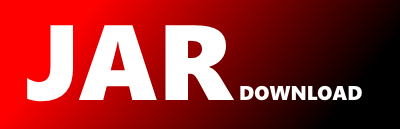
org.qas.qtest.api.services.project.model.DefectTrackingSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.api.JsonMapper;
import org.qas.api.internal.PropertyContainer;
/**
* DefectTrackingSystem
*
* @author Dzung Nguyen
* @version $Id DefectTrackingSystem 2014-03-27 15:45:30z dungvnguyen $
* @since 1.0
*/
public final class DefectTrackingSystem extends PropertyContainer {
@JsonProperty("id")
private Long id;
@JsonProperty("url")
private String url;
@JsonProperty("active")
private Boolean active;
@JsonProperty("connection_name")
private String connectionName;
@JsonProperty("system_name")
private String systemName;
/**
* Creates {@link org.qas.qtest.api.services.project.model.DefectTrackingSystem} object.
*/
public DefectTrackingSystem() {
setActive(Boolean.FALSE);
}
/**
* @return the defect tracking system identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the defect tracking system identifier.
*
* @param id the given defect tracking system identifier.
* @return current instance.
*/
public DefectTrackingSystem setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the defect tracking system identifier and return itself.
*
* @param id the given tracking system identifier.
* @return the current {@link org.qas.qtest.api.services.project.model.DefectTrackingSystem} object.
*/
public DefectTrackingSystem withId(Long id) {
setId(id);
return this;
}
/**
* @return the defect tracking system URL.
*/
public String getUrl() {
return url;
}
/**
* Sets defect tracking system URL.
*
* @param url the given defect tracking URL.
* @return current instance.
*/
public DefectTrackingSystem setUrl(String url) {
this.url = url;
return this;
}
/**
* Sets the defect tracking system URL.
*
* @param url the given defect tracking URL.
* @return current {@link org.qas.qtest.api.services.project.model.DefectTrackingSystem} object.
*/
public DefectTrackingSystem withUrl(String url) {
setUrl(url);
return this;
}
/**
* @return {@code true} if the this defect tracking system is active, otherwise {@code false}
*/
public Boolean isActive() {
return active;
}
/**
* Sets the active status to defect tracking system.
*
* @param active the given active status flag.
* @return current instance.
*/
public DefectTrackingSystem setActive(Boolean active) {
this.active = (active != null ? active : Boolean.FALSE);
return this;
}
/**
* Sets the active status to defect tracking system.
*
* @param active the given active status flag.
* @return current {@link DefectTrackingSystem} object.
*/
@JsonIgnore
public DefectTrackingSystem withActive(Boolean active) {
setActive(active);
return this;
}
/**
* @return the connection name.
*/
public String getConnectionName() {
return connectionName;
}
/**
* Sets the connection name.
*
* @param connectionName the given connection name.
* @return current instance.
*/
public DefectTrackingSystem setConnectionName(String connectionName) {
this.connectionName = connectionName;
return this;
}
/**
* Sets the connection name and return itself.
*
* @param connectionName the given connection name.
* @return current {@link DefectTrackingSystem} object.
*/
public DefectTrackingSystem withConnectionName(String connectionName) {
setConnectionName(connectionName);
return this;
}
/**
* @return the system name.
*/
public String getSystemName() {
return systemName;
}
/**
* Sets the system name.
*
* @param systemName the given system name to set.
* @return current instance.
*/
public DefectTrackingSystem setSystemName(String systemName) {
this.systemName = systemName;
return this;
}
/**
* Sets the system name and return the itself.
*
* @param systemName the given system name to set.
* @return current {@link DefectTrackingSystem} object.
*/
public DefectTrackingSystem withSystemName(String systemName) {
setSystemName(systemName);
return this;
}
@Override
@JsonIgnore
public DefectTrackingSystem clone() {
DefectTrackingSystem that = new DefectTrackingSystem();
that.setPropertiesFrom(this);
return that;
}
@Override
@JsonIgnore
public String elementName() {
return "defect-tracking-system";
}
@Override
@JsonIgnore
public String jsonElementName() {
return "defect_tracking_system";
}
@Override
public String toString() {
return JsonMapper.toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy