api.services.host.model.PongMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.host.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.Date;
/**
* AutomationHost
*
* @author Thong Nguyen
* @version $Id AutomationHost 2015-03-14 21:48:30z thongnguyen $
* @since 1.0
*/
public class PongMessage extends QTestBaseModel {
@JsonProperty("pong_message")
private String pongMessage;
@JsonProperty("received_time")
private Date receivedTime;
@JsonProperty("polling_frequency")
private Long pollingFrequency;
@JsonProperty("agents_configuration")
private String agentsConfigurationJson;
public PongMessage() {
}
/**
* @return the pong message.
*/
public String getPongMessage() {
return pongMessage;
}
/**
* Sets the pong message.
*
* @param pongMessage the given pong message.
* @return current instance.
*/
public PongMessage setPongMessage(String pongMessage) {
this.pongMessage = pongMessage;
return this;
}
/**
* Sets the pong message.
*
* @param pongMessage the given poing message.
* @return the current instance.
*/
public PongMessage withPongMessage(String pongMessage) {
setPongMessage(pongMessage);
return this;
}
/**
* @return the received time.
*/
public Date getReceivedTime() {
return receivedTime;
}
/**
* Sets the received time.
*
* @param receivedTime the given received time to set.
* @return current instance.
*/
public PongMessage setReceivedTime(Date receivedTime) {
this.receivedTime = receivedTime;
return this;
}
/**
* Sets the received time.
*
* @param receivedTime the given received time to set.
* @return the current instance.
*/
public PongMessage withReceivedTime(Date receivedTime) {
setReceivedTime(receivedTime);
return this;
}
public Long getPollingFrequency() {
return pollingFrequency;
}
public PongMessage setPollingFrequency(Long pollingFrequency) {
this.pollingFrequency = pollingFrequency;
return this;
}
public PongMessage withPollingFrequency(Long pollingFrequency) {
setPollingFrequency(pollingFrequency);
return this;
}
public String getAgentsConfigurationJson() {
return agentsConfigurationJson;
}
public void setAgentsConfigurationJson(String agentsConfigurationJson) {
this.agentsConfigurationJson = agentsConfigurationJson;
}
public PongMessage withAgentsConfigurationJson(String agentsConfigurationJson) {
setAgentsConfigurationJson(agentsConfigurationJson);
return this;
}
@Override
public String elementName() {
return "pong";
}
@Override
public String jsonElementName() {
return "pong";
}
@Override
protected PongMessage clone() {
PongMessage copy = new PongMessage();
copy.setPropertiesFrom(this);
return copy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy