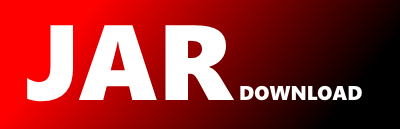
org.qas.qtest.api.services.project.ProjectService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.qtest.api.internal.QTestService;
import org.qas.qtest.api.internal.model.Field;
import org.qas.qtest.api.services.authenticate.AuthenticateServiceClient;
import org.qas.qtest.api.services.plan.model.Release;
import org.qas.qtest.api.services.project.model.*;
import java.util.List;
/**
* ProjectService
*
* @author Dzung Nguyen
* @version $Id ProjectService 2014-03-27 15:42:30z dungvnguyen $
* @since 1.0
*/
public interface ProjectService extends QTestService {
/**
* Lists all projects in user space.
*
* @param request the given request to listing all projects.
* @return all projects in user space.
* @throws AuthServiceException if an error occurs during listing all projects.
*/
List listProject(ListProjectRequest request) throws AuthServiceException;
/**
* Creates project from the given {@link CreateProjectRequest create project request}
*
* @param request the given {@link CreateProjectRequest create project request} to set.
* @return the created project instance.
* @throws AuthServiceException if an error occurs during creating project.
*/
Project createProject(CreateProjectRequest request) throws AuthServiceException;
/**
* Gets the project from the given get project request.
*
* @param request the given {@link GetProjectRequest get project request}.
* @return the project information.
* @throws AuthServiceException if an error occurs during getting project.
*/
Project getProject(GetProjectRequest request) throws AuthServiceException;
//~ Modules =================================================================
/**
* Update modules request.
*
* @param moduleRequest the given update module request.
* @return the current module.
* @throws AuthServiceException if an error occurs during updating module.
*/
Module updateModule(UpdateModuleRequest moduleRequest) throws AuthServiceException;
/**
* Move modules request.
*
* @param moduleRequest the given module request.
* @return the current module.
* @throws AuthServiceException if an error occurs during moving module.
*/
Module moveModule(MoveModuleRequest moduleRequest) throws AuthServiceException;
/**
* Creates module from the given {@link CreateModuleRequest create module request}
* object instance.
*
* @param request the given {@link CreateModuleRequest create module request} to set.
* @return the create module instance.
* @throws AuthServiceException if an error occurs during creating module.
*/
Module createModule(CreateModuleRequest request) throws AuthServiceException;
/**
* Gets module from the given {@link GetModuleRequest get module request} object
* instance.
*
* @param getModuleRequest the given {@link GetModuleRequest get module request}
* @return the {@link Module module} instance.
* @throws AuthServiceException if an error occurs during getting module.
*/
Module getModule(GetModuleRequest getModuleRequest) throws AuthServiceException;
/**
* Lists modules from the given {@link ListModuleRequest get module request} object
* instance.
*
* @param listModuleRequest the given {@link ListModuleRequest list module request}
* @return the list of {@link Module module}s.
* @throws AuthServiceException if an error occurs during listing modules.
*/
List listModule(ListModuleRequest listModuleRequest) throws AuthServiceException;
/**
* List all release of project
* @param listReleaseRequest request to list release
* @return a list of release
* @throws AuthServiceException if an error occurs during listing release
*/
List listRelease(ListReleaseRequest listReleaseRequest) throws AuthServiceException;
/**
* Search modules.
*
* @param searchModuleRequest the given search module request.
* @return the list of modules.
* @throws AuthServiceException if an error occurs during searching modules.
*/
List searchModule(SearchModuleRequest searchModuleRequest) throws AuthServiceException;
/**
* Delete module.
*
* @param moduleRequest the given delete module request.
* @throws AuthServiceException if an error occurs during deleting module.
*/
void deleteModule(DeleteModuleRequest moduleRequest) throws AuthServiceException;
//~ Fields ==================================================================
/**
* Creates custom field from the given {@link CreateCustomFieldRequest} instance.
*
* @param createCustomFieldRequest the given {@link CreateCustomFieldRequest} instance to set.
* @return the {@link Field} instance.
* @throws AuthServiceException if an error occurs during creating custom field to project.
*/
Field createCustomField(CreateCustomFieldRequest createCustomFieldRequest) throws AuthServiceException;
/**
* Update is_active of custom field
* @param updateCustomFieldRequest
* @return
* @throws AuthServiceException
*/
List updateCustomField(UpdateCustomFieldRequest updateCustomFieldRequest) throws AuthServiceException;
/**
* Updates system field from the given {@link UpdateSystemFieldRequest} instance.
*
* @param updateSystemFieldRequest the given {@link UpdateSystemFieldRequest} instance to set.
* @throws AuthServiceException if an error occurs during
*/
Field updateSystemField(UpdateSystemFieldRequest updateSystemFieldRequest) throws AuthServiceException;
/**
* Gets user permission.
*
* @param getUserPermissionsRequest the given {@link GetUserPermissionsRequest} instance.
* @return the {@link UserPermissions} object.
* @throws AuthServiceException if an error occurs during getting user permissions.
*/
UserPermissions getUserPermissions(GetUserPermissionsRequest getUserPermissionsRequest) throws AuthServiceException;
/**
* List all user permissions in all active projects.
*
* @param listUserPermissionsRequest the given {@link ListUserPermissionsRequest} instance.
* @return the list of {@link UserPermissions user permission} objects.
* @throws AuthServiceException if an error occurs during listing all user permissions on active projects.
*/
List listUserPermissions(ListUserPermissionsRequest listUserPermissionsRequest) throws AuthServiceException;
/**
* Gets all fields from the given field request.
*
* @param getFieldsRequest the given {@link GetFieldsRequest GET fields request} to get
* all fields.
* @return the list of fields.
* @throws AuthServiceException if an error occurs during getting fields.
*/
List getFields(GetFieldsRequest getFieldsRequest) throws AuthServiceException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy