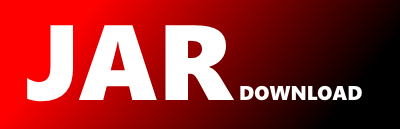
org.qas.qtest.api.services.plan.TestPlanServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.plan;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.Request;
import org.qas.api.internal.util.google.base.Throwables;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.internal.QTestApiWebServiceClient;
import org.qas.qtest.api.services.plan.model.Build;
import org.qas.qtest.api.services.plan.model.CreateBuildRequest;
import org.qas.qtest.api.services.plan.model.CreateReleaseRequest;
import org.qas.qtest.api.services.plan.model.Release;
import org.qas.qtest.api.services.plan.model.transform.BuildJsonUnmarshaller;
import org.qas.qtest.api.services.plan.model.transform.CreateBuildRequestMarshaller;
import org.qas.qtest.api.services.plan.model.transform.CreateReleaseRequestMarshaller;
import org.qas.qtest.api.services.plan.model.transform.ReleaseJsonUnmarshaller;
/**
* TestPlanServiceClient
*
* @author Dzung Nguyen
* @version $Id TestPlanServiceClient 2014-07-18 15:02:30z dungvnguyen $
* @since 1.0
*/
public class TestPlanServiceClient extends QTestApiWebServiceClient
implements TestPlanService {
/**
* Constructs a new client to invoke service method on TestPlanService using
* the default qTest credentials provider and default client configuration options.
*/
public TestPlanServiceClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestPlanService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestPlanService
*/
public TestPlanServiceClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service method on TestPlanService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public TestPlanServiceClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestPlanService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestPlanService
*/
public TestPlanServiceClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = new StaticQTestCredentialsProvider(credentials);
init();
}
/**
* Constructs a new client to invoke service method on TestPlanService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
*/
public TestPlanServiceClient(QTestCredentialsProvider credentialsProvider) {
this(credentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on TestPlanService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to TestPlanService
*/
public TestPlanServiceClient(QTestCredentialsProvider credentialsProvider, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = credentialsProvider;
init();
}
@Override
public Build createBuild(CreateBuildRequest createBuildRequest) throws AuthServiceException {
try {
Request request = new CreateBuildRequestMarshaller().marshall(createBuildRequest);
return invoke(request, BuildJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("An error occurs during creating build.", ex);
}
}
@Override
public Release createRelease(CreateReleaseRequest createReleaseRequest) throws AuthServiceException {
try {
Request request = new CreateReleaseRequestMarshaller().marshall(createReleaseRequest);
return invoke(request, ReleaseJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("An error occurs during creating release.", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy