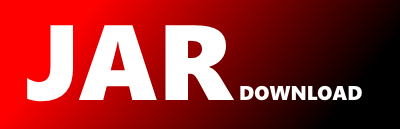
org.qas.qtest.api.services.project.ProjectServiceAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.internal.model.Field;
import org.qas.qtest.api.services.project.model.*;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
/**
* ProjectServiceAsyncClient
*
* @author Dzung Nguyen
* @version $Id ProjectServiceAsyncClient 2014-03-27 22:28:30z dungvnguyen $
* @since 1.0
*/
public class ProjectServiceAsyncClient extends ProjectServiceClient implements ProjectServiceAsync {
/**
* Constructs a new client to invoke service method on ProjectService using
* the default qTest credentials provider and default client configuration options.
*/
public ProjectServiceAsyncClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the default qTest credentials provider and default client configuration options.
*
* @param executorService the executor service for executing asynchronous request.
*/
public ProjectServiceAsyncClient(ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
*/
public ProjectServiceAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService.
* @param executorService the executor service for executing asynchronous request.
*/
public ProjectServiceAsyncClient(ClientConfiguration clientConfiguration, ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public ProjectServiceAsyncClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param executorService the executor service for executing asynchronous request.
*/
public ProjectServiceAsyncClient(QTestCredentials credentials, ExecutorService executorService) {
this(credentials, new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
*/
public ProjectServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
this(credentials, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
* @param executorService the executor service for executing asynchronous request.
*/
public ProjectServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration,
ExecutorService executorService) {
this(new StaticQTestCredentialsProvider(credentials), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on ProjectServiceAsync using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
*/
public ProjectServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration) {
this(credentialsProvider, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on ProjectServiceAsync using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
* @param executorService the executor service for executing asynchronous request.
*/
public ProjectServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(credentialsProvider, clientConfiguration);
this.executorService = executorService;
}
@Override
public Future> listProjectAsync(final ListProjectRequest listProjectRequest) throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
return listProject(listProjectRequest);
}
});
}
@Override
public Future> listProjectAsync(final ListProjectRequest listProjectRequest,
final AsyncHandler> asyncHandler) throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
List result;
try {
result = listProject(listProjectRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listProjectRequest, result);
return result;
}
});
}
@Override
public Future createProjectAsync(final CreateProjectRequest createProjectRequest)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Project call() throws Exception {
return createProject(createProjectRequest);
}
});
}
@Override
public Future createProjectAsync(final CreateProjectRequest createProjectRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Project call() throws Exception {
Project result;
try {
result = createProject(createProjectRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createProjectRequest, result);
return result;
}
});
}
@Override
public Future createModuleAsync(final CreateModuleRequest createModuleRequest)
throws AuthServiceException {
return executorService.submit(new Callable(){
@Override
public Module call() throws Exception {
return createModule(createModuleRequest);
}
});
}
@Override
public Future createModuleAsync(final CreateModuleRequest createModuleRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Module call() throws Exception {
Module result;
try {
result = createModule(createModuleRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createModuleRequest, result);
return result;
}
});
}
@Override
public Future getModuleAsync(final GetModuleRequest getModuleRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Module call() throws Exception {
return getModule(getModuleRequest);
}
});
}
@Override
public Future getModuleAsync(final GetModuleRequest getModuleRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Module call() throws Exception {
Module result;
try {
result = getModule(getModuleRequest);
} catch (Exception e) {
asyncHandler.onError(e);
throw e;
}
asyncHandler.onSuccess(getModuleRequest, result);
return result;
}
});
}
@Override
public Future> listModuleAsync(final ListModuleRequest listModuleRequest)
throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
return listModule(listModuleRequest);
}
});
}
@Override
public Future> listModuleAsync(final ListModuleRequest listModuleRequest,
final AsyncHandler> asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
List result;
try {
result = listModule(listModuleRequest);
} catch (Exception e) {
asyncHandler.onError(e);
throw e;
}
asyncHandler.onSuccess(listModuleRequest, result);
return result;
}
});
}
@Override
public Future createCustomFieldAsync(final CreateCustomFieldRequest createCustomFieldRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Field call() throws Exception {
return createCustomField(createCustomFieldRequest);
}
});
}
@Override
public Future createCustomFieldAsync(final CreateCustomFieldRequest createCustomFieldRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Field call() throws Exception {
final Field result;
try {
result = createCustomField(createCustomFieldRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createCustomFieldRequest, result);
return result;
}
});
}
@Override
public Future updateSystemFieldAsync(final UpdateSystemFieldRequest updateSystemFieldRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Field call() throws Exception {
return updateSystemField(updateSystemFieldRequest);
}
});
}
@Override
public Future updateSystemFieldAsync(final UpdateSystemFieldRequest updateSystemFieldRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public Field call() throws Exception {
final Field result;
try {
result = updateSystemField(updateSystemFieldRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateSystemFieldRequest, result);
return result;
}
});
}
@Override
public Future> getFieldsAsync(final GetFieldsRequest getFieldsRequest) throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
return getFields(getFieldsRequest);
}
});
}
@Override
public Future> getFieldsAsync(final GetFieldsRequest getFieldsRequest,
final AsyncHandler> asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
final List result;
try {
result = getFields(getFieldsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getFieldsRequest, result);
return result;
}
});
}
@Override
public Future getUserPermissionsAsync(final GetUserPermissionsRequest getUserPermissionsRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public UserPermissions call() throws Exception {
return getUserPermissions(getUserPermissionsRequest);
}
});
}
@Override
public Future getUserPermissionsAsync(final GetUserPermissionsRequest getUserPermissionsRequest,
final AsyncHandler asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public UserPermissions call() throws Exception {
final UserPermissions result;
try {
result = getUserPermissions(getUserPermissionsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getUserPermissionsRequest, result);
return result;
}
});
}
@Override
public Future> listUserPermissionsAsync(final ListUserPermissionsRequest listUserPermissionsRequest)
throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
return listUserPermissions(listUserPermissionsRequest);
}
});
}
@Override
public Future> listUserPermissionsAsync(final ListUserPermissionsRequest listUserPermissionsRequest,
final AsyncHandler> asyncHandler)
throws AuthServiceException {
return executorService.submit(new Callable>() {
@Override
public List call() throws Exception {
final List result;
try {
result = listUserPermissions(listUserPermissionsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listUserPermissionsRequest, result);
return result;
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy