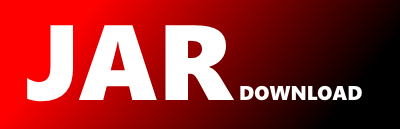
org.qas.qtest.api.services.project.ProjectServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project;
import org.qas.api.AuthClientException;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.Request;
import org.qas.api.internal.util.google.base.Throwables;
import org.qas.api.transform.VoidJsonUnmarshaller;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.internal.QTestApiWebServiceClient;
import org.qas.qtest.api.internal.model.Field;
import org.qas.qtest.api.internal.model.transform.FieldJsonUnmarshaller;
import org.qas.qtest.api.internal.model.transform.ListFieldJsonUnmarshaller;
import org.qas.qtest.api.services.plan.model.Release;
import org.qas.qtest.api.services.project.model.*;
import org.qas.qtest.api.services.project.model.transform.*;
import java.util.List;
/**
* ProjectServiceClient
*
* @author Dzung Nguyen
* @version $Id ProjectServiceClient 2014-03-27 18:51:30z dungvnguyen $
* @since 1.0
*/
public class ProjectServiceClient extends QTestApiWebServiceClient
implements ProjectService {
/**
* Constructs a new client to invoke service method on ProjectService using
* the default qTest credentials provider and default client configuration options.
*/
public ProjectServiceClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
*/
public ProjectServiceClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public ProjectServiceClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
*/
public ProjectServiceClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = new StaticQTestCredentialsProvider(credentials);
init();
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
*/
public ProjectServiceClient(QTestCredentialsProvider credentialsProvider) {
this(credentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service method on ProjectService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to ProjectService
*/
public ProjectServiceClient(QTestCredentialsProvider credentialsProvider, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.credentialsProvider = credentialsProvider;
init();
}
@Override
public List listProject(ListProjectRequest listProjectRequest) throws AuthServiceException {
try {
Request request = new ListProjectRequestMarshaller().marshall(listProjectRequest);
return invoke(request, ListProjectJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list project request", ex);
}
}
@Override
public Project createProject(CreateProjectRequest createProjectRequest) throws AuthServiceException {
try {
Request request = new CreateProjectRequestMarshaller().marshall(createProjectRequest);
return invoke(request, ProjectJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute create project request", ex);
}
}
@Override
public Project getProject(GetProjectRequest getProjectRequest) throws AuthServiceException {
try {
Request request = new GetProjectRequestMarshaller().marshall(getProjectRequest);
return invoke(request, new ProjectJsonUnmarshaller().getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute get project request", ex);
}
}
@Override
public Module updateModule(UpdateModuleRequest moduleRequest) throws AuthServiceException {
try {
Request request = new UpdateModuleRequestMarshaller().marshall(moduleRequest);
return invoke(request, ModuleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute update module request", ex);
}
}
@Override
public Module moveModule(MoveModuleRequest moduleRequest) throws AuthServiceException {
try {
Request request = new MoveModuleRequestMarshaller().marshall(moduleRequest);
return invoke(request, ModuleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute move module request", ex);
}
}
@Override
public Module createModule(CreateModuleRequest createModuleRequest) throws AuthServiceException {
try {
Request request = new CreateModuleRequestMarshaller().marshall(createModuleRequest);
return invoke(request, ModuleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute create module request", ex);
}
}
@Override
public Module getModule(GetModuleRequest getModuleRequest) throws AuthServiceException {
try {
Request request = new GetModuleRequestMarshaller().marshall(getModuleRequest);
return invoke(request, ModuleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute get module request", ex);
}
}
@Override
public List listModule(ListModuleRequest listModuleRequest) throws AuthServiceException {
try {
Request request = new ListModuleRequestMarshaller().marshall(listModuleRequest);
return invoke(request, ListModuleJsonUnmarshaller.getInstance());
} catch (Exception e) {
Throwables.propagateIfInstanceOf(e, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list module request", e);
}
}
@Override
public List listRelease(ListReleaseRequest listReleaseRequest) throws AuthServiceException {
try {
Request request = new ListReleaseRequestMarshaller().marshall(listReleaseRequest);
return invoke(request, ListReleaseJsonUnmarshaller.getInstance());
} catch (Exception e) {
Throwables.propagateIfInstanceOf(e, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during execute list release request", e);
}
}
@Override
public List searchModule(SearchModuleRequest searchModuleRequest) throws AuthServiceException {
try {
Request request = new SearchModuleRequestMarshaller().marshall(searchModuleRequest);
return invoke(request, ListModuleJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during executing search modules request.", ex);
}
}
@Override
public void deleteModule(DeleteModuleRequest moduleRequest) throws AuthServiceException {
try {
Request request = new DeleteModuleRequestMarshaller().marshall(moduleRequest);
invoke(request, new VoidJsonUnmarshaller());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during executing delete module request.", ex);
}
}
@Override
public Field createCustomField(CreateCustomFieldRequest createCustomFieldRequest) throws AuthServiceException {
try {
Request request = new CreateCustomFieldRequestMarshaller().marshall(createCustomFieldRequest);
return invoke(request, FieldJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during creating custom field of specific project", ex);
}
}
@Override
public List updateCustomField(UpdateCustomFieldRequest updateCustomFieldRequest) throws AuthServiceException {
try {
Request request = new UpdateCustomFieldRequestMarshaller().marshall(updateCustomFieldRequest);
return invoke(request, ListFieldJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during update custom field of specific project", ex);
}
}
@Override
public Field updateSystemField(UpdateSystemFieldRequest updateSystemFieldRequest) throws AuthServiceException {
try {
Request request = new UpdateSystemFieldRequestMarshaller().marshall(updateSystemFieldRequest);
return invoke(request, FieldJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during updating system field of specific project", ex);
}
}
@Override
public List getFields(GetFieldsRequest getFieldsRequest) throws AuthServiceException {
try {
Request request = new GetFieldsRequestMarshaller().marshall(getFieldsRequest);
return invoke(request, ListFieldJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during getting all fields.", ex);
}
}
@Override
public UserPermissions getUserPermissions(GetUserPermissionsRequest getUserPermissionsRequest) throws AuthServiceException {
try {
Request request = new GetUserPermissionsRequestMarshaller().marshall(getUserPermissionsRequest);
return invoke(request, UserPermissionsJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during getting user permissions.", ex);
}
}
@Override
public List listUserPermissions(ListUserPermissionsRequest listUserPermissionsRequest)
throws AuthServiceException {
try {
Request request = new ListUserPermissionsRequestMarshaller().marshall(listUserPermissionsRequest);
return invoke(request, ListUserPermissionsJsonUnmarshaller.getInstance());
} catch (Exception ex) {
Throwables.propagateIfInstanceOf(ex, AuthClientException.class);
throw new AuthClientException("Unknown error occurs during listing user permissions.", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy